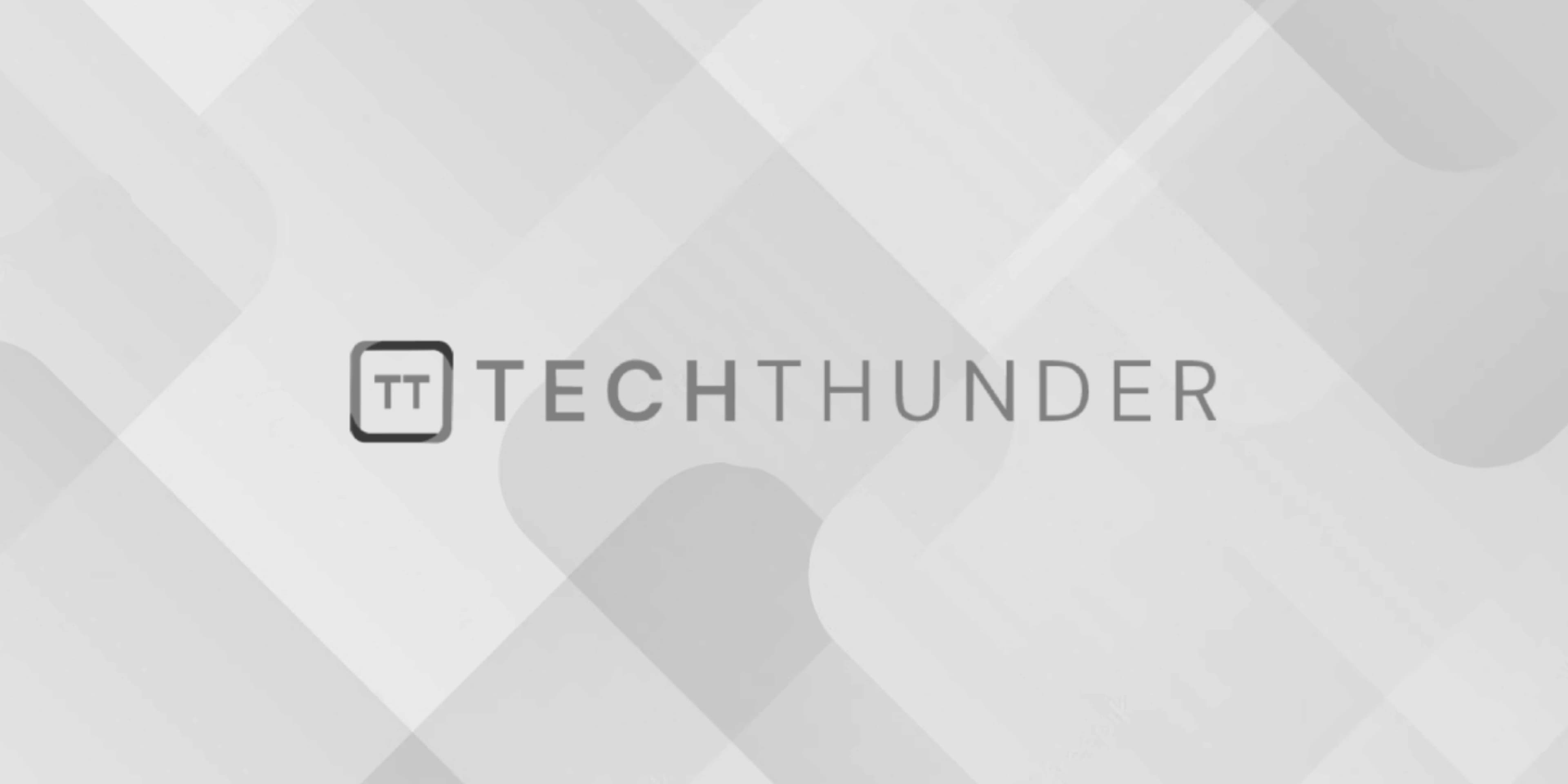
53 views
C Format Specifier
The format specifiers are used in functions like printf
and scanf
to specify the format of input or output data. Format specifiers define how the data should be interpreted or displayed. Here are some commonly used format specifiers in C:
- Integer Format Specifiers:
%d
: Signed integer (decimal).%u
: Unsigned integer (decimal).%x
: Unsigned integer (hexadecimal).%o
: Unsigned integer (octal).
C
int num = 42;
printf("Decimal: %d, Hexadecimal: %x, Octal: %o\n", num, num, num);
- Floating-Point Format Specifiers:
%f
: Floating-point number (decimal notation).%e
or%E
: Floating-point number (scientific notation).%g
or%G
: Floating-point number (compact notation).
C
float pi = 3.14159265359;
printf("Decimal: %f, Scientific: %e, Compact: %g\n", pi, pi, pi);
- Character Format Specifiers:
%c
: Single character.%s
: String (array of characters).
C
char ch = 'A';
char name[] = "John";
printf("Character: %c, String: %s\n", ch, name);
- Pointer Format Specifier:
%p
: Pointer address.
C
int value = 42;
int *ptr = &value;
printf("Pointer Address: %p\n", (void *)ptr);
- Width and Precision Specifiers:
%*d
: Specifies width (e.g.,%5d
for a minimum width of 5).%.2f
: Specifies precision (e.g.,%.2f
for 2 decimal places).
C
int num = 42;
float pi = 3.14159265359;
printf("Width: %5d, Precision: %.2f\n", num, pi);
- Other Format Specifiers:
%ld
: Long integer.%lu
: Unsigned long integer.%lld
: Long long integer.%llu
: Unsigned long long integer.%c
: Character.%s
: String.%lf
: Double-precision floating-point number.%Lf
: Long double-precision floating-point number.
C
long num1 = 1234567890L;
unsigned long num2 = 9876543210UL;
long long num3 = 123456789012345LL;
unsigned long long num4 = 987654321098765ULL;
printf("Long: %ld, Unsigned Long: %lu\n", num1, num2);
printf("Long Long: %lld, Unsigned Long Long: %llu\n", num3, num4);
These format specifiers are used in functions like printf
for formatting output and in scanf
for formatting input. Using the correct format specifier is important to ensure that data is correctly interpreted or displayed. Incorrect format specifiers can lead to undefined behavior or incorrect results.