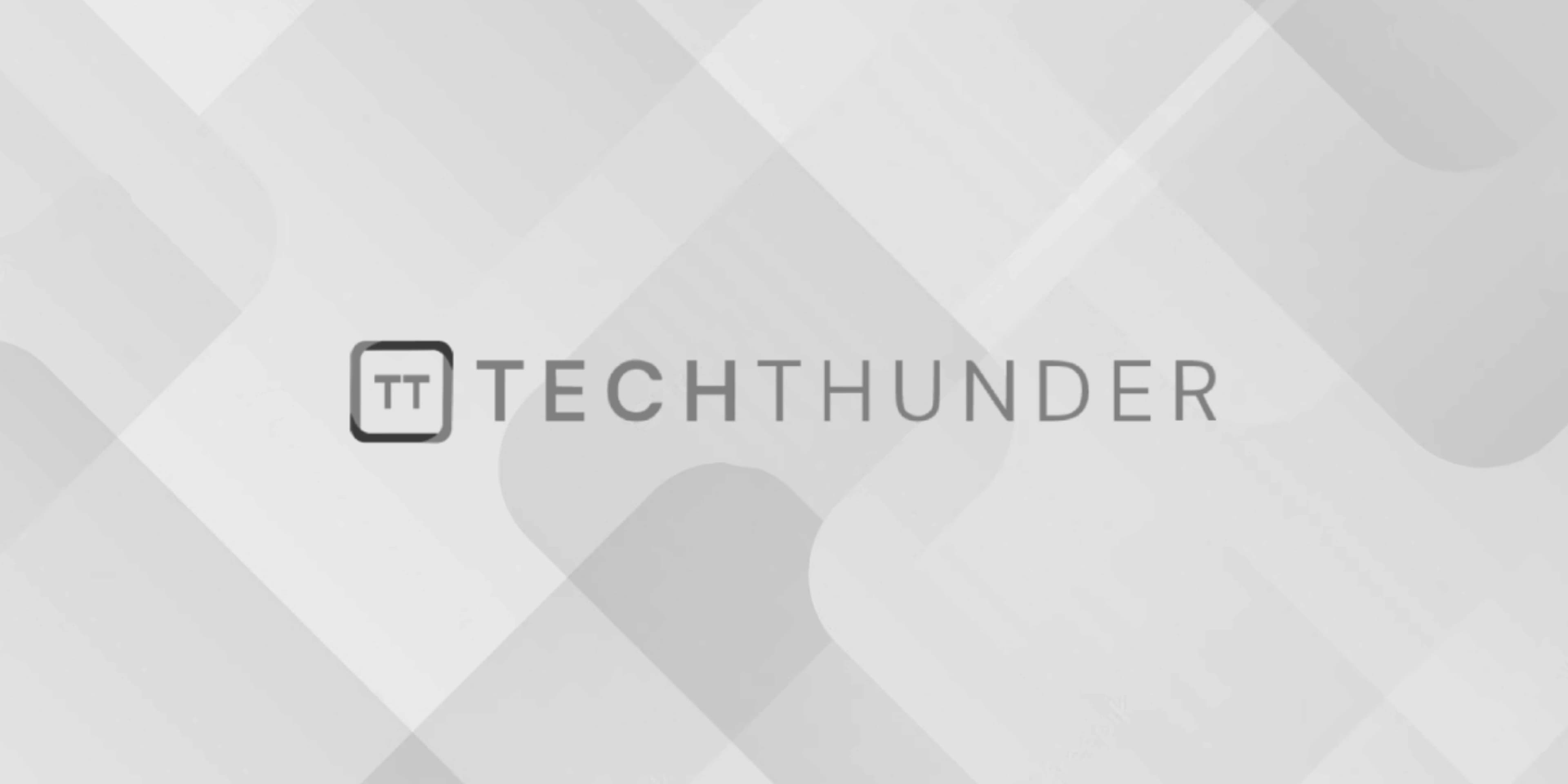
175 views
C #ifdef
The #ifdef
directive is used for conditional compilation. It checks whether a specific macro is defined, and if the macro is defined, it includes a block of code for compilation. If the macro is not defined, the code within the #ifdef
block is excluded from compilation.
Here’s the basic syntax of the #ifdef
directive:
C
#ifdef macro_name
// Code to compile if macro_name is defined
#endif
macro_name
is the name of the macro that you want to check for definition.
Here’s an example of how the #ifdef
directive is used:
C
#include <stdio.h>
#define DEBUG // Define DEBUG macro
int main() {
#ifdef DEBUG
printf("Debug mode is enabled.\n");
#else
printf("Debug mode is disabled.\n");
#endif
return 0;
}
In this example:
- The
DEBUG
macro is defined using#define
. This macro serves as a switch to enable or disable debugging code. - Inside the program, the
#ifdef
directive checks whether theDEBUG
macro is defined. Since it is defined, the code within the#ifdef
block is included in compilation. - As a result, “Debug mode is enabled.” is printed when the program is executed.
You can use the #ifdef
directive to conditionally include or exclude code based on the presence or absence of specific macros. This is commonly used for enabling or disabling debugging code, selecting different code paths for different platforms, or configuring feature flags in your C programs.