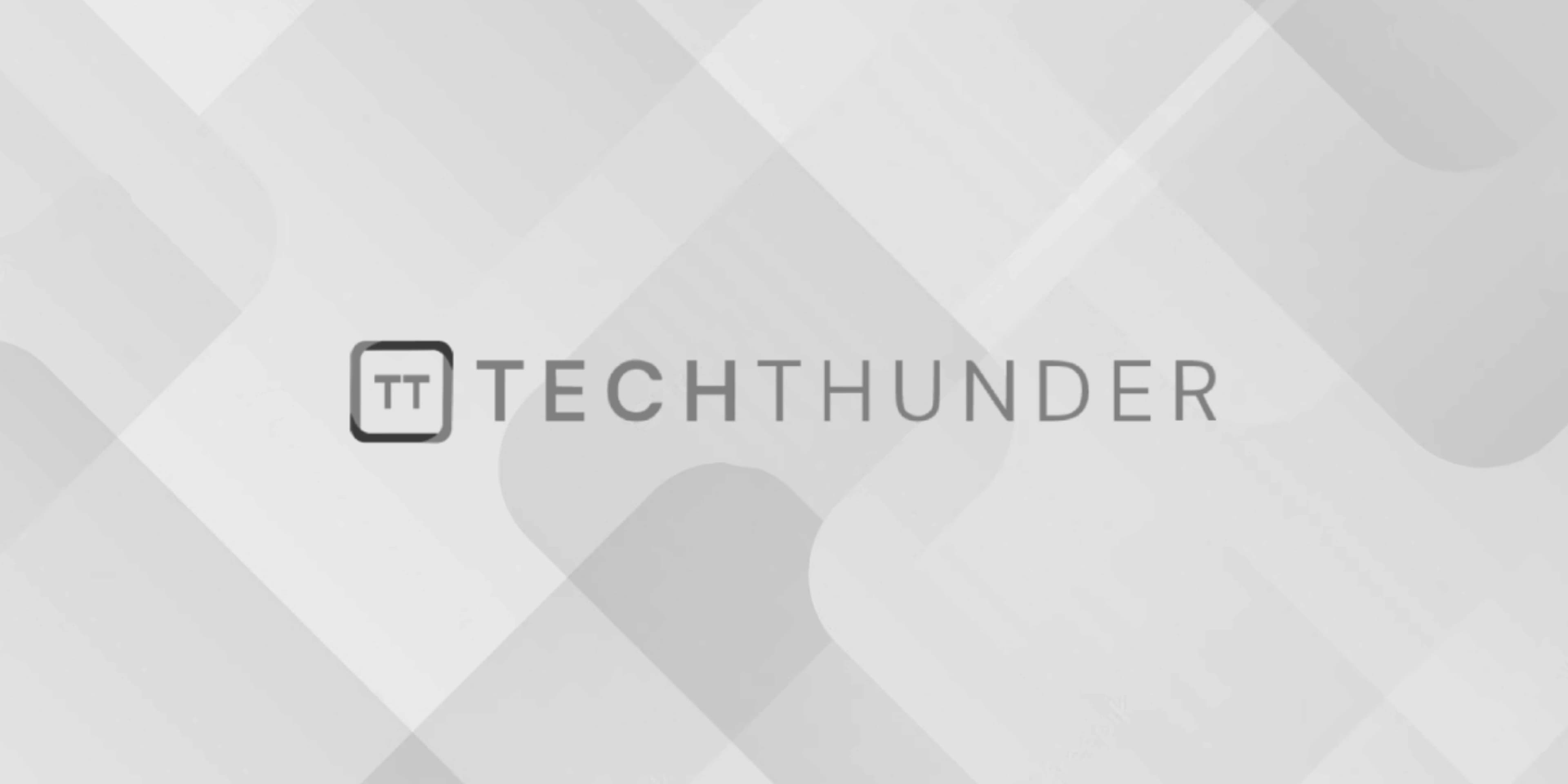
209 views
Binary to Decimal Number in C
To convert a binary number to a decimal number in C, you can follow a simple algorithm that involves iterating through the binary digits from right to left and multiplying each digit by the appropriate power of 2. Here’s a C program to perform this conversion:
C
#include <stdio.h>
#include <math.h>
int binaryToDecimal(long long binaryNumber) {
int decimalNumber = 0, i = 0, remainder;
while (binaryNumber != 0) {
remainder = binaryNumber % 10;
binaryNumber /= 10;
decimalNumber += remainder * pow(2, i);
++i;
}
return decimalNumber;
}
int main() {
long long binaryNumber;
printf("Enter a binary number: ");
scanf("%lld", &binaryNumber);
int decimalNumber = binaryToDecimal(binaryNumber);
printf("Decimal equivalent: %d\n", decimalNumber);
return 0;
}
In this program:
- We define a function
binaryToDecimal
that takes along long
integer representing a binary number as input and returns its decimal equivalent. - Inside the
binaryToDecimal
function, we initializedecimalNumber
to 0 to store the result andi
to 0 to keep track of the position of each binary digit. - We use a
while
loop to iterate through the binary digits. In each iteration, we extract the rightmost digit (remainder) using the modulo operator (%
) and divide the binary number by 10 to remove the rightmost digit. - We calculate the decimal value of the extracted digit by multiplying it by 2 raised to the power of
i
and add it todecimalNumber
. - We increment
i
to move to the next position in the binary number. - Finally, we return the
decimalNumber
.
In the main
function, we take input from the user as a binary number, call the binaryToDecimal
function to perform the conversion, and then display the decimal equivalent.
Compile and run the program, and it will convert a binary number to its decimal representation.