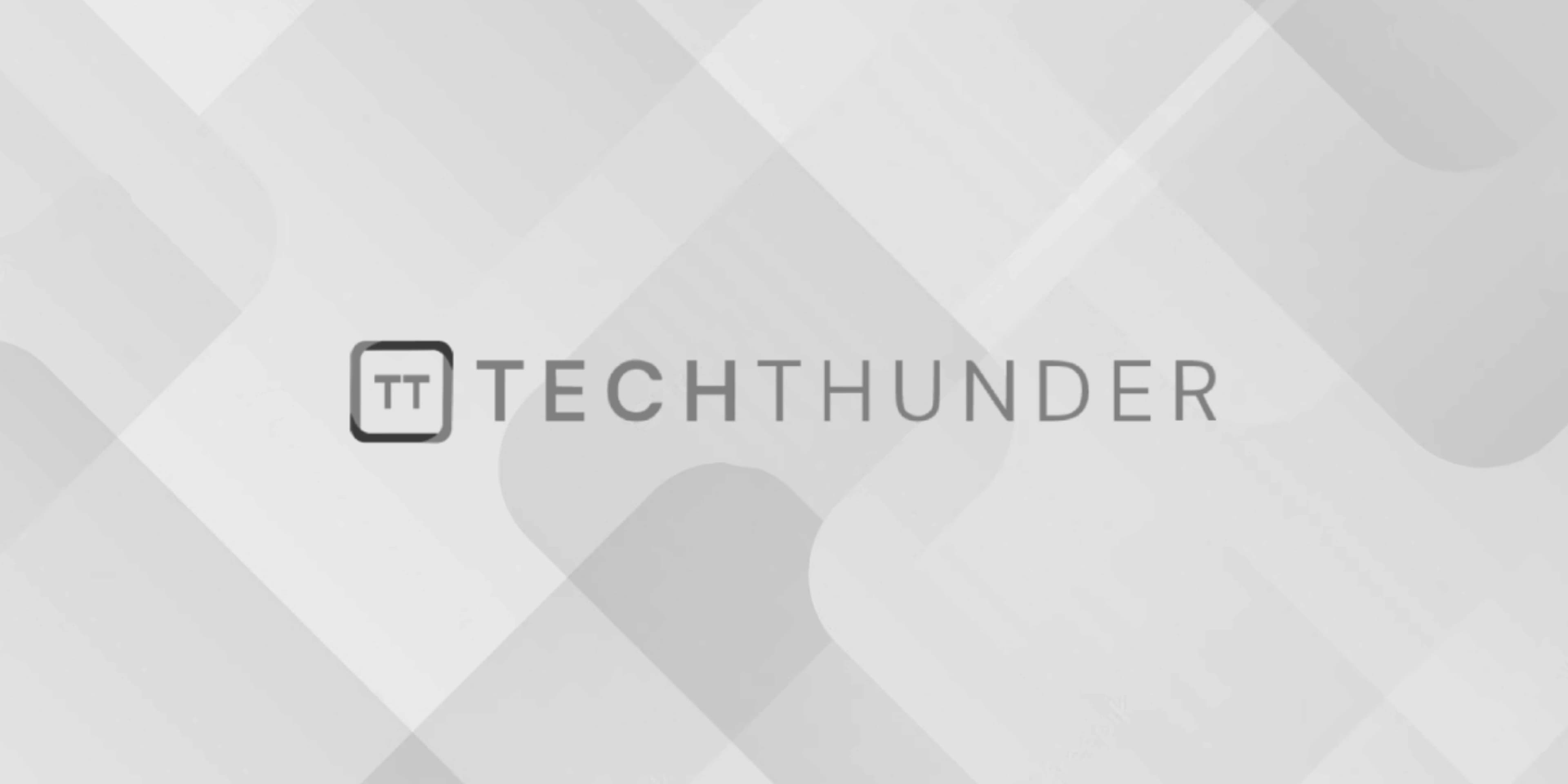
C #undef
The #undef
directive is used to undefine (remove) a previously defined macro. Macros are defined using the #define
directive, and they can be undefined using #undef
. This is useful when you want to remove or “undefine” a macro that you no longer need or want to use in your code.
Here’s the basic syntax of the #undef
directive:
#undef macro_name
macro_name
is the name of the macro that you want to undefine.
For example, suppose you have defined a macro called DEBUG
like this:
#define DEBUG
You can later undefine it using the #undef
directive:
#undef DEBUG
After this #undef
directive, the DEBUG
macro is no longer defined, and any subsequent use of DEBUG
in the code will not result in macro expansion.
Here’s an example of how #undef
can be used:
#include <stdio.h>
#define DEBUG // Define DEBUG macro
int main() {
#ifdef DEBUG
printf("Debug mode is enabled.\n");
#else
printf("Debug mode is disabled.\n");
#endif
#undef DEBUG // Undefine DEBUG macro
#ifdef DEBUG
printf("This will not be printed because DEBUG is undefined.\n");
#else
printf("Debug mode is still disabled.\n");
#endif
return 0;
}
In this example:
- The
DEBUG
macro is defined at the beginning of the program using#define
. - Inside the program, conditional compilation (
#ifdef
and#else
) is used to check whetherDEBUG
is defined and print different messages accordingly. - After the first conditional block, the
#undef DEBUG
directive is used to undefine theDEBUG
macro. - In the second conditional block, it’s confirmed that
DEBUG
is indeed undefined, and the corresponding message is printed.
By using #undef
, you can selectively remove macros from your code when they are no longer needed or when their behavior should change. This can help manage code complexity and configuration in C programs.