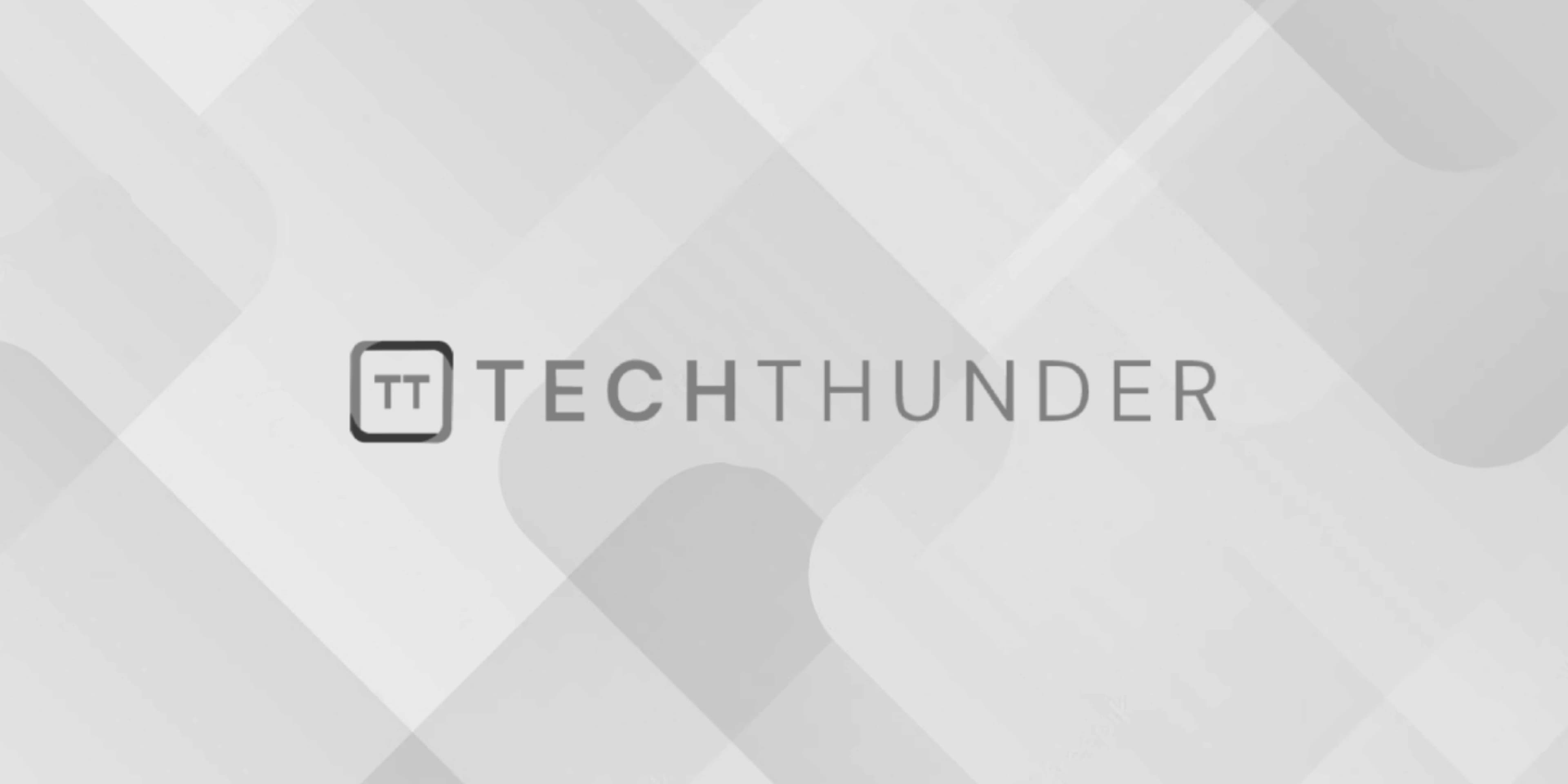
220 views
Literals in C
The literals are constant values that appear directly in your source code. They represent fixed values and data, such as integers, floating-point numbers, characters, and strings. Literals are used to initialize variables, specify constant values in expressions, and provide input or output data in your C program. Here are some common types of literals in C:
- Integer Literals:
- Integer literals represent whole numbers.
- Examples:
42
,-123
,0
,255
.
- Floating-Point Literals:
- Floating-point literals represent real numbers with decimal points.
- Examples:
3.14159
,-0.01
,1.0
.
- Character Literals:
- Character literals represent individual characters enclosed in single quotes.
- Examples:
'A'
,'7'
,'%'
.
- String Literals:
- String literals represent sequences of characters enclosed in double quotes.
- Example:
"Hello, World!"
.
- Boolean Literals:
- C does not have native boolean literals like some other languages. Instead, integers are often used to represent boolean values:
0
typically represents “false.”- Non-zero values (often
1
) represent “true.”
- Escape Sequences in String and Character Literals:
- You can use escape sequences within string and character literals to represent special characters. For example:
"\n"
represents a newline character."\t"
represents a tab character."\""
represents a double quote within a string.'\''
represents a single quote within a character literal.
- Octal and Hexadecimal Notation:
- Integer literals can be written in octal notation by prefixing them with
0
, such as075
. - Integer literals can also be written in hexadecimal notation by prefixing them with
0x
or0X
, such as0x1A
.
- Suffixes for Integer and Floating-Point Literals:
- You can use suffixes to specify the data type of integer and floating-point literals. For example:
42L
represents a long integer.3.14f
represents a float.2.5e3
represents a double in scientific notation.
Here are some examples of literals in C code:
C
int num = 42; // Integer literal
float pi = 3.14159; // Floating-point literal
char letter = 'A'; // Character literal
char name[] = "John"; // String literal
int isTrue = 1; // Boolean-like (1 represents true)
In C, literals play a crucial role in initializing variables, specifying constants, and providing data in your program. They are used extensively in C code to represent various types of data values.