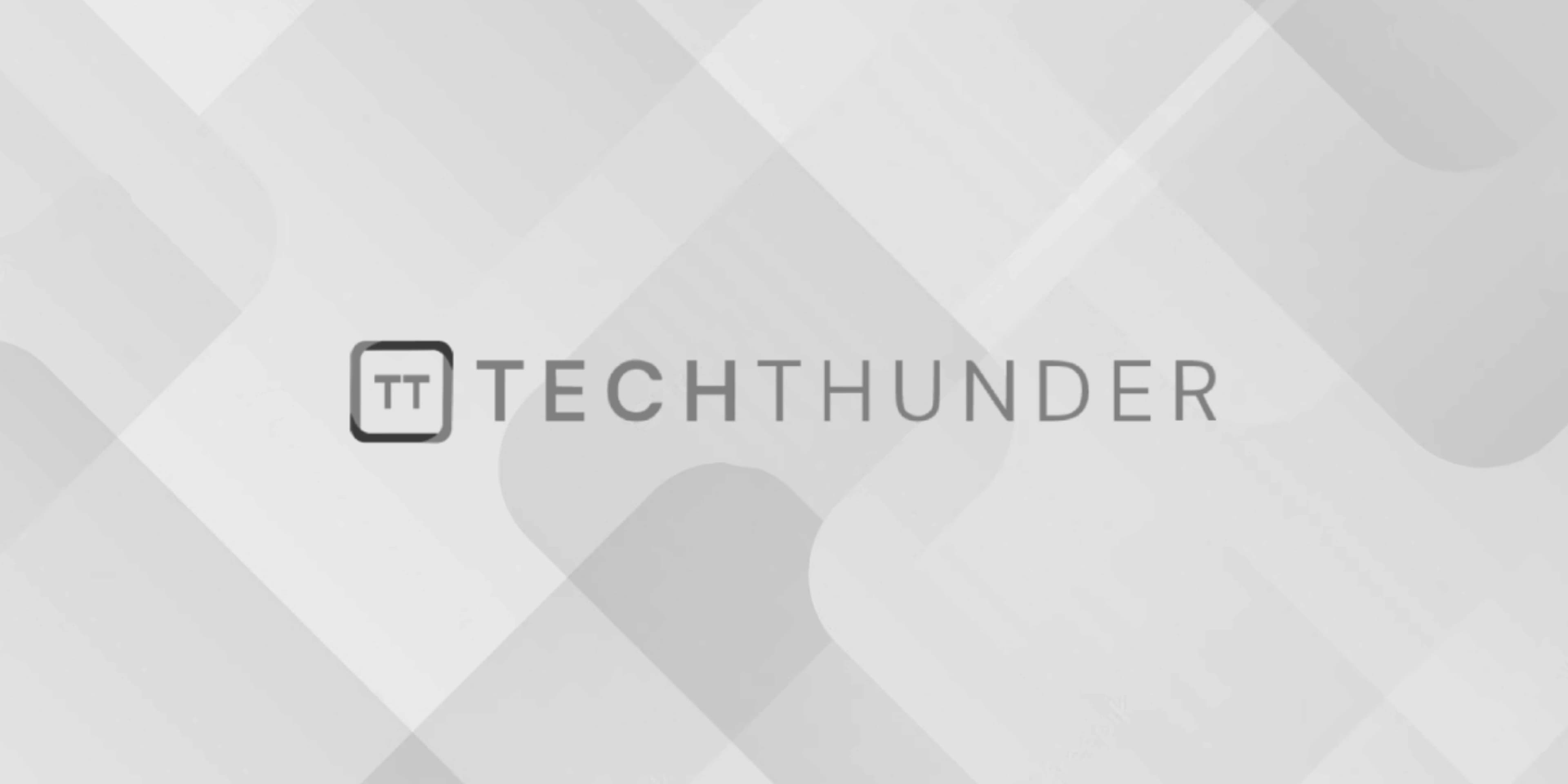
274 views
Break Vs Continue in C
The break
and continue
are control flow statements used within loops to alter the flow of the program. They serve different purposes:
break
Statement:
- The
break
statement is used to exit the current loop prematurely when a certain condition is met. When encountered inside a loop, it immediately terminates the loop and transfers control to the first statement after the loop. - It is often used to exit a loop when a specific condition is satisfied or to implement an early exit strategy.
- Example of
break
:for (int i = 1; i <= 10; i++) { if (i == 5) { break; // Exit the loop when i is 5 } printf("%d ", i); }
In this example, the loop will terminate wheni
becomes 5, and the output will be1 2 3 4
.
continue
Statement:
- The
continue
statement is used to skip the rest of the code within the current iteration of a loop and proceed to the next iteration of the loop. It does not exit the loop entirely; instead, it skips the remaining statements within the current iteration. - It is often used when you want to skip specific iterations of a loop based on a condition, without terminating the entire loop.
- Example of
continue
:for (int i = 1; i <= 10; i++) { if (i % 2 == 0) { continue; // Skip even numbers } printf("%d ", i); }
In this example, thecontinue
statement is used to skip even numbers, so the output will be1 3 5 7 9
.
In summary:
break
is used to exit the current loop prematurely.continue
is used to skip the remaining statements within the current iteration and proceed to the next iteration of the loop.
Both break
and continue
are valuable control flow tools that allow you to control the behavior of loops based on specific conditions or criteria.