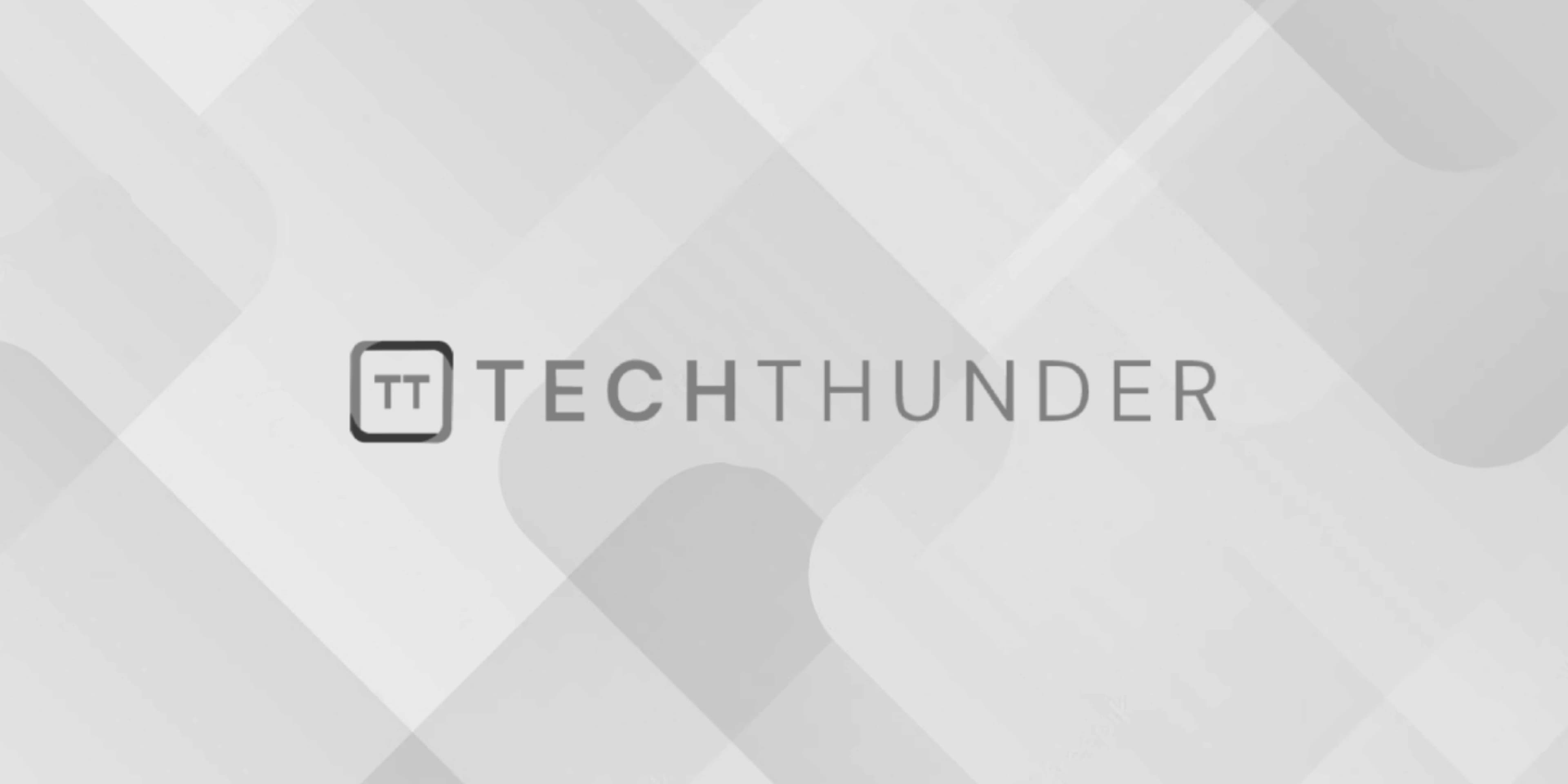
309 views
C for loop
The for
loop is a control structure that allows you to repeatedly execute a block of code a specific number of times. It is one of the three main types of loops in C, along with the while
loop and the do-while
loop. The for
loop is commonly used when you know the exact number of iterations needed. The syntax of a for
loop is as follows:
C
for (initialization; condition; increment/decrement) {
// Code to be executed in each iteration
}
Here’s how a for
loop works:
- Initialization: The loop starts with the initialization step. Here, you typically declare and initialize a loop control variable. This variable is used to keep track of the loop’s progress.
- Condition: After initialization, the condition is evaluated. If the condition is true, the loop’s body is executed; otherwise, the loop terminates.
- Loop Body: The code inside the loop body is executed if the condition is true. This is where you place the code that should be repeated.
- Increment/Decrement: After executing the loop body, the increment or decrement statement is executed. This step typically updates the loop control variable.
- Repeat: Steps 2 to 4 are repeated until the condition becomes false. When the condition is false, the loop terminates, and program execution continues with the code after the
for
loop.
Here’s an example of a simple for
loop that counts from 1 to 5 and prints the values:
C
#include <stdio.h>
int main() {
for (int i = 1; i <= 5; i++) {
printf("%d\n", i);
}
return 0;
}
In this example:
- The loop control variable
i
is initialized to 1. - The condition
i <= 5
is checked before each iteration. - The loop body prints the value of
i
. - The
i
variable is incremented by 1 in each iteration (i++
).
The output of this program will be:
Plaintext
1
2
3
4
5
You can use for
loops for various purposes, including iterating over arrays, generating sequences of numbers, and performing repetitive tasks with a known number of iterations.