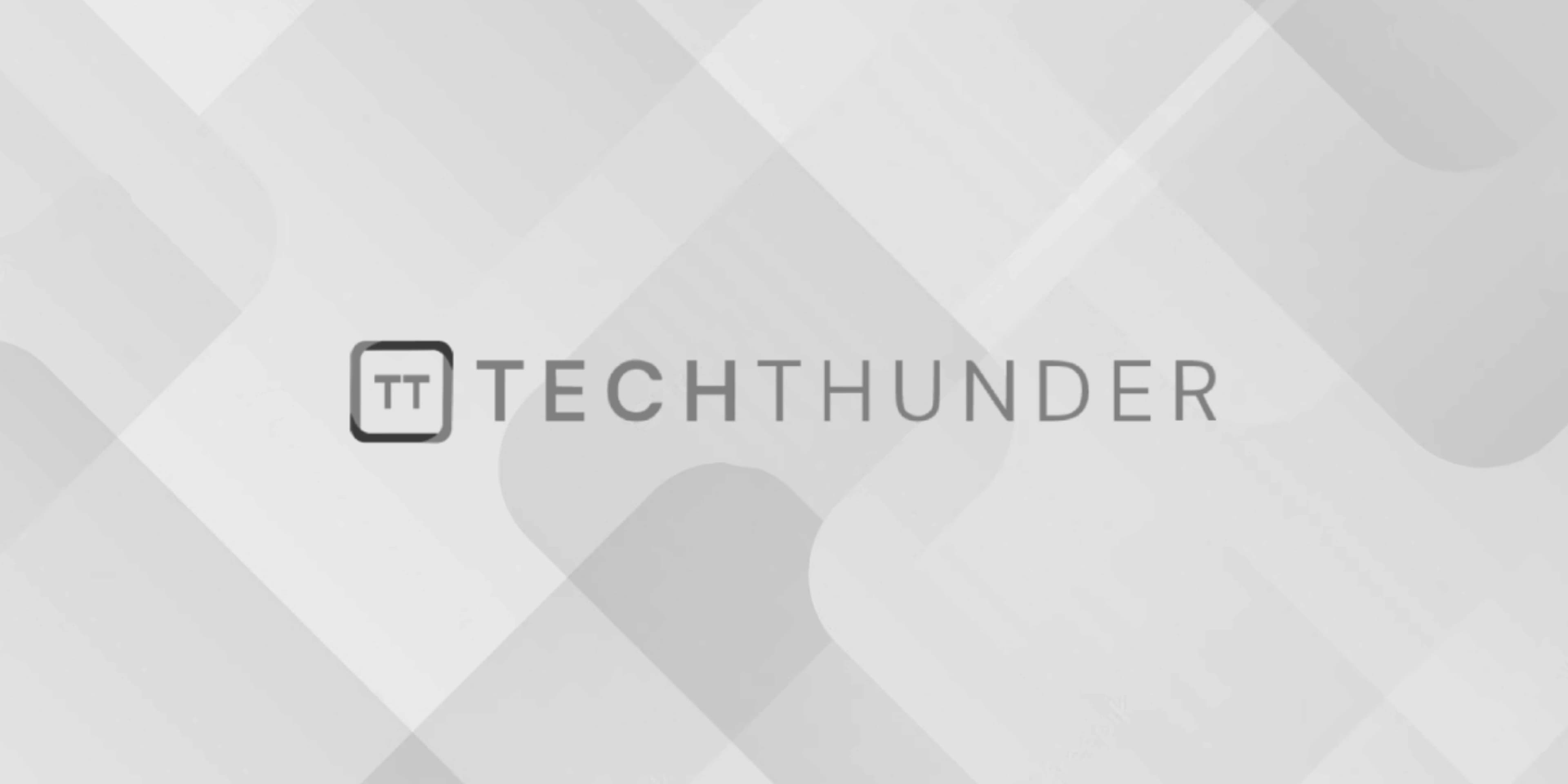
Ceil Function in C
The ceil()
function is part of the math library (<math.h>
) and is used to round a floating-point number up to the nearest integer greater than or equal to that number. It returns a double value.
Here’s the syntax of the ceil()
function:
double ceil(double x);
x
: The floating-point number you want to round up to the nearest integer.
The ceil()
function returns the smallest integral value (as a double) that is greater than or equal to x
. The returned value is of type double
, so you may need to cast it to an integer type if you want an integer result.
Here’s an example of how to use the ceil()
function:
#include <stdio.h>
#include <math.h>
int main() {
double num = 5.4;
double result = ceil(num);
printf("Original number: %.1lf\n", num);
printf("Ceiled number: %.1lf\n", result);
return 0;
}
In this example:
- We include the
<math.h>
header to use theceil()
function. - We have a floating-point number
num
with the value5.4
. - We use the
ceil(num)
function to roundnum
up to the nearest integer greater than or equal to it. - The result is printed, showing that
5.4
is rounded up to6.0
.
Remember that the ceil()
function always returns a double-precision floating-point number, so if you need an integer result, you can cast it to an integer type, such as int
. For example:
int integerResult = (int)ceil(num);
This will give you the ceiled value as an integer.