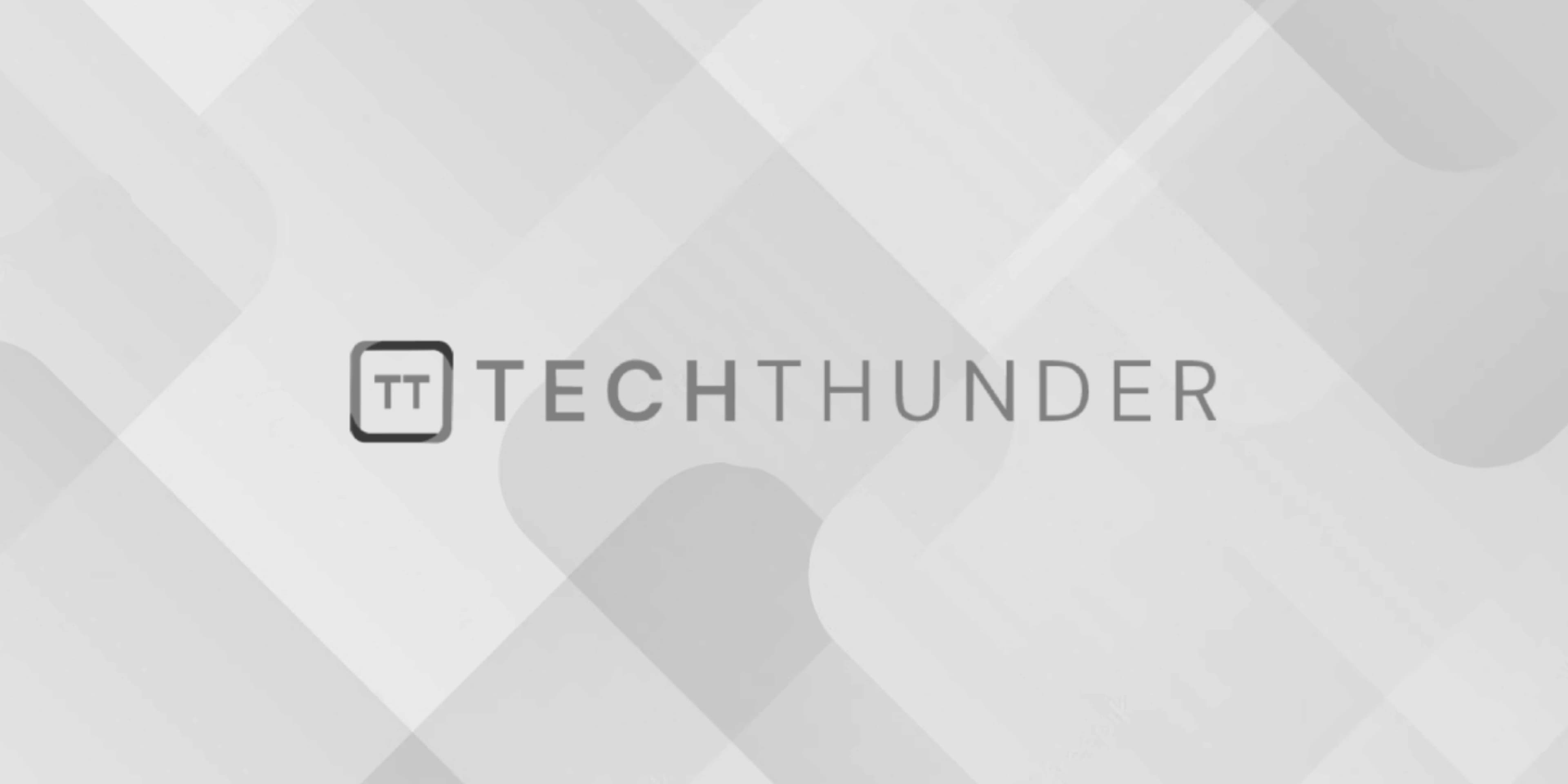
105 views
C Program to find the roots of quadratic equation
To find the roots of a quadratic equation of the form ax^2 + bx + c = 0
, you can use the quadratic formula, which is:
C
x = (-b ± √(b^2 - 4ac)) / (2a)
Here’s a C program that calculates the roots of a quadratic equation when the coefficients a
, b
, and c
are provided by the user:
C
#include <stdio.h>
#include <math.h>
int main() {
double a, b, c;
double discriminant, root1, root2;
// Input coefficients from the user
printf("Enter coefficients (a, b, and c): ");
scanf("%lf %lf %lf", &a, &b, &c);
// Calculate the discriminant
discriminant = b * b - 4 * a * c;
// Check the value of the discriminant to determine the roots
if (discriminant > 0) {
// Two real and distinct roots
root1 = (-b + sqrt(discriminant)) / (2 * a);
root2 = (-b - sqrt(discriminant)) / (2 * a);
printf("Roots are real and distinct:\n");
printf("Root 1 = %.2lf\n", root1);
printf("Root 2 = %.2lf\n", root2);
} else if (discriminant == 0) {
// One real root (root1 and root2 are the same)
root1 = -b / (2 * a);
printf("Root is real and identical:\n");
printf("Root 1 = Root 2 = %.2lf\n", root1);
} else {
// Complex roots (real and imaginary parts)
double realPart = -b / (2 * a);
double imaginaryPart = sqrt(-discriminant) / (2 * a);
printf("Roots are complex:\n");
printf("Root 1 = %.2lf + %.2lfi\n", realPart, imaginaryPart);
printf("Root 2 = %.2lf - %.2lfi\n", realPart, imaginaryPart);
}
return 0;
}
In this program:
- The user is prompted to input the coefficients
a
,b
, andc
. - The discriminant is calculated using the formula
discriminant = b^2 - 4ac
. - The value of the discriminant is used to determine the nature of the roots:
- If
discriminant > 0
, there are two real and distinct roots. - If
discriminant == 0
, there is one real root (root1 and root2 are the same). - If
discriminant < 0
, there are complex roots with real and imaginary parts. - The roots are then calculated and displayed accordingly.
Compile and run the program, and it will calculate and display the roots of the quadratic equation based on the coefficients entered by the user.