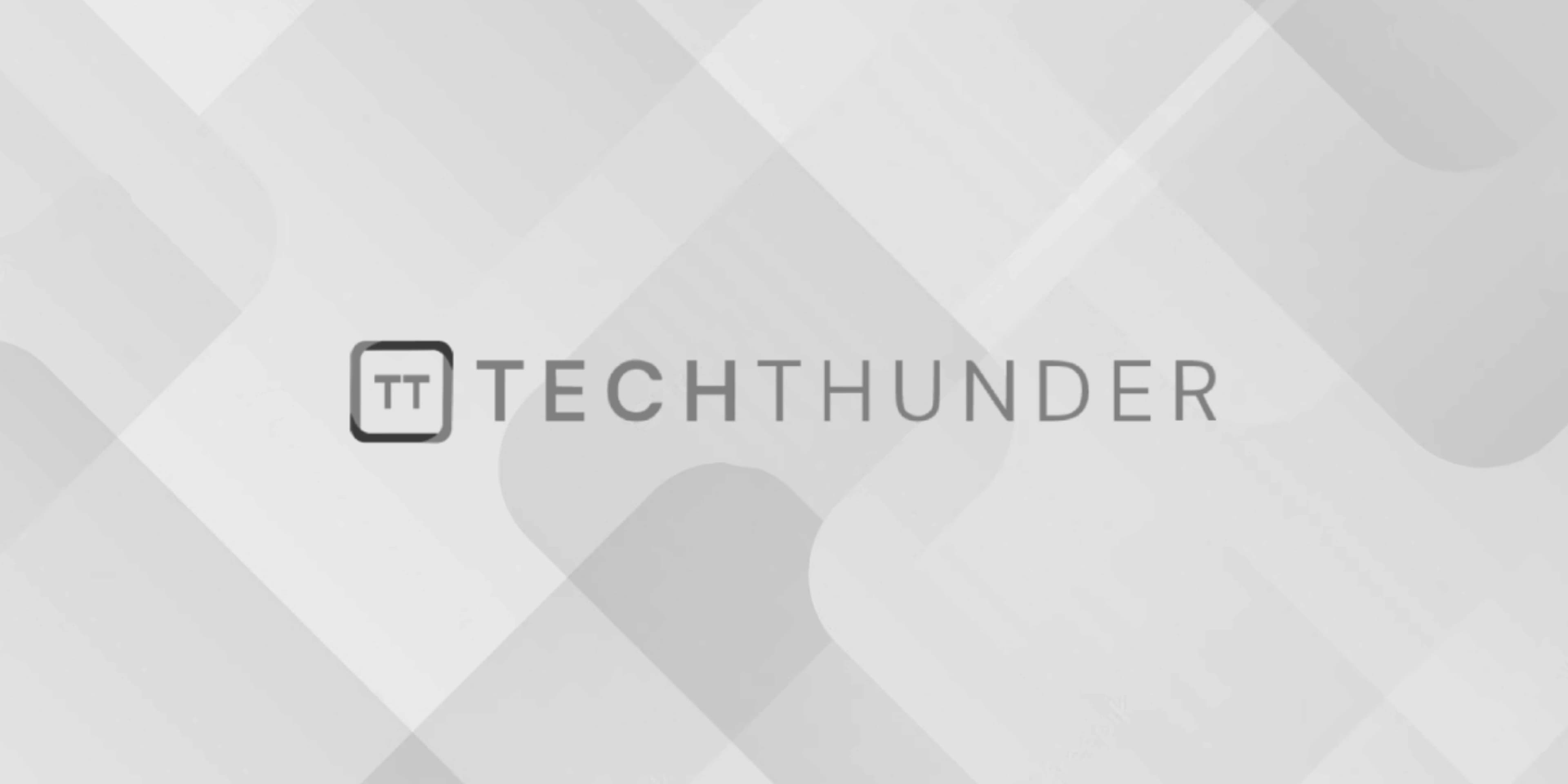
flowchart in C
Creating a flowchart in C is not something that can be directly done within the C programming language itself. Flowcharts are graphical representations of algorithms or processes and are typically created using flowcharting software or drawing tools, not by writing code in C.
Here’s a general process for creating a flowchart for a C program:
- Plan Your Algorithm: Before creating a flowchart, you need to have a clear understanding of the algorithm or logic you want to represent. Write down the steps and decisions that your program will make.
- Choose a Flowcharting Tool: There are various flowcharting tools available, both online and offline, that allow you to create flowcharts easily. Some popular choices include Microsoft Visio, Lucidchart, Draw.io, and even simple drawing applications like Microsoft Paint or a pencil and paper.
- Create Symbols: Flowcharts use specific symbols to represent different elements like processes, decisions, input/output, etc. Familiarize yourself with these symbols and use them appropriately in your flowchart.
- Design the Flowchart: Use the chosen flowcharting tool to design your flowchart based on the algorithm you’ve planned. Start with a start symbol, add shapes for processes and decisions, connect them with arrows to show the flow of control, and end with an end symbol.
- Label Symbols: Add labels to each symbol to describe the action or decision being taken at that step.
- Test and Review: Carefully review your flowchart to ensure it accurately represents your program’s logic. Step through the flowchart manually to see if it matches your algorithm.
- Share or Implement: Once you have a finalized flowchart, you can share it with others as documentation for your program. It can also be a helpful guide when implementing the actual C code for your program.
Here’s a simple example of what a flowchart might look like for a C program that calculates the factorial of a number:
Start
|
V
Input N
|
V
N = 0?
|
| No
|-----------------|
| |
| Yes |
| |
V |
Output 1 |
| |
|-----------------|
|
V
Result = 1
|
V
For i = 1 to N
|
V
Result = Result * i
|
V
Next i
|
V
Output Result
|
V
End
This flowchart visually represents the steps involved in calculating the factorial of a number N in a C program.
Remember that while flowcharts are valuable for designing and understanding algorithms, you’ll still need to write the actual C code to implement the logic described in the flowchart.