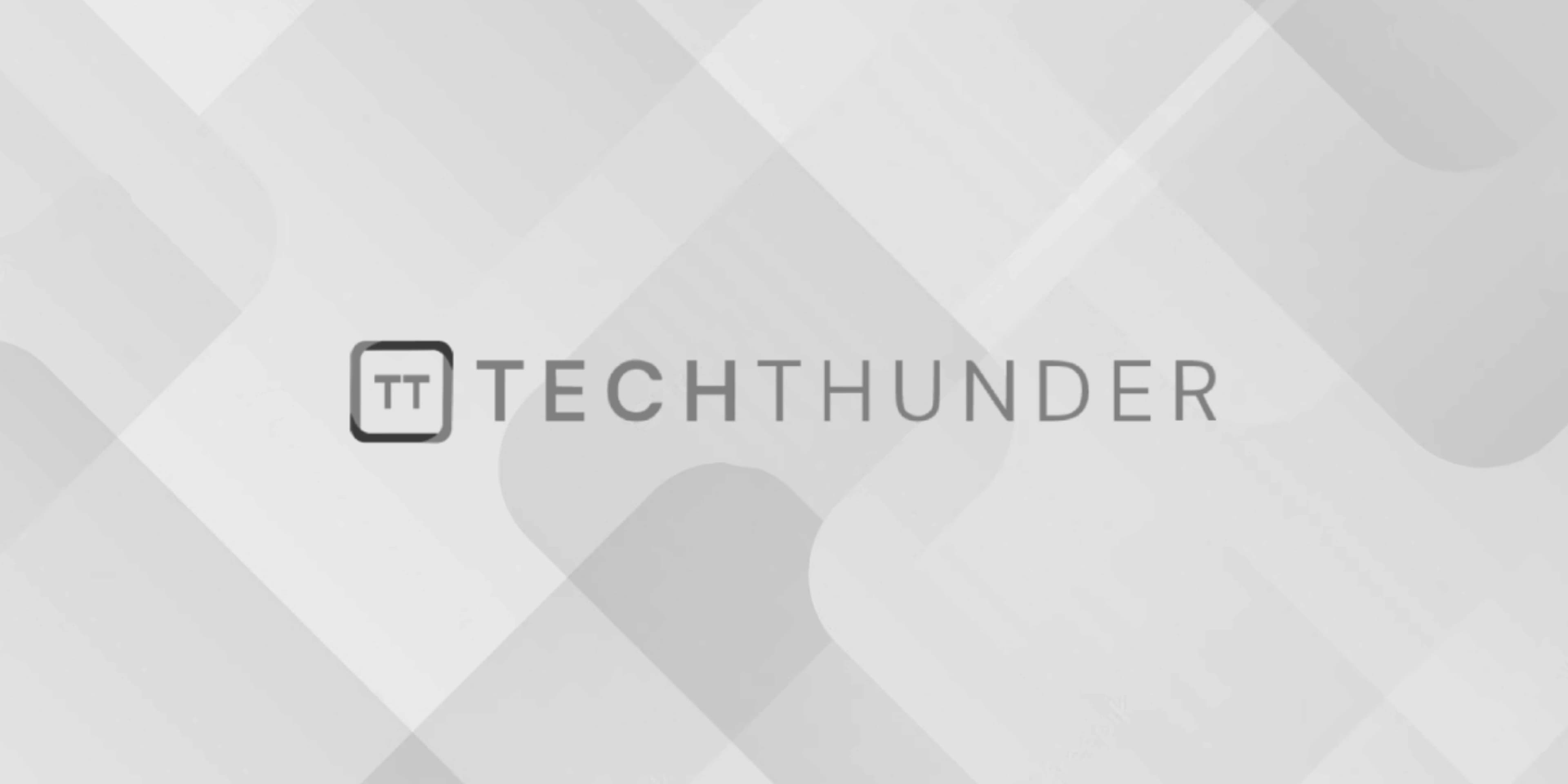
Range of Int in C
The range of the int
data type is implementation-dependent, which means it can vary from one C compiler and system to another. However, the C standard (as of C99 and C11) specifies minimum requirements for the range of int
.
The C standard specifies that an int
must have a minimum range of at least -32,767 to +32,767 (i.e., -2^15+1 to 2^15-1) for a two’s complement system. This corresponds to 16 bits, where one bit is used to represent the sign (positive or negative) of the integer.
Here’s the general formula for calculating the range of int
on a two’s complement system:
- Minimum
int
value: -2^(n-1) wheren
is the number of bits used forint
. - Maximum
int
value: 2^(n-1) – 1
For example, on most modern systems, including those using the x86 architecture, the int
data type typically has a range of -2,147,483,647 to +2,147,483,647 for a 32-bit int
, and -9,223,372,036,854,775,807 to +9,223,372,036,854,775,807 for a 64-bit int
.
To determine the exact range of int
on your specific system, you can use the limits.h
header and check the values of INT_MIN
and INT_MAX
. For example:
#include <stdio.h>
#include <limits.h>
int main() {
printf("Minimum int value: %d\n", INT_MIN);
printf("Maximum int value: %d\n", INT_MAX);
return 0;
}
This program will print the minimum and maximum values of the int
data type for your specific system.