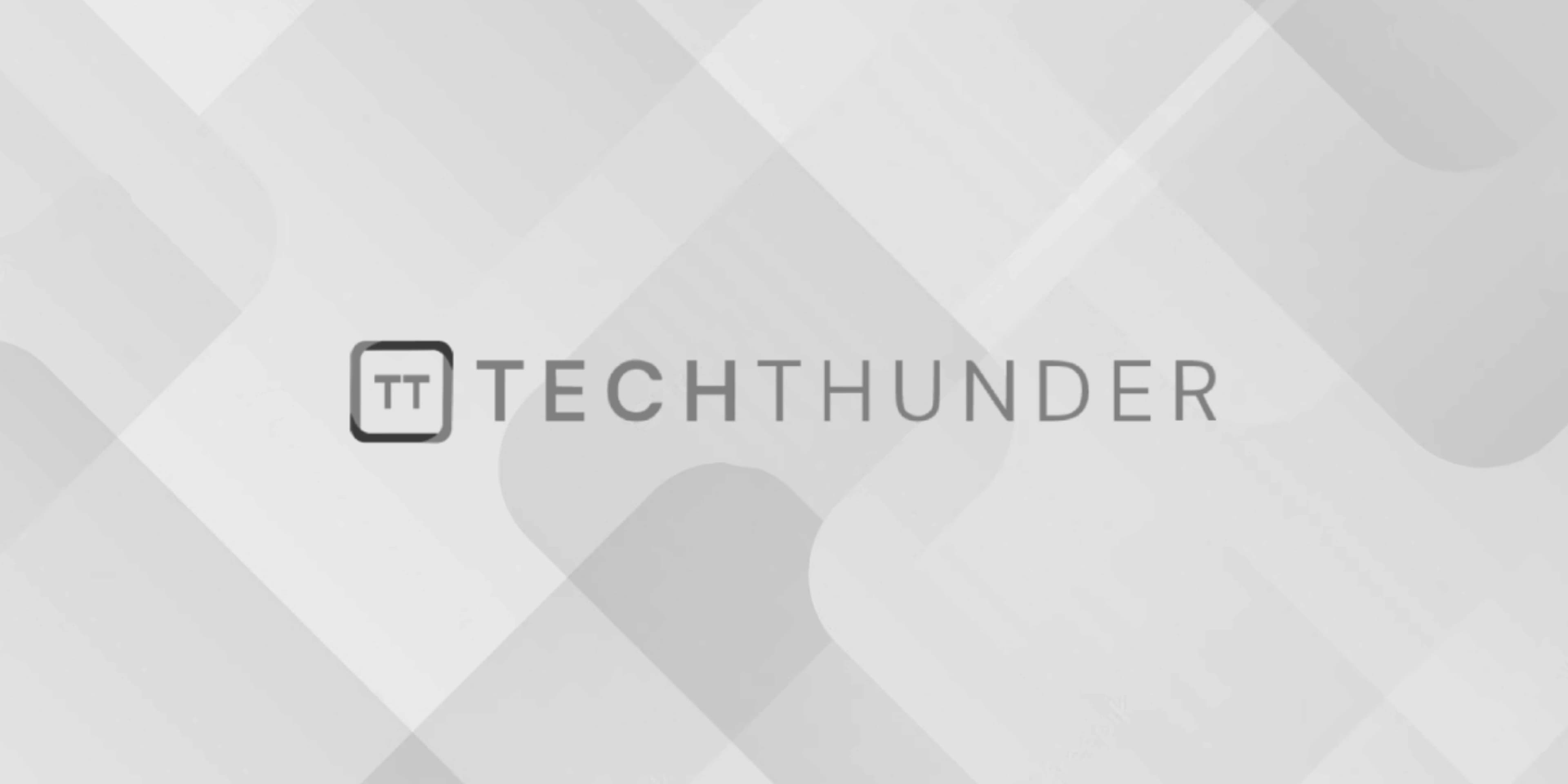
200 views
FCFS Program in C
FCFS (First-Come, First-Served) is a simple CPU scheduling algorithm in which the process that arrives first is allocated the CPU first. Here’s a C program that simulates the FCFS scheduling algorithm:
C
#include <stdio.h>
struct Process {
int id; // Process ID
int arrival; // Arrival time
int burst; // Burst time
int waiting; // Waiting time
int turnaround; // Turnaround time
};
// Function to calculate waiting time and turnaround time for each process
void calculateTimes(struct Process processes[], int n) {
int total_waiting = 0;
int total_turnaround = 0;
// First process has 0 waiting time
processes[0].waiting = 0;
processes[0].turnaround = processes[0].burst;
for (int i = 1; i < n; i++) {
// Waiting time for the current process is the turnaround time of the previous process
processes[i].waiting = processes[i - 1].turnaround;
// Turnaround time for the current process is waiting time + burst time
processes[i].turnaround = processes[i].waiting + processes[i].burst;
total_waiting += processes[i].waiting;
total_turnaround += processes[i].turnaround;
}
// Calculate average waiting time and average turnaround time
double avg_waiting = (double)total_waiting / n;
double avg_turnaround = (double)total_turnaround / n;
// Print the results
printf("Process\tArrival Time\tBurst Time\tWaiting Time\tTurnaround Time\n");
for (int i = 0; i < n; i++) {
printf("%d\t%d\t\t%d\t\t%d\t\t%d\n", processes[i].id, processes[i].arrival, processes[i].burst, processes[i].waiting, processes[i].turnaround);
}
printf("Average Waiting Time: %.2lf\n", avg_waiting);
printf("Average Turnaround Time: %.2lf\n", avg_turnaround);
}
int main() {
int n; // Number of processes
printf("Enter the number of processes: ");
scanf("%d", &n);
struct Process processes[n];
// Input process details
for (int i = 0; i < n; i++) {
processes[i].id = i + 1;
printf("Enter Arrival Time for Process %d: ", i + 1);
scanf("%d", &processes[i].arrival);
printf("Enter Burst Time for Process %d: ", i + 1);
scanf("%d", &processes[i].burst);
}
// Sort processes based on arrival time (FCFS)
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (processes[j].arrival > processes[j + 1].arrival) {
struct Process temp = processes[j];
processes[j] = processes[j + 1];
processes[j + 1] = temp;
}
}
}
// Calculate waiting time and turnaround time
calculateTimes(processes, n);
return 0;
}
In this program:
- We define a
struct Process
to represent each process with its ID, arrival time, burst time, waiting time, and turnaround time. - The
calculateTimes
function calculates the waiting time and turnaround time for each process and computes the average waiting time and average turnaround time. - In the
main
function, we input the details of each process, sort them based on their arrival times in ascending order (FCFS), and then callcalculateTimes
to calculate and display the times for each process.
You can run this program with different sets of process arrival and burst times to see how FCFS scheduling works and how it calculates waiting and turnaround times for each process.