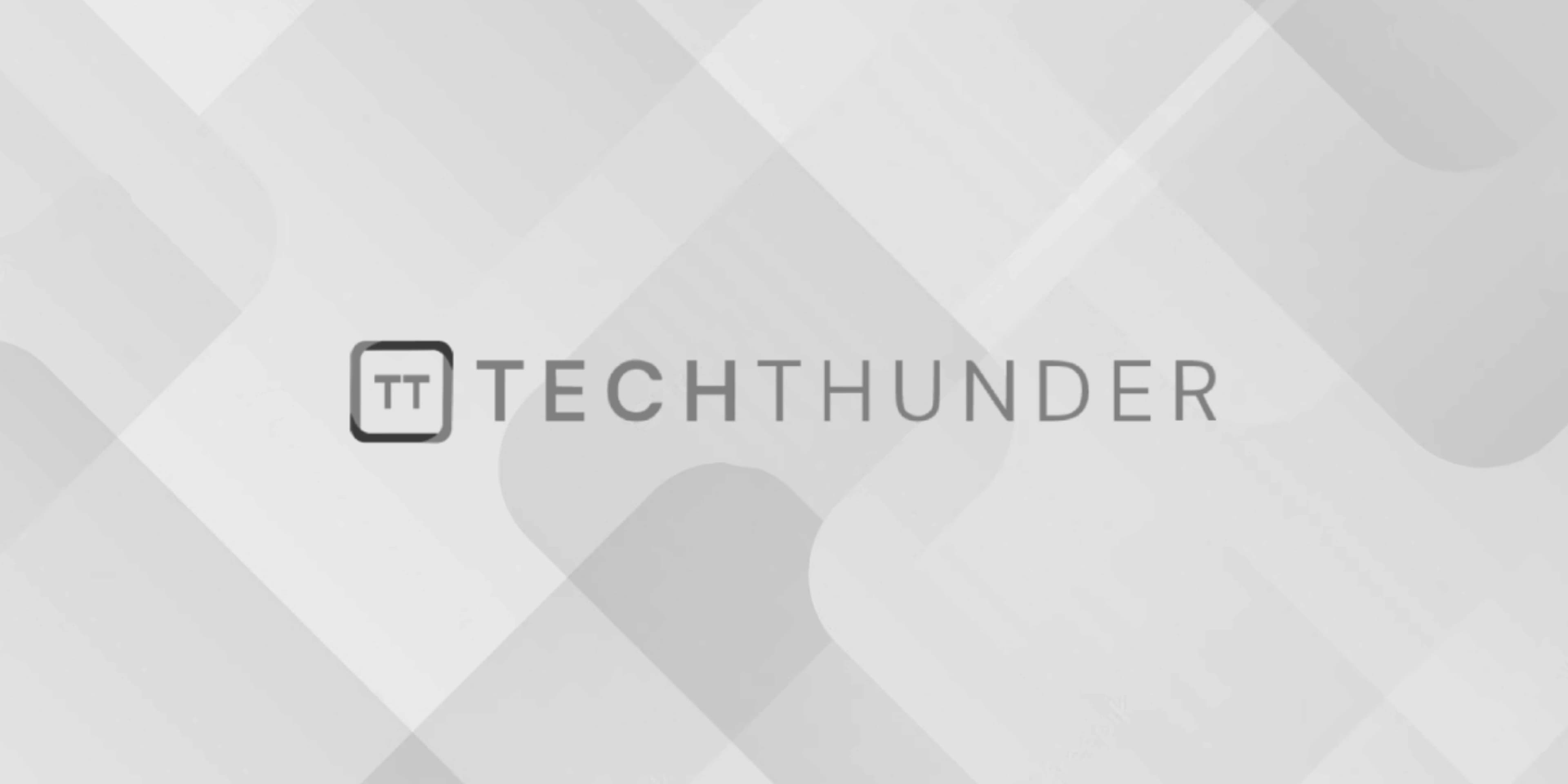
Top 10+ C Programs
The list of more than 10 C programs with brief descriptions:
1. Hello, World!
The classic introductory program that prints “Hello, World!” to the console.
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
2. Factorial
Computes the factorial of a given number using recursion or iteration.
3. Fibonacci Series
Generates the Fibonacci series up to a specified number of terms.
4. Prime Number Checker
Checks if a given number is prime or not.
5. Palindrome Checker
Determines whether a given string or number is a palindrome (reads the same forwards and backward).
6. Matrix Multiplication
Multiplies two matrices and prints the result.
7. Reverse a String
Reverses a string, such as “Hello” becoming “olleH.”
8.Sum of Digits
Calculates the sum of digits of a given number.
9. Armstrong Number
Checks if a number is an Armstrong number (a number that is equal to the sum of its own digits each raised to the power of the number of digits).
10. Factorial of a Number
Calculates the factorial of a given positive integer.
11. GCD (Greatest Common Divisor)
Computes the greatest common divisor of two numbers using the Euclidean algorithm.
12. Binary to Decimal Conversion
Converts a binary number to its decimal equivalent.
13. Decimal to Binary Conversion
Converts a decimal number to its binary equivalent.
14. String Concatenation
Concatenates two strings.
15. Counting the Number of Words in a String
Counts the number of words in a given string.
16. Sum of an Array
Calculates the sum of elements in an integer array.
17. Search for an Element in an Array
Searches for a specific element in an array and returns its index.
18. Bubble Sort
Implements the bubble sort algorithm to sort an array of integers.
19. File Handling
Reads from and writes to a text file.
20. Linked List Operations
Implements various operations on a singly linked list, such as insertion, deletion, and traversal.
These programs cover a wide range of basic and intermediate C programming concepts and can be used for practice and learning purposes.