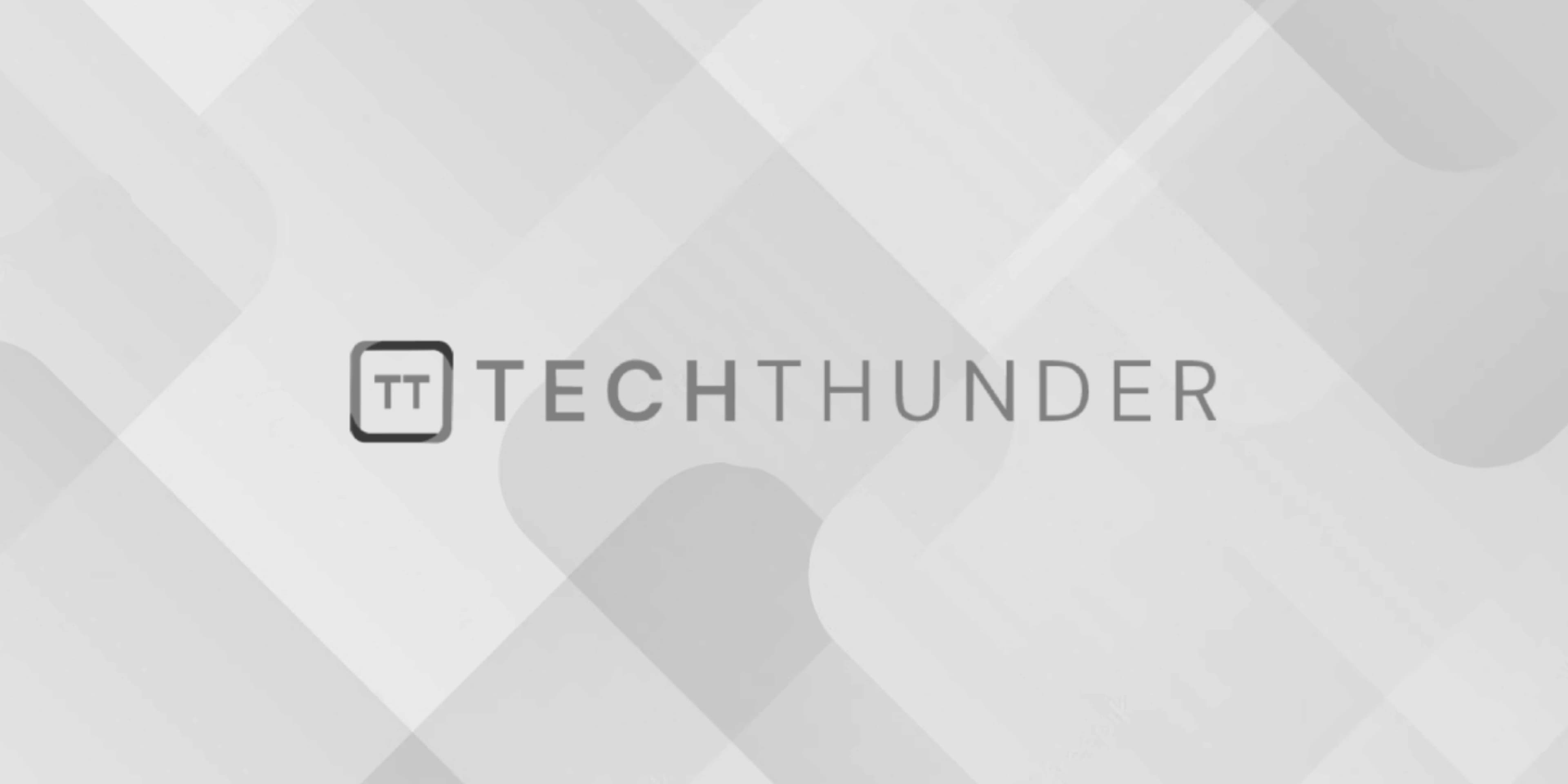
277 views
qsort() in C
The qsort()
is a standard C library function used for sorting an array or a collection of elements. It is part of the <stdlib.h>
header and provides a way to perform a generic sorting operation on an array or any collection of elements.
Here is the general syntax of the qsort()
function:
C
void qsort(void *base, size_t nmemb, size_t size, int (*compar)(const void *, const void *));
Parameters:
base
: Pointer to the beginning of the array to be sorted.nmemb
: Number of elements in the array.size
: Size in bytes of each element in the array.compar
: Pointer to a comparison function that defines the sorting order. This function takes twoconst void *
arguments and returns an integer less than, equal to, or greater than zero if the first argument should be considered less than, equal to, or greater than the second argument, respectively.
Here’s an example of how to use qsort()
to sort an array of integers in ascending order:
C
#include <stdio.h>
#include <stdlib.h>
// Comparison function for qsort to sort integers in ascending order
int compare(const void *a, const void *b) {
return (*(int *)a - *(int *)b);
}
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
// Using qsort to sort the array
qsort(arr, n, sizeof(int), compare);
printf("Sorted array in ascending order:\n");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
In this example:
- We define a comparison function
compare
that compares two integers and returns the result of the subtraction (a - b
). - We use
qsort()
to sort thearr
array of integers in ascending order by providing the array pointer, the number of elements, the size of each element, and the comparison function as arguments. - After sorting, we print the sorted array.
You can modify the compare
function to sort elements in different orders (e.g., descending order) or sort arrays of structures or other data types by changing the comparison logic accordingly.