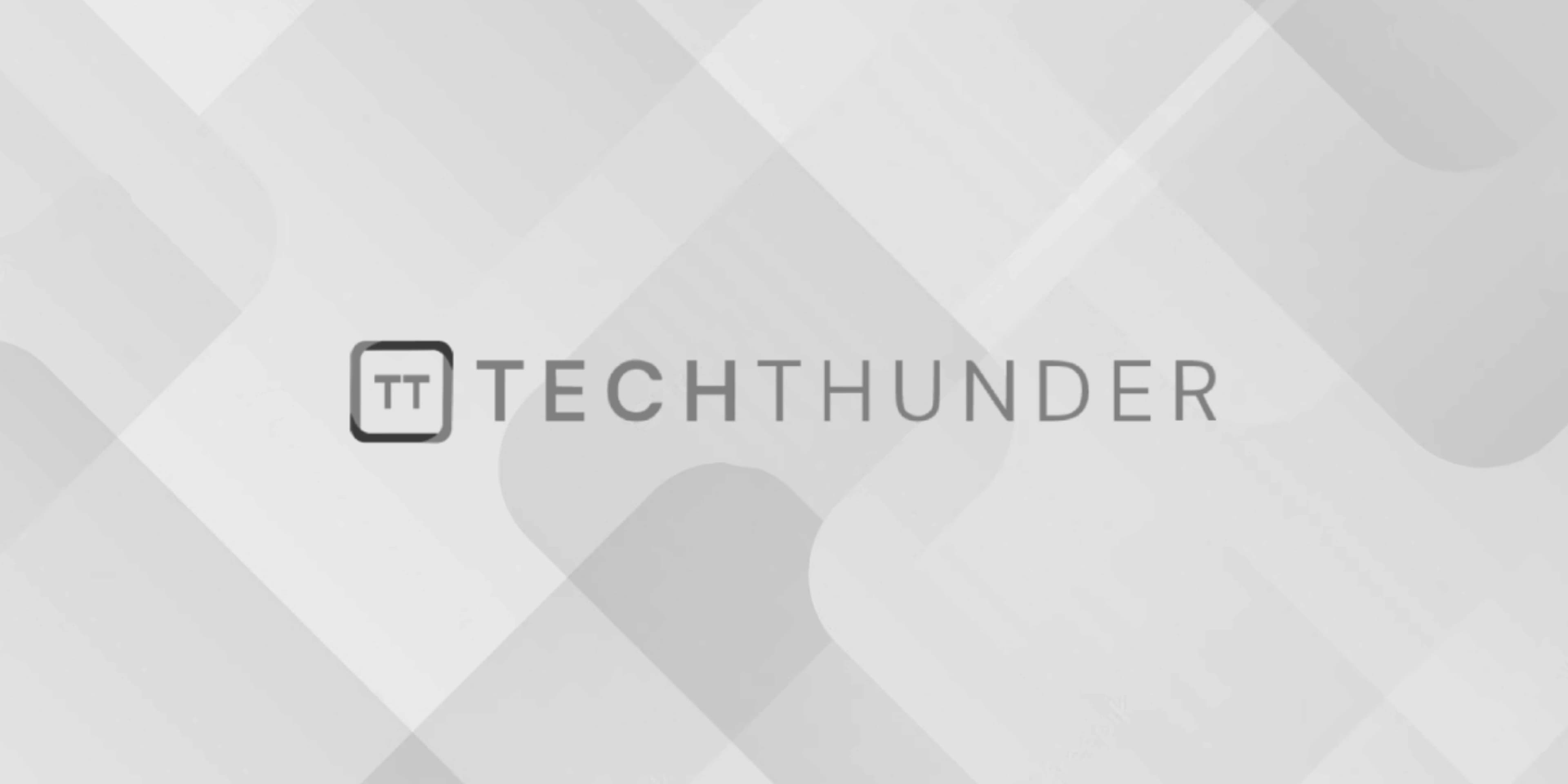
Understanding the extern Keyword in C
In C programming, the extern
keyword is used to declare a variable or a function in a source file that is intended to be used in another source file. The extern
keyword informs the compiler that the specified variable or function is defined in another source file, and the actual definition will be provided at the linking phase.
Here are two common uses of the extern
keyword:
- Declaring Global Variables:
// File1.c
int globalVariable; // Definition of the variable
// File2.c
extern int globalVariable; // Declaration of the variable from File1.c
In this example, File1.c
contains the definition of the global variable globalVariable
. In File2.c
, the extern
keyword is used to declare the existence of globalVariable
without allocating memory for it. When the program is linked, the linker will resolve the reference to globalVariable
and connect it to the actual definition in File1.c
.
- Declaring Functions:
// File1.c
void myFunction() {
// Function definition
}
// File2.c
extern void myFunction(); // Declaration of the function from File1.c
Here, File1.c
contains the definition of the function myFunction
. In File2.c
, the extern
keyword is used to declare the function without providing its actual implementation. The linking phase will resolve this declaration to the definition in File1.c
.
It’s important to note that using extern
is not mandatory for functions because function declarations are implicitly assumed to be extern
if not specified otherwise. However, explicitly using extern
for functions can improve code clarity.
In summary, extern
is used to declare variables or functions in one source file that are defined in another source file, allowing the linker to connect references to actual implementations during the linking phase.