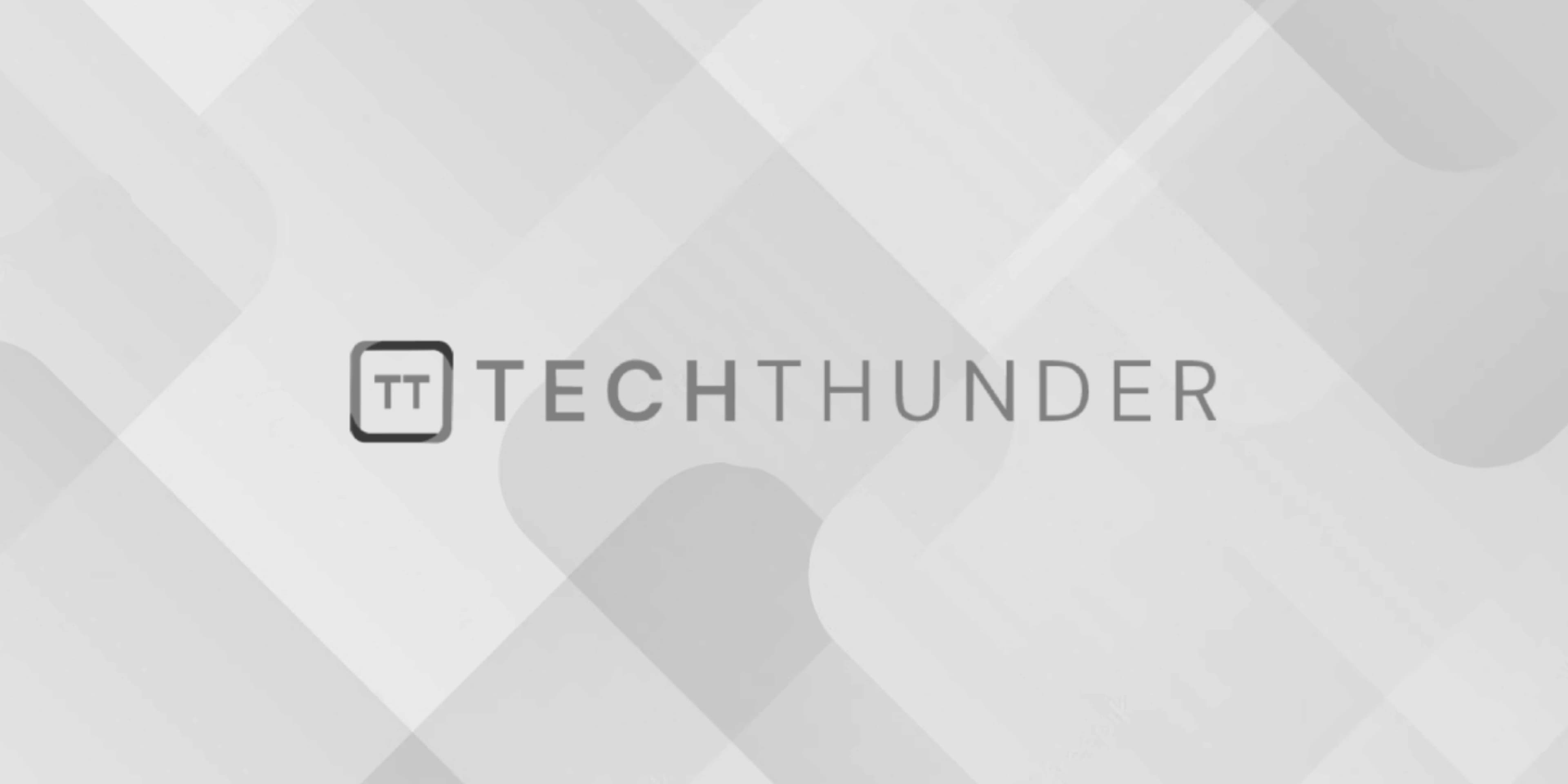
102 views
C fputs() fgets()
The C fputs()
and fgets()
are functions used for performing input and output operations on files. They are often used for reading and writing strings to and from files. Here’s an explanation of each function:
fputs()
Function:
fputs()
stands for “file put string.”- It is used to write a string to a specified file.
- The function signature is as follows:
int fputs(const char *str, FILE *stream);
str
: A pointer to the null-terminated string you want to write to the file.stream
: A pointer to aFILE
structure, which represents the file you want to write to.- Returns a non-negative value on success or
EOF
(end-of-file) on failure.
- Example usage:
C
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "w"); // Open the file for writing
if (file != NULL) {
const char *text = "Hello, world!";
if (fputs(text, file) != EOF) {
printf("Data written successfully.\n");
} else {
printf("Error writing to the file.\n");
}
fclose(file);
} else {
printf("Unable to open the file.\n");
}
return 0;
}
fgets()
Function:
fgets()
stands for “file get string.”- It is used to read a line (or a specified number of characters) from a file into a character array.
- The function signature is as follows:
char *fgets(char *str, int n, FILE *stream);
str
: A pointer to the character array where the read string will be stored.n
: The maximum number of characters to read (including the null terminator).stream
: A pointer to aFILE
structure, which represents the file you want to read from.- Returns a pointer to
str
on success,NULL
on failure or when the end of the file is reached.
- Example usage:
C
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "r"); // Open the file for reading
if (file != NULL) {
char buffer[100];
if (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("Read from the file: %s", buffer);
} else {
printf("Error reading from the file.\n");
}
fclose(file);
} else {
printf("Unable to open the file.\n");
}
return 0;
}
These functions provide a basic way to perform input and output operations on text files in C. Make sure to open files using fopen()
before using fputs()
or fgets()
, and close them using fclose()
when you’re done to free up resources and ensure data is written or read correctly.