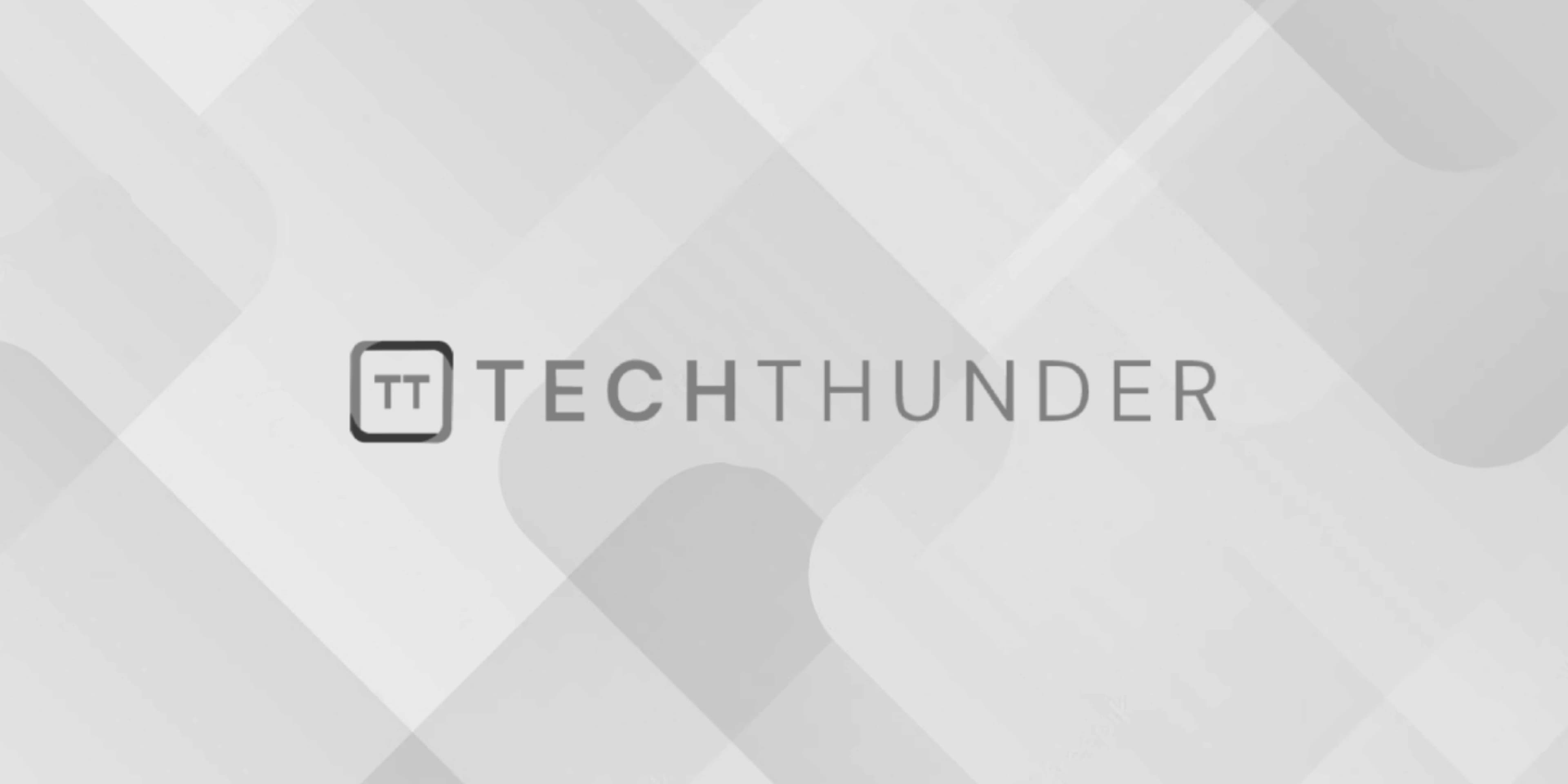
isprint() function in C
The isprint()
function is a part of the C Standard Library and is used to check if a character is a printable character or not. Printable characters are those characters that can be displayed or printed, and they generally include alphanumeric characters, punctuation marks, and some special characters. The isprint()
function is defined in the ctype.h
header.
Here’s the prototype of the isprint()
function:
#include <ctype.h>
int isprint(int c);
c
: An integer that represents a character (or the result ofgetc()
orfgetc()
).
The isprint()
function returns a non-zero value if the character c
is a printable character, and it returns 0
(false) if the character is not printable.
Here’s an example of how to use the isprint()
function:
#include <stdio.h>
#include <ctype.h>
int main() {
int ch;
printf("Enter a character: ");
ch = getchar();
if (isprint(ch)) {
printf("'%c' is a printable character.\n", ch);
} else {
printf("'%c' is not a printable character.\n", ch);
}
return 0;
}
In this example:
- We use
getchar()
to read a character from the user. - We then use
isprint()
to check if the entered character is printable or not. - Depending on the result, we print a message indicating whether the character is printable or not.
This function is often used when dealing with character validation or filtering in C programs, especially when you need to ensure that the input contains only printable characters.