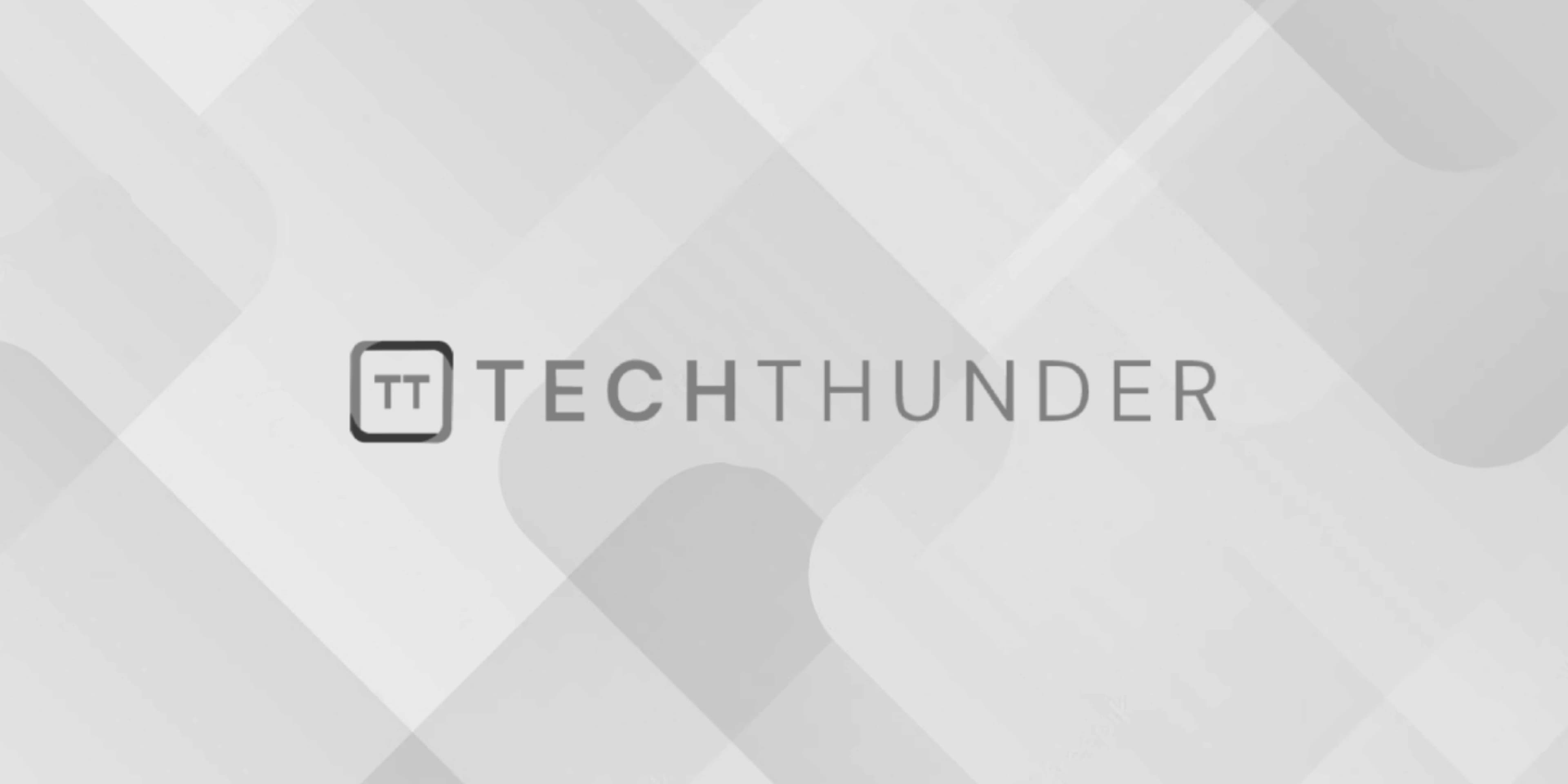
233 views
Hexadecimal to Binary in C
You can convert a hexadecimal number to binary using bitwise operations and some basic programming logic. Here’s a C program that demonstrates how to do this conversion:
C
#include <stdio.h>
#include <string.h>
// Function to convert a hexadecimal digit to its binary equivalent
char* hexToBinary(char hexDigit) {
switch (hexDigit) {
case '0': return "0000";
case '1': return "0001";
case '2': return "0010";
case '3': return "0011";
case '4': return "0100";
case '5': return "0101";
case '6': return "0110";
case '7': return "0111";
case '8': return "1000";
case '9': return "1001";
case 'A': case 'a': return "1010";
case 'B': case 'b': return "1011";
case 'C': case 'c': return "1100";
case 'D': case 'd': return "1101";
case 'E': case 'e': return "1110";
case 'F': case 'f': return "1111";
default:
return NULL;
}
}
int main() {
char hex[17]; // Assuming 16 characters max (plus the null terminator)
printf("Enter a hexadecimal number: ");
scanf("%16s", hex);
int len = strlen(hex);
printf("Binary equivalent: ");
for (int i = 0; i < len; i++) {
char* binary = hexToBinary(hex[i]);
if (binary != NULL) {
printf("%s", binary);
} else {
printf("Invalid hexadecimal digit: %c\n", hex[i]);
return 1; // Exit with an error code
}
}
printf("\n");
return 0;
}
This program defines a function hexToBinary
that converts a hexadecimal digit to its binary representation using a switch statement. The main
function takes a hexadecimal input from the user, converts each digit to binary, and prints the binary equivalent. If an invalid hexadecimal digit is entered, it will display an error message and return with a non-zero exit code.
Compile and run the program, and you can input a hexadecimal number to see its binary equivalent.