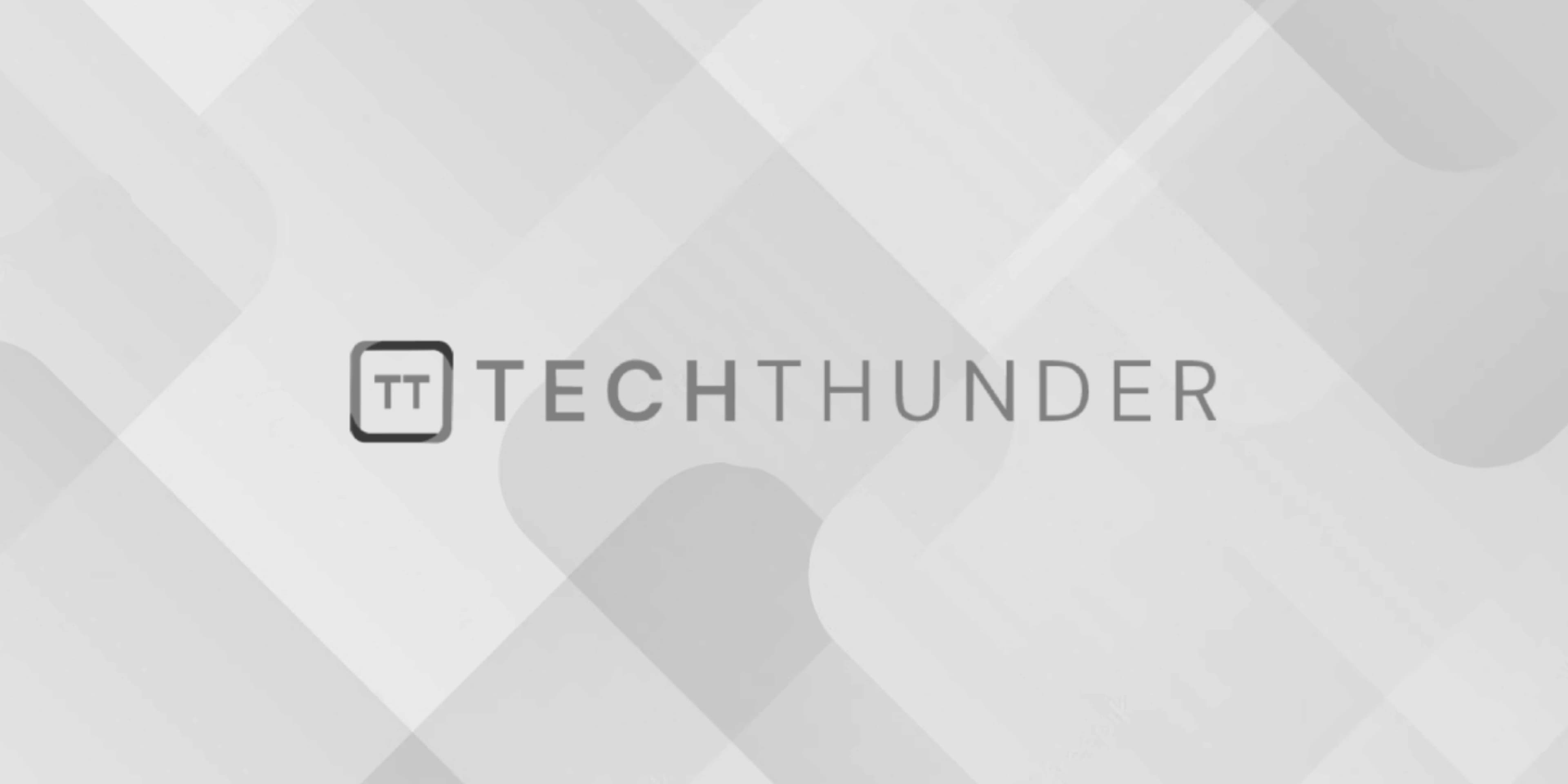
C Pointer Arithmetic
Pointer arithmetic is a fundamental concept in C, allowing you to manipulate memory addresses through pointers. C provides a simple and powerful mechanism for performing arithmetic operations on pointers to navigate arrays, structures, and other data structures efficiently. Pointer arithmetic involves adding or subtracting integers to/from pointer values to access elements or move through memory.
Here are some common pointer arithmetic operations in C:
- Increment and Decrement:
- You can increment and decrement pointers to move through an array or a memory block. Incrementing a pointer of type
T
advances it bysizeof(T)
bytes.
int arr[] = {10, 20, 30, 40};
int *ptr = arr;
ptr++; // Moves to the next element
- Indexing Arrays:
- You can use pointer arithmetic to access elements of an array. Instead of using array notation, you can use pointer arithmetic to achieve the same result.
int arr[] = {10, 20, 30, 40};
int *ptr = arr;
int secondElement = *(ptr + 1); // Accesses the second element (20)
- Subtracting Pointers:
- Subtracting one pointer from another of the same type gives you the number of elements between them. This is commonly used to calculate the length or the offset of data structures.
int arr[] = {10, 20, 30, 40};
int *ptr1 = &arr[0];
int *ptr2 = &arr[2];
int distance = ptr2 - ptr1; // Calculates the number of elements between ptr1 and ptr2 (2)
- Pointer Arithmetic with Structures:
- You can use pointer arithmetic to navigate through structures when they are stored in contiguous memory.
struct Point {
int x;
int y;
};
struct Point points[3];
struct Point *ptr = points;
int yCoordinate = (ptr + 2)->y; // Accesses the y-coordinate of the third point
- Character Pointers (Strings):
- Pointer arithmetic is commonly used with character pointers (strings) to traverse and manipulate strings.
char str[] = "Hello";
char *ptr = str;
while (*ptr != '\0') {
printf("%c", *ptr);
ptr++;
}
Pointer arithmetic is a powerful tool in C, but it should be used carefully to avoid undefined behavior. Common pitfalls include accessing memory beyond the bounds of an array or dereferencing uninitialized or invalid pointers. Careful bounds checking and validation of pointer values are essential for writing safe and correct C code.