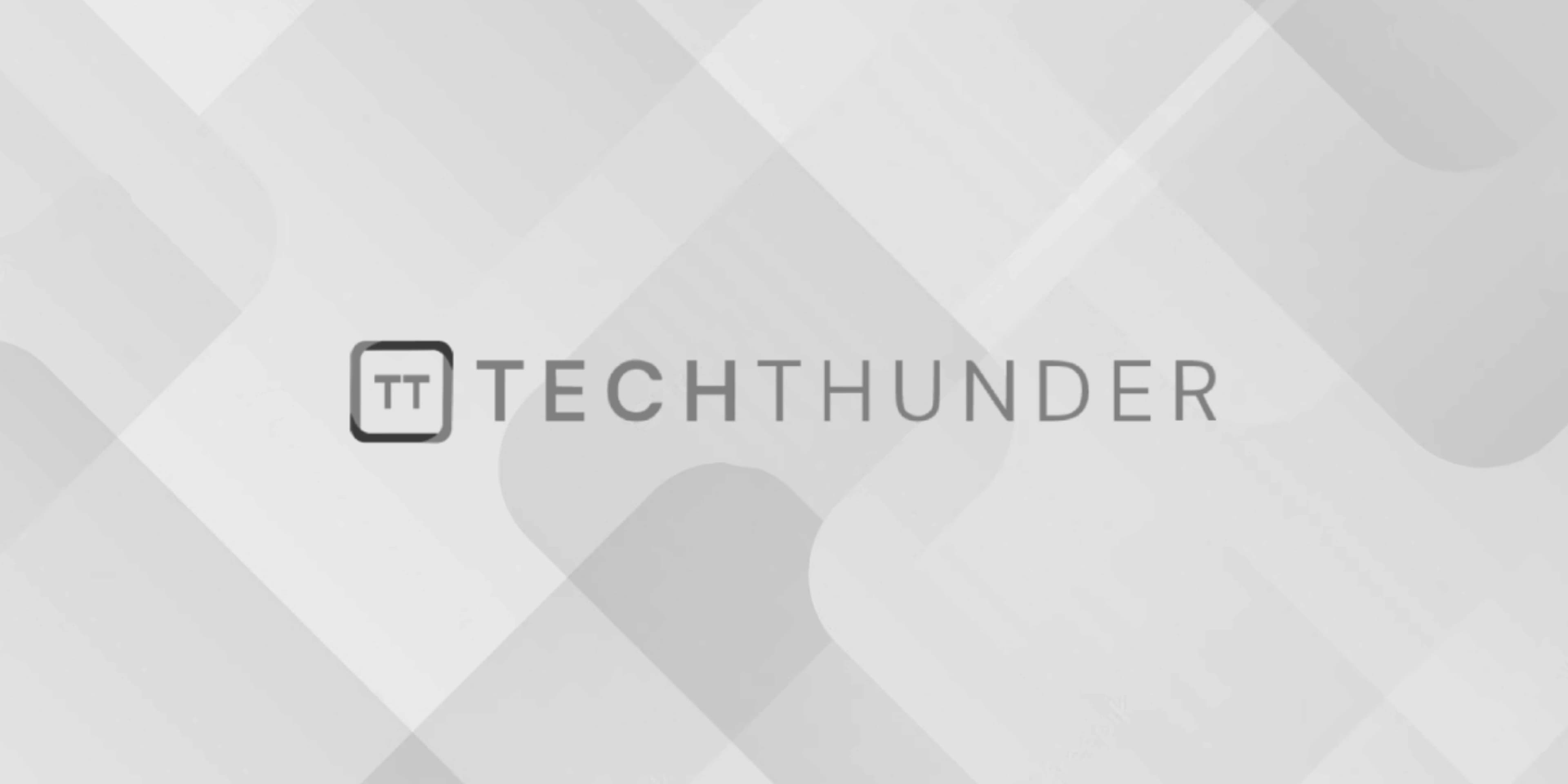
161 views
C #ifndef
The #ifndef
directive is used for conditional compilation. It checks whether a specific macro is not defined, and if the macro is not defined, it includes a block of code for compilation. If the macro is defined, the code within the #ifndef
block is excluded from compilation.
Here’s the basic syntax of the #ifndef
directive:
C
#ifndef macro_name
// Code to compile if macro_name is not defined
#endif
macro_name
is the name of the macro that you want to check for absence (i.e., not being defined).
Here’s an example of how the #ifndef
directive is used:
C
#include <stdio.h>
// Uncomment the next line to disable debug mode
//#undef DEBUG
int main() {
#ifndef DEBUG
printf("Debug mode is disabled.\n");
#else
printf("Debug mode is enabled.\n");
#endif
return 0;
}
In this example:
- The
DEBUG
macro is not defined initially. However, you can see that there’s a line//#undef DEBUG
, which is commented out. If you uncomment this line, it will undefine theDEBUG
macro. - Inside the program, the
#ifndef
directive checks whether theDEBUG
macro is not defined. Since it is not defined initially, the code within the#ifndef
block is included in compilation. - As a result, “Debug mode is disabled.” is printed when the program is executed.
The #ifndef
directive is often used for conditional compilation to include or exclude code based on the absence of specific macros. This can be useful for enabling or disabling features, debugging code, or defining different code paths based on configuration options.