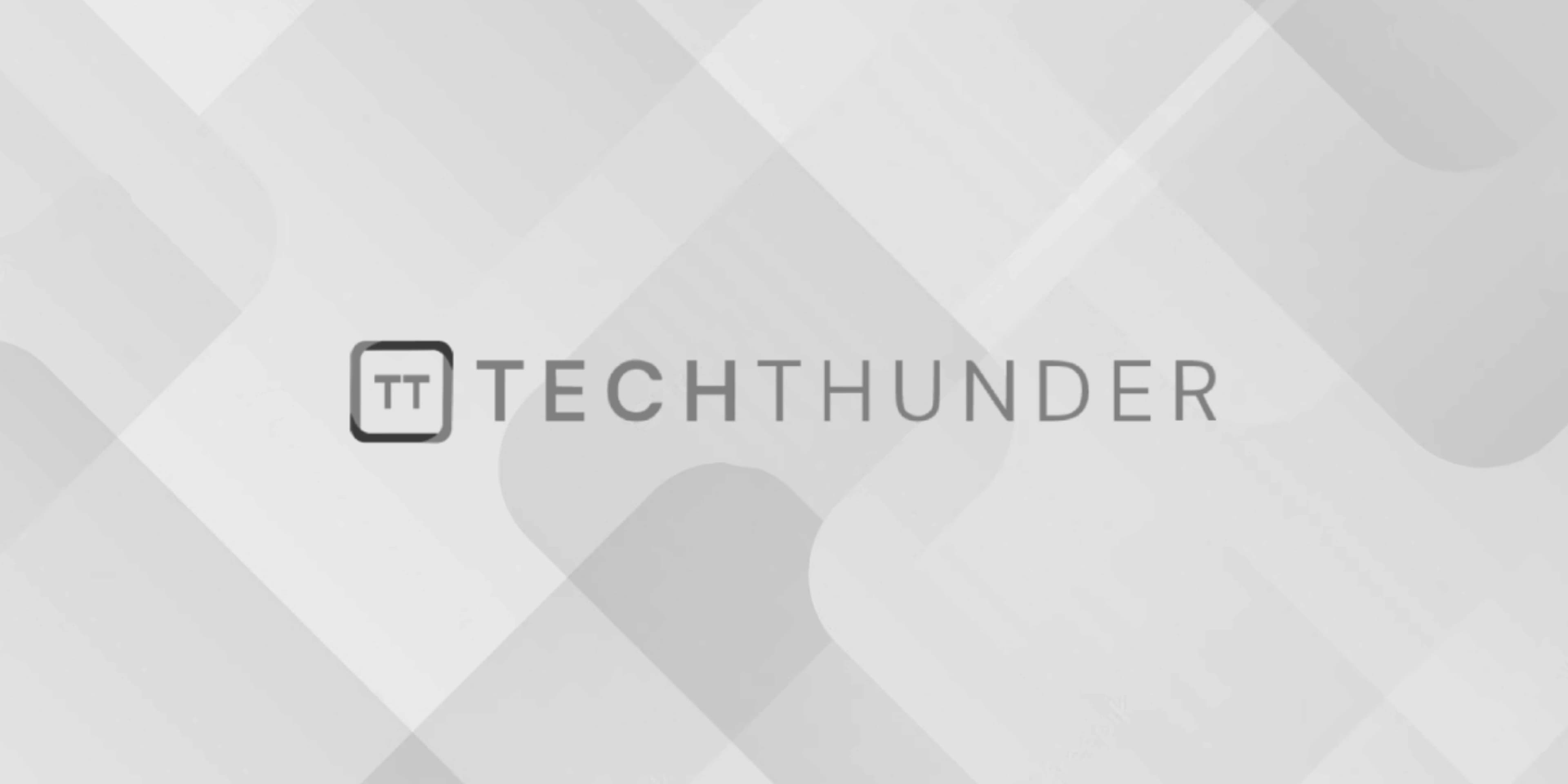
73 views
Leap year program in C
You can create a program to determine whether a given year is a leap year or not. Leap years have an extra day, February 29th, and follow a specific rule:
- A year is a leap year if it is divisible by 4.
- However, if a year is divisible by 100, it is not a leap year unless it is also divisible by 400.
Here’s a C program to check if a year is a leap year or not:
C
#include <stdio.h>
int main() {
int year;
printf("Enter a year: ");
scanf("%d", &year);
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
printf("%d is a leap year.\n", year);
} else {
printf("%d is not a leap year.\n", year);
}
return 0;
}
In this program:
- We prompt the user to enter a year.
- We use the condition
(year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)
to check if the year is divisible by 4 but not divisible by 100 (or divisible by 400). If this condition is true, the year is a leap year. - If the condition is met, we print that the year is a leap year; otherwise, we print that it is not a leap year.
Compile and run the program, and it will determine whether the entered year is a leap year or not based on the leap year rule.