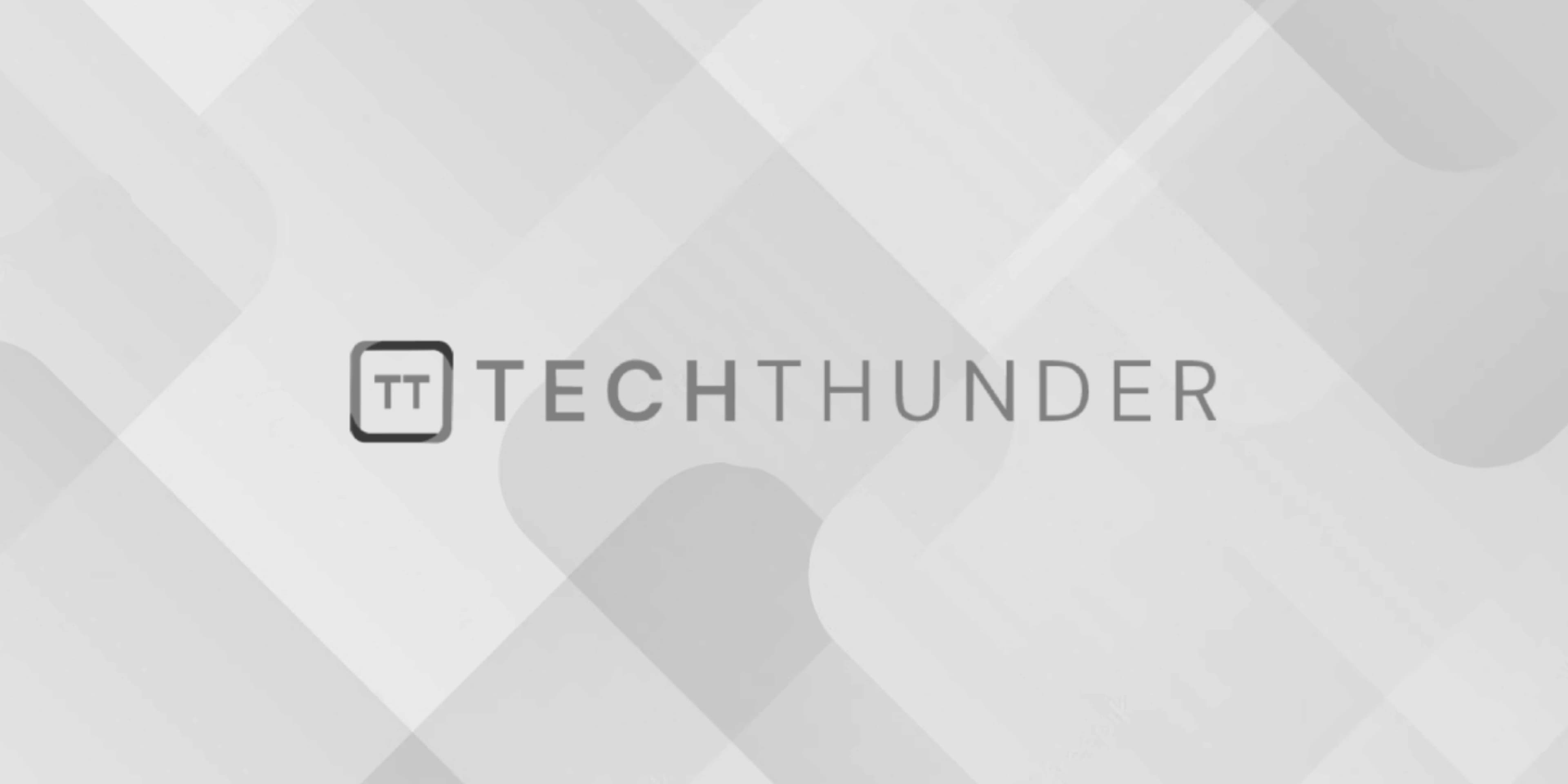
Data Segments
In computer programming, a data segment is a region of a program’s memory space that is used for storing global and static variables. These variables have a lifetime that extends for the duration of the program’s execution and are typically used for storing data that should persist across function calls and throughout the program’s execution. Data segments are an essential part of a program’s memory organization.
Data segments are usually divided into several sections, each serving a specific purpose:
- Initialized Data Segment (.data): This section stores global and static variables that are explicitly initialized with a value. When the program starts, these variables are set to their specified initial values. For example:
int globalVar = 42; // Initialized data segment
- Uninitialized Data Segment (.bss): This section stores global and static variables that are not explicitly initialized. These variables are typically initialized to zero by the system when the program starts. For example:
static int uninitializedVar; // Uninitialized data segment (set to 0)
- Constants Segment (.rodata): This section stores read-only data, such as string literals and other constants, which cannot be modified during program execution. For example:
const char* message = "Hello, World!"; // Constants segment (read-only)
- Thread-Local Storage (TLS): In multithreaded programs, there may be segments dedicated to thread-local storage, where each thread has its own separate area for data.
- Custom Data Sections: Depending on the compiler and platform, you can define custom data sections for specialized data storage requirements.
It’s important to note that the specific names and characteristics of these segments can vary depending on the operating system and compiler being used. However, the general concept of data segments remains consistent across different systems.
Here’s a simplified memory layout to illustrate the organization of data segments:
---------------------
| Code Segment | (contains program instructions)
---------------------
| Data Segment | (contains global and static variables)
| .data | (initialized data)
| .bss | (uninitialized data)
| .rodata | (constants)
| TLS | (thread-local storage, if applicable)
---------------------
| Stack Segment | (contains function call stack)
---------------------
| Heap Segment | (dynamic memory allocation, e.g., malloc)
---------------------
Understanding data segments is important for managing memory in your programs, as it helps you control the scope and lifetime of your variables and data.