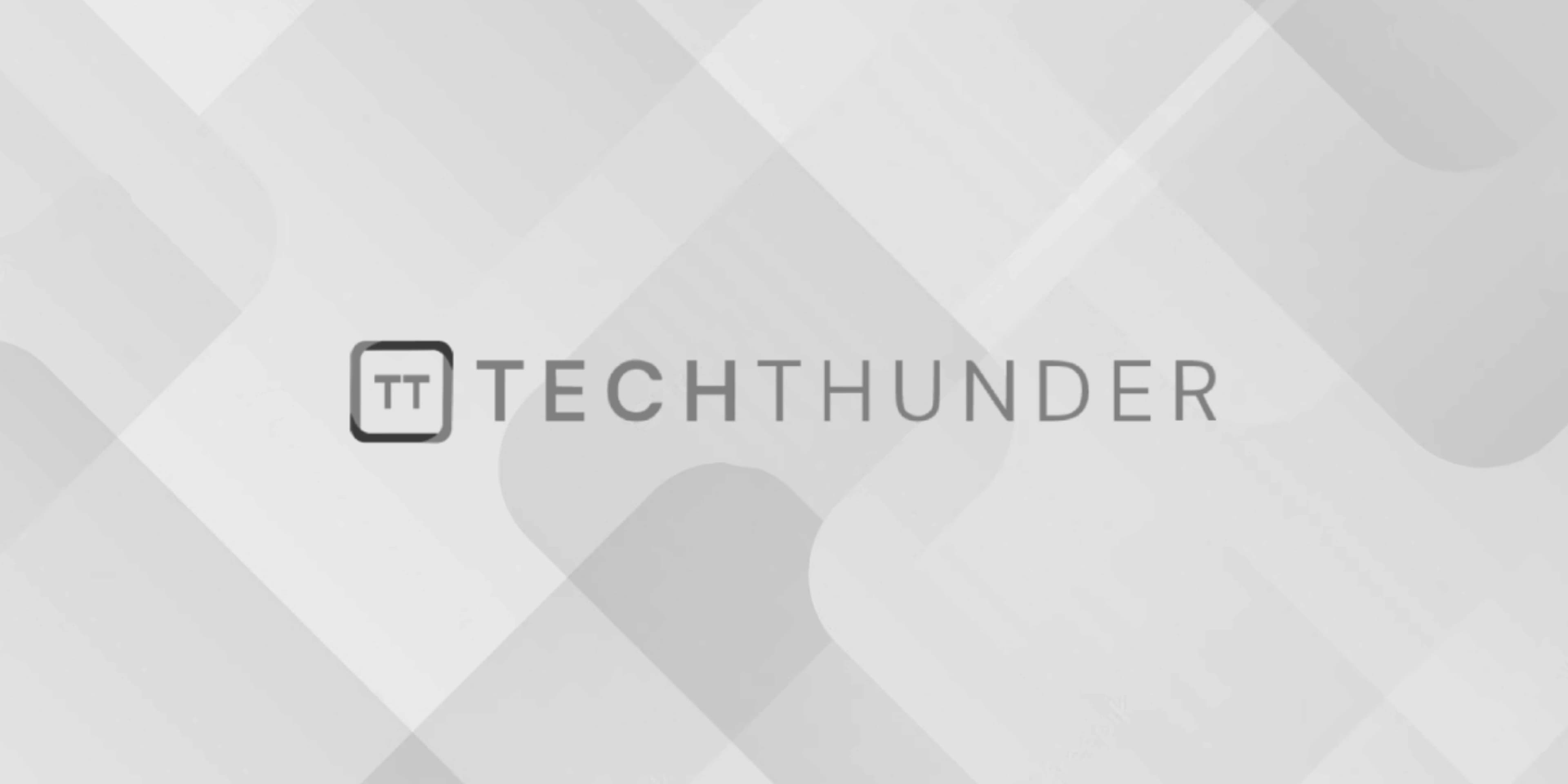
130 views
C Math Functions
C provides a set of math functions as part of the standard library, which are declared in the <math.h>
header. These functions allow you to perform various mathematical operations, including basic arithmetic, trigonometry, logarithms, exponentiation, and more. Here are some commonly used math functions in C:
- Basic Arithmetic Functions:
int abs(int x)
: Returns the absolute value of an integerx
.double fabs(double x)
: Returns the absolute value of a floating-point numberx
.double sqrt(double x)
: Returns the square root of a numberx
.double pow(double x, double y)
: Returnsx
raised to the power ofy
.
- Trigonometric Functions:
double sin(double x)
: Returns the sine of an anglex
(in radians).double cos(double x)
: Returns the cosine of an anglex
(in radians).double tan(double x)
: Returns the tangent of an anglex
(in radians).double atan(double x)
: Returns the arctangent of a numberx
(in radians).double atan2(double y, double x)
: Returns the arctangent of the ratioy/x
(in radians).
- Logarithmic Functions:
double log(double x)
: Returns the natural logarithm of a numberx
.double log10(double x)
: Returns the base-10 logarithm of a numberx
.double log2(double x)
: Returns the base-2 logarithm of a numberx
.
- Exponential Functions:
double exp(double x)
: Returns the exponential value ofx
.double exp2(double x)
: Returns 2 raised to the power ofx
.
- Rounding Functions:
double floor(double x)
: Returns the largest integer less than or equal tox
.double ceil(double x)
: Returns the smallest integer greater than or equal tox
.double round(double x)
: Roundsx
to the nearest integer value.
- Other Functions:
double fmod(double x, double y)
: Returns the remainder ofx
divided byy
.double hypot(double x, double y)
: Returns the hypotenuse length of a right triangle with sides of lengthx
andy
.double copysign(double x, double y)
: Returnsx
with the sign ofy
.
These are just some of the commonly used math functions available in C. To use these functions, include the <math.h>
header and link your program with the math library (e.g., by adding -lm
during compilation on Unix-like systems).
Here’s an example of using some of these functions:
C
#include <stdio.h>
#include <math.h>
int main() {
double x = 2.0;
double y = 3.0;
printf("Square root of %f is %f\n", x, sqrt(x));
printf("2 raised to the power of %f is %f\n", y, exp2(y));
printf("The sine of %f radians is %f\n", x, sin(x));
return 0;
}
This example demonstrates the use of sqrt()
, exp2()
, and sin()
functions to perform basic mathematical operations.