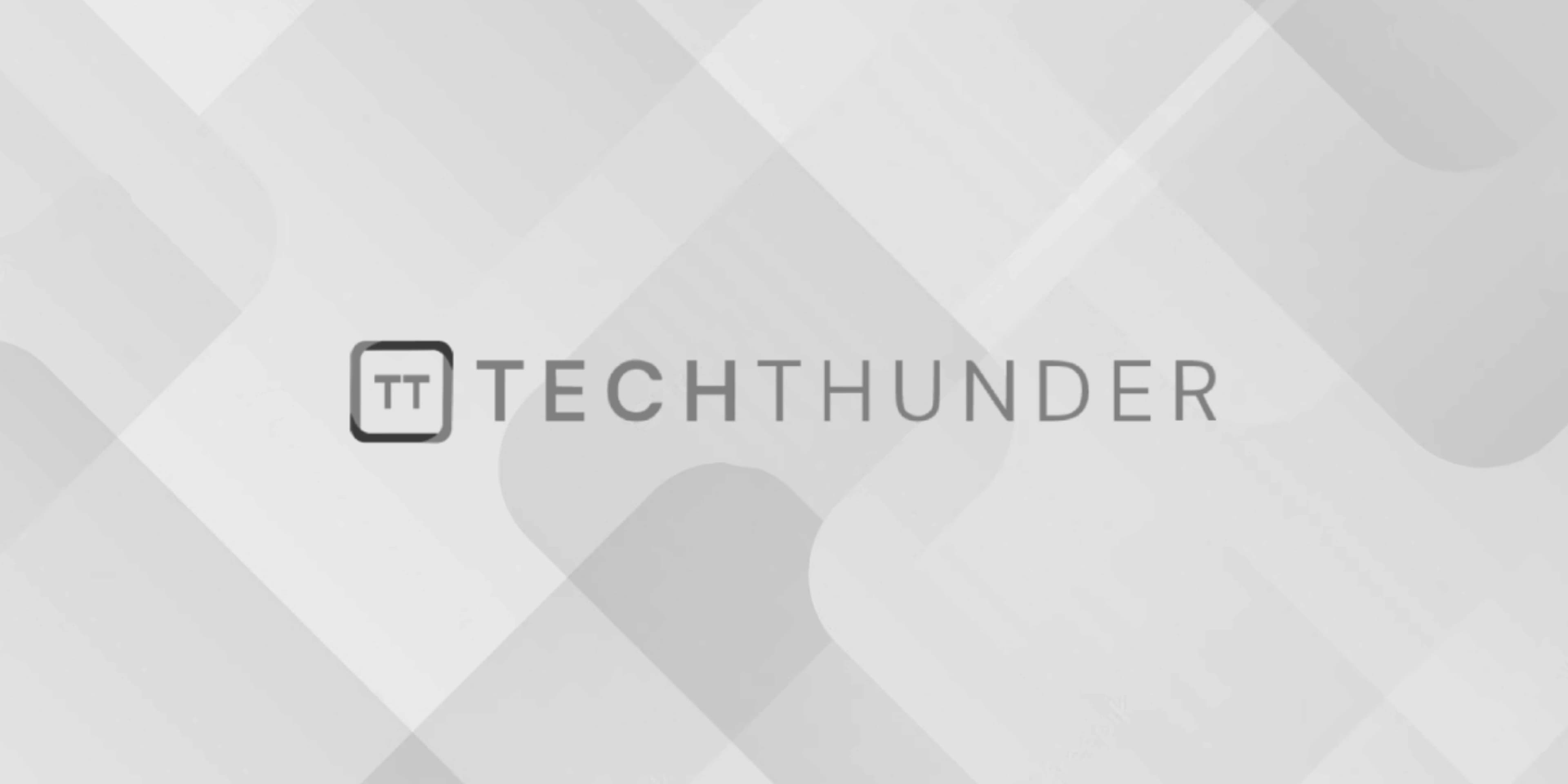
222 views
Floyds Triangle in C
Floyd’s Triangle is a right-angled triangular array of natural numbers, used in computer science and mathematics for various purposes. It is named after American computer scientist Robert W. Floyd. Floyd’s Triangle is constructed in the following way:
Plaintext
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
Each row of the triangle contains consecutive natural numbers starting from 1, and each row has one more number than the previous row. To print Floyd’s Triangle in C, you can use nested loops to generate and display the numbers in the desired pattern. Here’s an example program to print Floyd’s Triangle:
C
#include <stdio.h>
int main() {
int n, num = 1;
printf("Enter the number of rows for Floyd's Triangle: ");
scanf("%d", &n);
printf("Floyd's Triangle:\n");
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
printf("%2d ", num);
num++;
}
printf("\n");
}
return 0;
}
In this program:
- We first ask the user to enter the number of rows they want for Floyd’s Triangle.
- We use two nested loops to print the numbers in the triangle pattern. The outer loop (
i
) controls the number of rows, and the inner loop (j
) prints the numbers in each row. - The variable
num
keeps track of the current number to be printed. We initialize it to 1 and increment it for each number printed. - We use the
%2d
format specifier inprintf
to ensure that each number is printed with at least two characters of width, making the triangle appear properly aligned.
Compile and run the program, and it will print Floyd’s Triangle with the number of rows specified by the user.