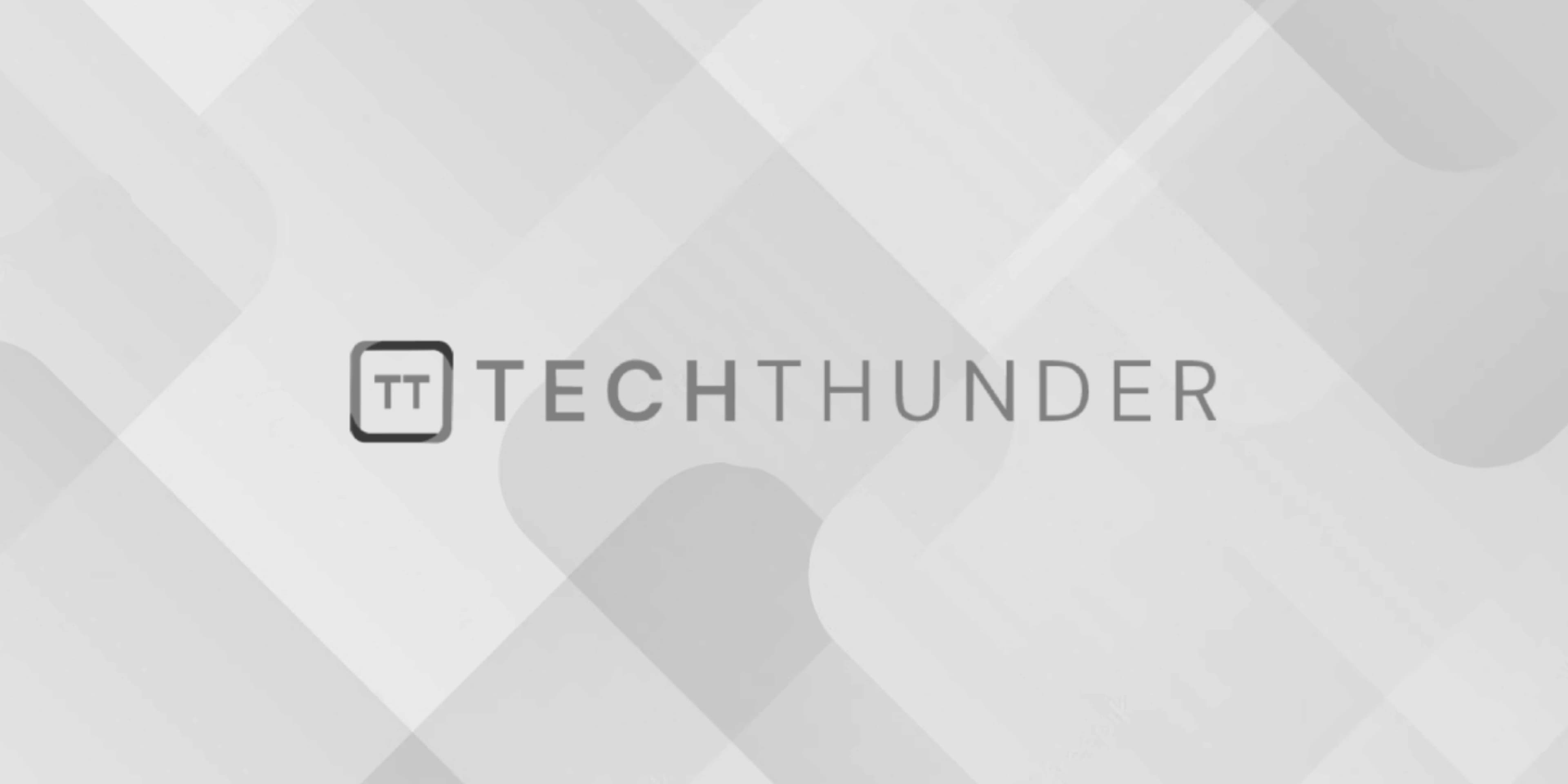
Execution of printf with ++ Operators in C
The behavior of the printf
function with ++
operators can be a bit tricky because it depends on whether the ++
operator is used as a postfix operator or a prefix operator, and it also depends on the order of evaluation in the expression. Let’s explore both scenarios:
1. Postfix ++
Operator:
When the ++
operator is used as a postfix operator (e.g., x++
), the current value of the variable is used in the expression, and then the variable is incremented. Here’s an example:
#include <stdio.h>
int main() {
int x = 5;
printf("x: %d\n", x); // Prints "x: 5"
printf("x++: %d\n", x++); // Prints "x++: 5"
printf("x: %d\n", x); // Prints "x: 6"
return 0;
}
In this example, x++
returns the current value of x
, which is 5, and then increments x
to 6.
2. Prefix ++
Operator:
When the ++
operator is used as a prefix operator (e.g., ++x
), the variable is incremented first, and then its value is used in the expression. Here’s an example:
#include <stdio.h>
int main() {
int x = 5;
printf("x: %d\n", x); // Prints "x: 5"
printf("++x: %d\n", ++x); // Prints "++x: 6"
printf("x: %d\n", x); // Prints "x: 6"
return 0;
}
In this example, ++x
increments the value of x
to 6, and then the incremented value is used in the printf
statement.
So, whether printf
is executed with ++
operators as postfix or prefix depends on how the ++
operator is used in your code. If it’s used as a postfix operator, the current value is used in the printf
. If it’s used as a prefix operator, the updated value after the increment is used. The order of evaluation in expressions also plays a role in determining the behavior.