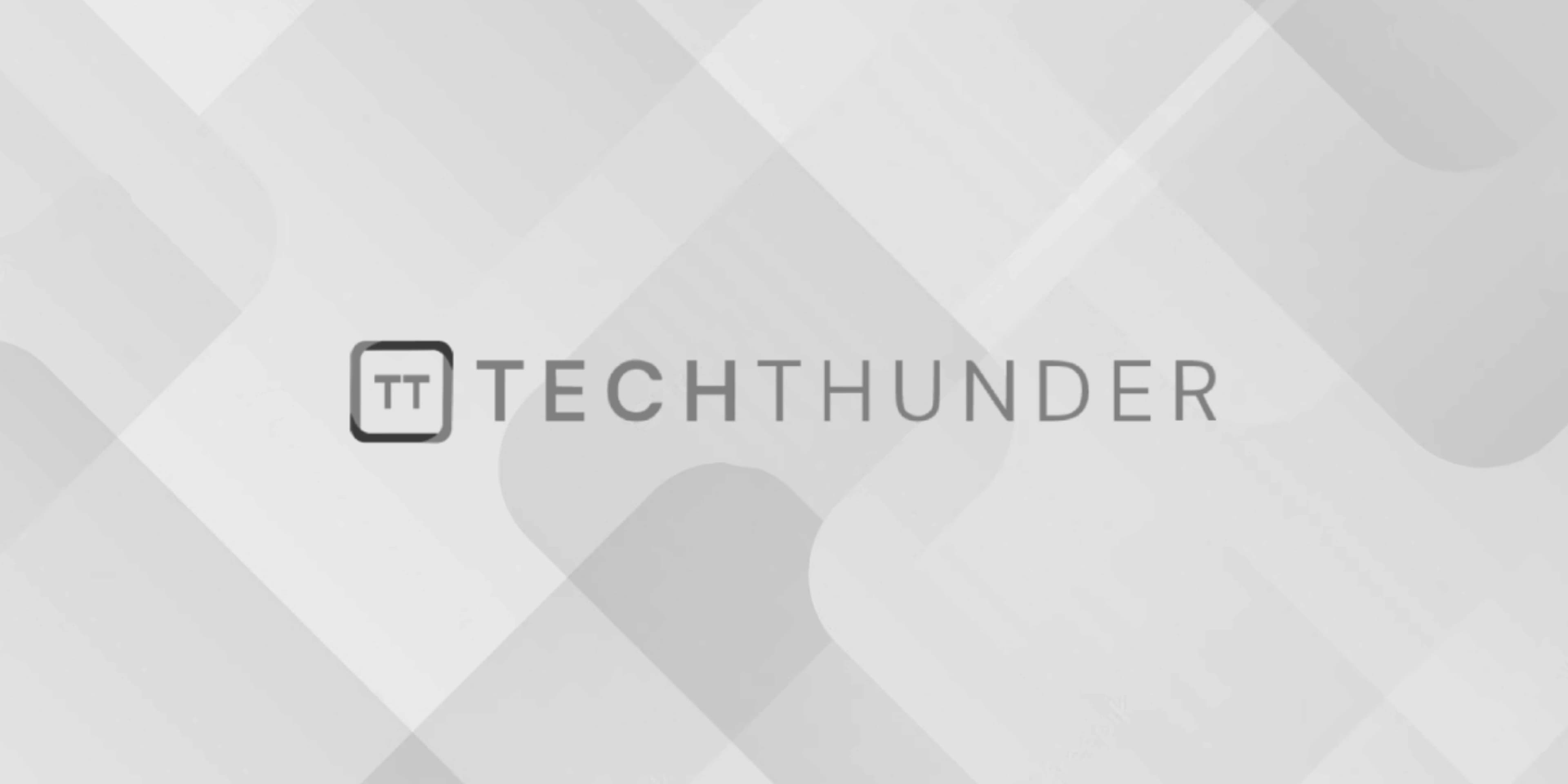
110 views
Find out Power without Using POW Function in C
You can calculate the power of a number without using the pow()
function by implementing a custom power function that uses a loop to repeatedly multiply the base number. Here’s an example in C:
C
#include <stdio.h>
// Function to calculate the power of a number
double power(double base, int exponent) {
double result = 1.0;
if (exponent > 0) {
for (int i = 0; i < exponent; i++) {
result *= base;
}
} else if (exponent < 0) {
for (int i = 0; i > exponent; i--) {
result /= base;
}
}
return result;
}
int main() {
double base;
int exponent;
printf("Enter the base: ");
scanf("%lf", &base);
printf("Enter the exponent: ");
scanf("%d", &exponent);
double result = power(base, exponent);
printf("Result: %.4lf\n", result);
return 0;
}
In this program:
- The
power
function calculates the power of a number by repeatedly multiplying (exponent > 0
) or dividing (exponent < 0
) thebase
number by itself. - The
main
function takes user input for thebase
andexponent
, calls thepower
function to calculate the result, and then prints the result.
This program allows you to calculate the power of a number without using the pow()
function. However, keep in mind that this implementation is relatively simple and may not handle all edge cases, such as very large exponents or negative non-integer exponents, which would require more advanced algorithms for precision and efficiency.