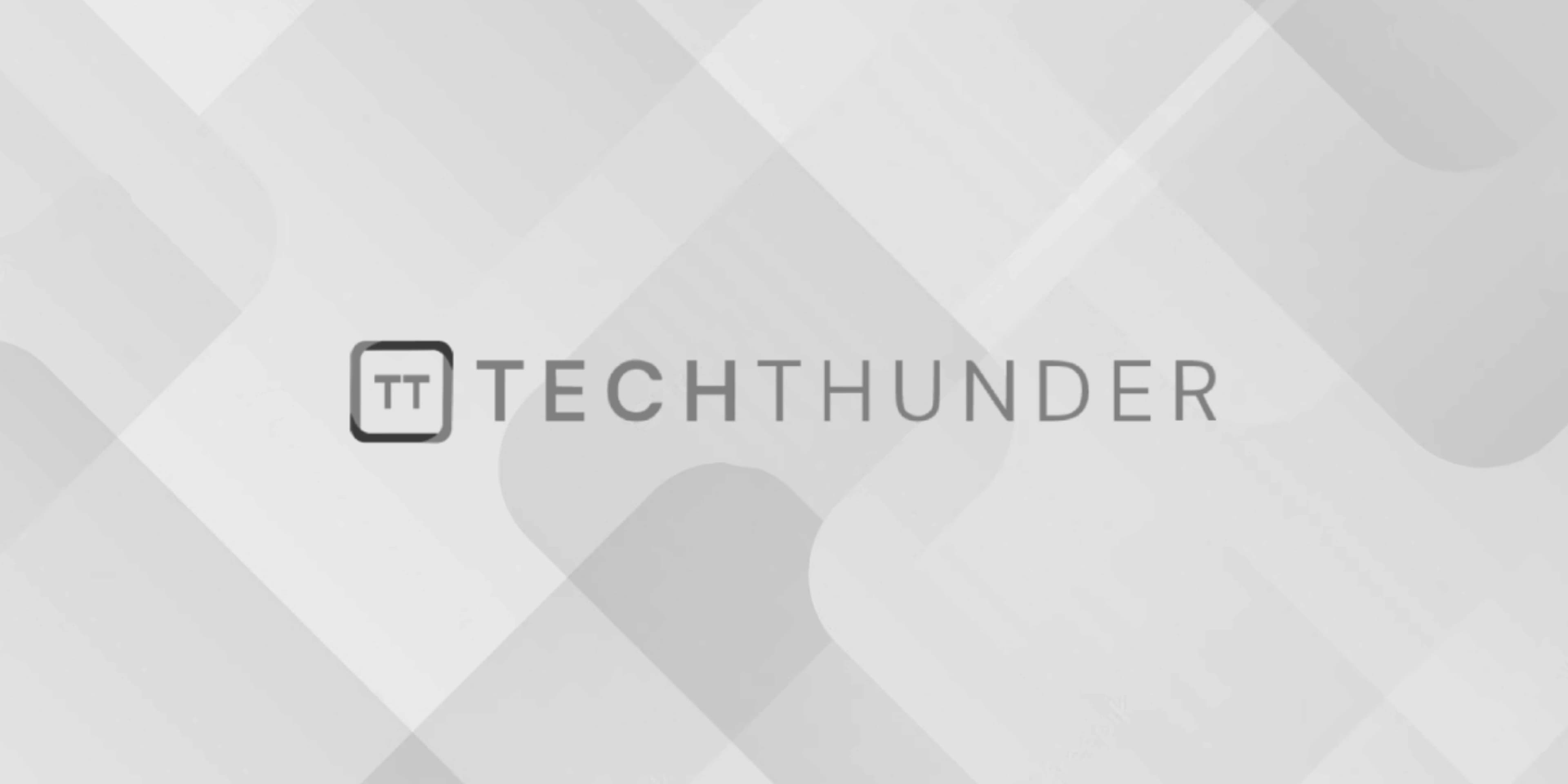
strchr() function in C
The strchr()
function in C is used to search for the first occurrence of a specific character within a null-terminated string. It is part of the C Standard Library and is declared in the <string.h>
header.
Here’s the syntax of the strchr()
function:
char *strchr(const char *str, int character);
str
: A pointer to the null-terminated string in which you want to search for the character.character
: An integer representing the character you want to search for.
The function returns a pointer to the first occurrence of the specified character within the string. If the character is not found, it returns a null pointer (NULL
).
Here’s an example of how to use the strchr()
function:
#include <stdio.h>
#include <string.h>
int main() {
const char *str = "Hello, World!";
char target = 'o';
// Search for the first occurrence of 'o' in the string
char *result = strchr(str, target);
if (result != NULL) {
printf("'%c' found at position %ld\n", target, result - str);
} else {
printf("'%c' not found in the string.\n", target);
}
return 0;
}
In this example:
- We have a string
str
containing the text “Hello, World!” and a charactertarget
(‘o’) that we want to search for. - We call
strchr(str, target)
to search for the first occurrence of ‘o’ in the string. - If the character is found, the function returns a pointer to the ‘o’ character in the string, and we calculate its position by subtracting
str
from the result pointer. - If the character is not found, the function returns
NULL
, indicating that the character does not exist in the string.
Output (in this case):
'o' found at position 4
Keep in mind that strchr()
only finds the first occurrence of the specified character. If you want to find subsequent occurrences, you can continue searching from the next character after the found occurrence. Additionally, there is a related function called strrchr()
that searches for the last occurrence of a character in a string.