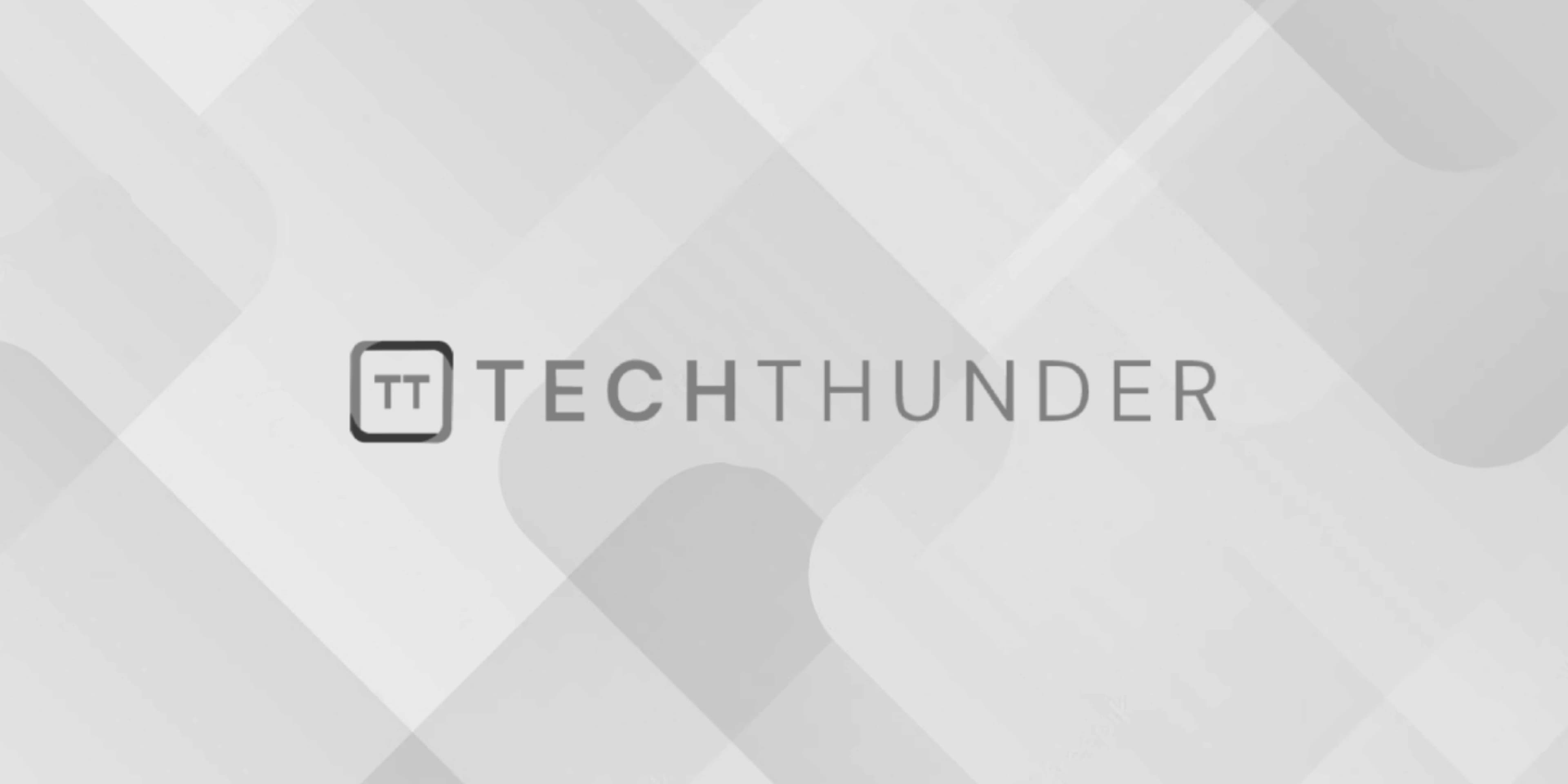
Integer Promotions in C
The integer promotions are a set of rules used by the C compiler to promote certain types of integer values to a common type before performing certain operations. These rules help ensure that expressions involving mixed types are handled consistently, according to the C language standard.
The integer promotions include the following rules:
- If an
int
can represent all values of the original type (as defined by the C standard), the value is converted toint
. This means thatchar
andshort
values are promoted toint
if anint
can represent all possible values of the original type. If not, they are promoted tounsigned int
. - If
int
cannot represent all values of the original type (e.g., ifchar
is signed and the range ofint
is not sufficient), the value is converted tounsigned int
. - If the original type is
unsigned int
, it remainsunsigned int
. - If the original type is a bit field (a type defined with the
bit-field
declarator), it’s promoted toint
if it can represent all possible values of the original type; otherwise, it’s promoted tounsigned int
.
The primary purpose of integer promotions is to ensure that expressions involving mixed types are handled consistently, and that integer arithmetic follows a predictable set of rules. This helps prevent unexpected behavior and ensures that C code behaves consistently across different platforms.
Here’s an example that demonstrates integer promotions:
#include <stdio.h>
int main() {
char a = 100;
short b = 200;
int result;
// The following expression involves integer promotions:
result = a + b;
printf("Result: %d\n", result);
return 0;
}
In this example:
char
variablea
is promoted toint
.short
variableb
is promoted toint
.- The addition operation is performed with two
int
values. - The result is an
int
value, which is printed usingprintf
.
In general, understanding integer promotions is essential for writing correct and portable C code, especially when dealing with mixed types in expressions.