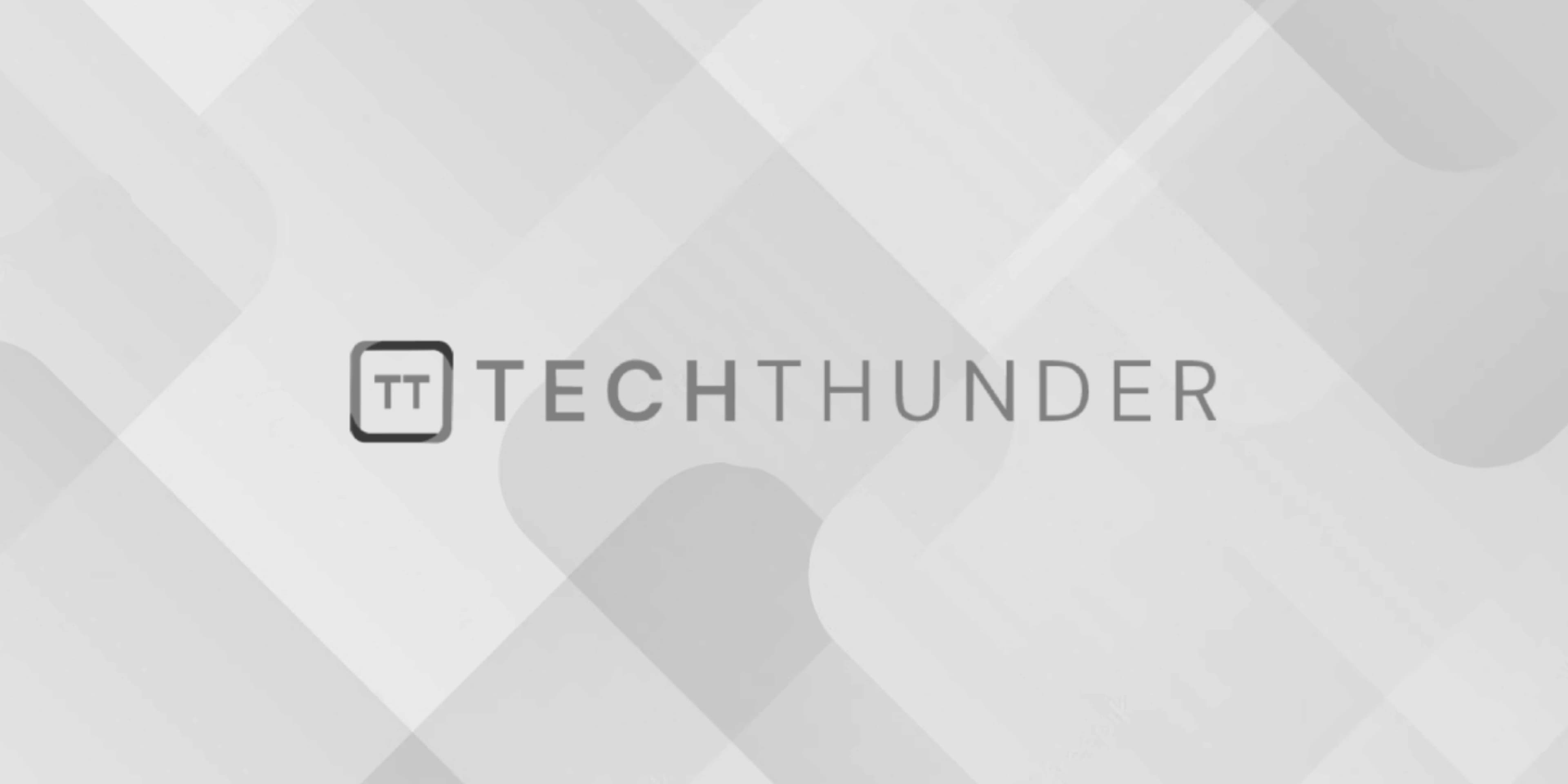
195 views
Variables vs Constants in C
The variables and constants serve different purposes and have distinct characteristics:
Variables:
- Mutable: Variables are storage locations in memory that can hold data, and their values can be changed during program execution. You can assign new values to variables as needed.
- Declared using Data Types: Variables are declared using data types (e.g.,
int
,float
,char
) that specify the type of data they can store. The data type determines the range and format of values the variable can hold. - Memory Allocation: Variables consume memory space, and the amount of memory allocated depends on the data type. For example, an
int
typically requires 4 bytes of memory. - Initialization: Variables may or may not be initialized when declared. If not initialized, they may contain garbage values.
- Scope: Variables have a scope that defines where they can be accessed. Common scopes include local (within a function), global (accessible throughout the program), and block scope (within a block of code).
- Lifetime: Variables have a lifetime that determines when they are created and destroyed. Local variables are created when the function is called and destroyed when the function exits. Global variables typically last throughout the program’s execution.
Constants:
- Immutable: Constants are values that remain fixed throughout the program’s execution. Once defined, their values cannot be changed.
- Declared using Keywords: Constants in C are declared using keywords like
const
or by using preprocessor macros (e.g.,#define
). - No Memory Allocation: Constants do not consume memory space at runtime. They are typically replaced with their literal values during compilation.
- Must Be Initialized: Constants must be initialized when declared. You cannot create an uninitialized constant.
- Scope: Constants can have different scopes, including local and global, depending on how they are declared.
- Lifetime: Constants exist for the entire duration of the program, similar to global variables.
Example:
C
#include <stdio.h>
// Constants
#define PI 3.14159265359
const int MAX_VALUE = 100;
int main() {
// Variables
int x = 5;
float y = 2.5;
// Constants
const int MIN_VALUE = 0;
// Usage
x = x + 1; // Variable can be modified
// PI = 3.14; // Error: Cannot modify constant
printf("x = %d\n", x);
printf("PI = %f\n", PI);
return 0;
}
In this example, x
and y
are variables, and PI
, MAX_VALUE
, and MIN_VALUE
are constants. You can see that variables can be modified, while constants are immutable. Constants are typically used to define values that should not change during the program’s execution, providing clarity and preventing accidental modifications.