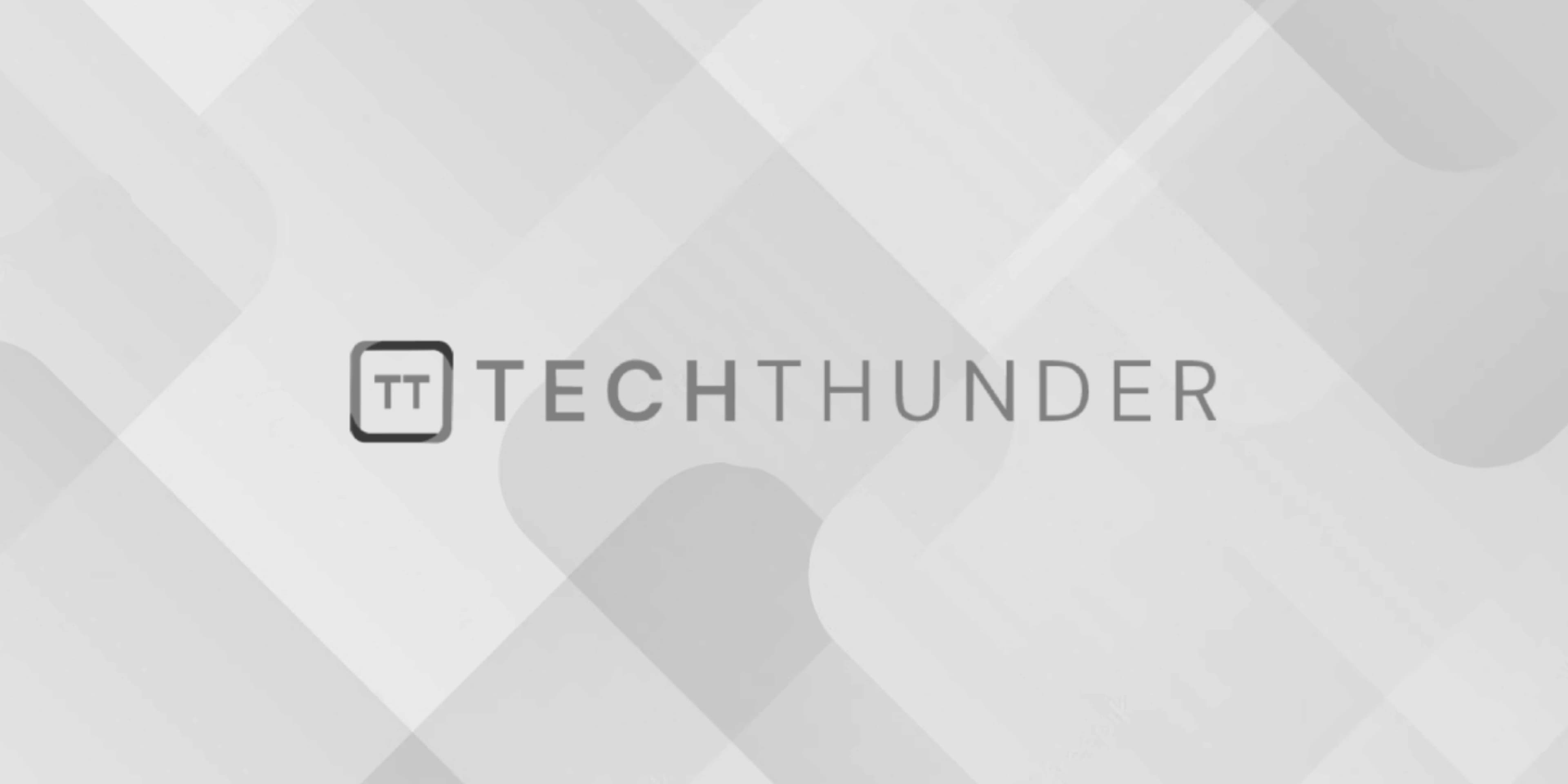
Simpson Method
The Simpson’s method, also known as Simpson’s rule or Simpson’s 1/3 rule, is a numerical integration technique used to approximate the definite integral of a function. It’s a rule for approximating the area under a curve by dividing it into a series of trapezoids and parabolas. Simpson’s method is a more accurate alternative to the simpler trapezoidal rule for numerical integration.
Here’s the basic idea of Simpson’s method:
- Divide the interval over which you want to find the definite integral into an even number of subintervals (typically 2, 4, 6, etc.).
- Calculate the width of each subinterval (h) by dividing the total interval width (b – a) by the number of subintervals.
- Approximate the integral within each pair of adjacent subintervals using a parabolic (quadratic) function. This is done by fitting a quadratic polynomial to the three function values at the interval boundaries and the midpoint of the subinterval.
- Calculate the area under each parabola within each subinterval using the quadratic formula for integration.
- Sum up the areas of all the subintervals to obtain the approximate value of the definite integral.
The formula for Simpson’s rule with an even number of subintervals is:
[ \text{Approximate Integral} \approx \frac{h}{3} \left[ f(x_0) + 4f(x_1) + 2f(x_2) + 4f(x_3) + 2f(x_4) + \ldots + 4f(x_{n-1}) + f(x_n) \right] ]
Where:
- (n) is the number of subintervals (an even number).
- (h) is the width of each subinterval, calculated as (\frac{b – a}{n}).
- (x_i) represents the boundary points of the subintervals.
In practice, Simpson’s method is often implemented as a loop, and you sum up the values of the function at the boundary points and the midpoint of each subinterval as per the formula above.
Here’s a simplified example of implementing Simpson’s method in C to approximate the definite integral of a function:
#include <stdio.h>
#include <math.h>
// Function to integrate
double f(double x) {
return x * x; // Replace with your function
}
int main() {
double a, b, h, integral;
int n, i;
printf("Enter lower limit (a): ");
scanf("%lf", &a);
printf("Enter upper limit (b): ");
scanf("%lf", &b);
printf("Enter number of subintervals (even): ");
scanf("%d", &n);
h = (b - a) / n;
integral = f(a) + f(b); // Initialize with boundary values
for (i = 1; i < n; i++) {
if (i % 2 == 0) {
integral += 2 * f(a + i * h); // Even subintervals
} else {
integral += 4 * f(a + i * h); // Odd subintervals
}
}
integral *= h / 3.0; // Multiply by (h/3) as per Simpson's rule
printf("Approximate Integral: %lf\n", integral);
return 0;
}
This program calculates the definite integral of the function (x^2) over a specified interval using Simpson’s rule. You can replace the f()
function with your own function to compute the integral of different functions.