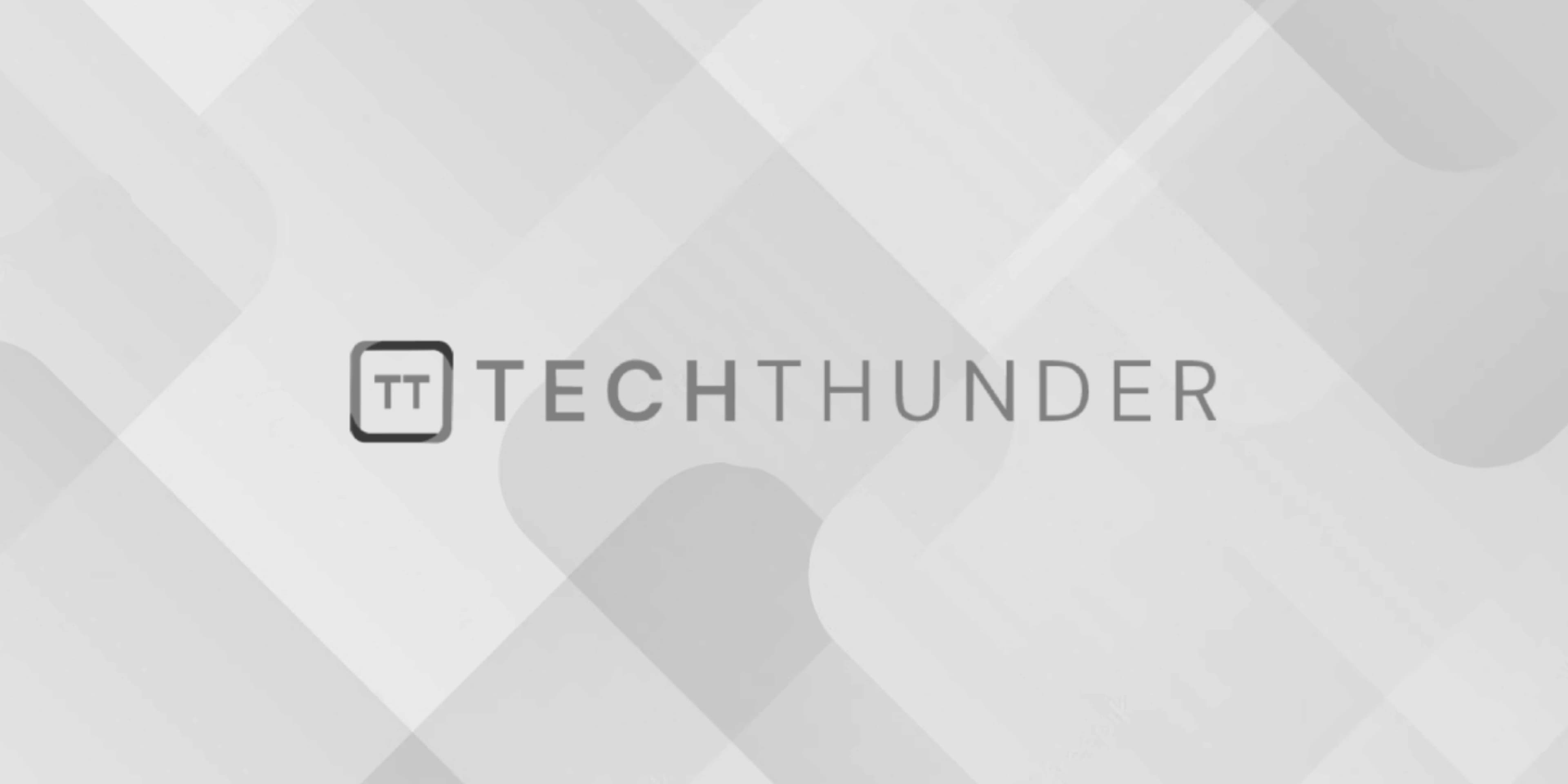
165 views
Number of even and odd numbers into given range in C
You can create a C program to count the number of even and odd numbers within a given range. Here’s an example:
C
#include <stdio.h>
int main() {
int start, end;
int evenCount = 0, oddCount = 0;
printf("Enter the starting number: ");
scanf("%d", &start);
printf("Enter the ending number: ");
scanf("%d", &end);
// Ensure that the start number is even if it's smaller
if (start % 2 != 0) {
start++;
}
// Count even and odd numbers within the range
for (int i = start; i <= end; i += 2) {
evenCount++;
}
for (int i = start + 1; i <= end; i += 2) {
oddCount++;
}
printf("Number of even numbers in the range: %d\n", evenCount);
printf("Number of odd numbers in the range: %d\n", oddCount);
return 0;
}
In this program:
- You input the starting and ending numbers to define the range.
- The program uses a loop to iterate through the range, starting from the nearest even number (if the start number is odd) and counting both even and odd numbers within the range.
- The counts of even and odd numbers are displayed as output.
This program calculates the counts of even and odd numbers in the specified range, considering both the starting and ending numbers, inclusive.