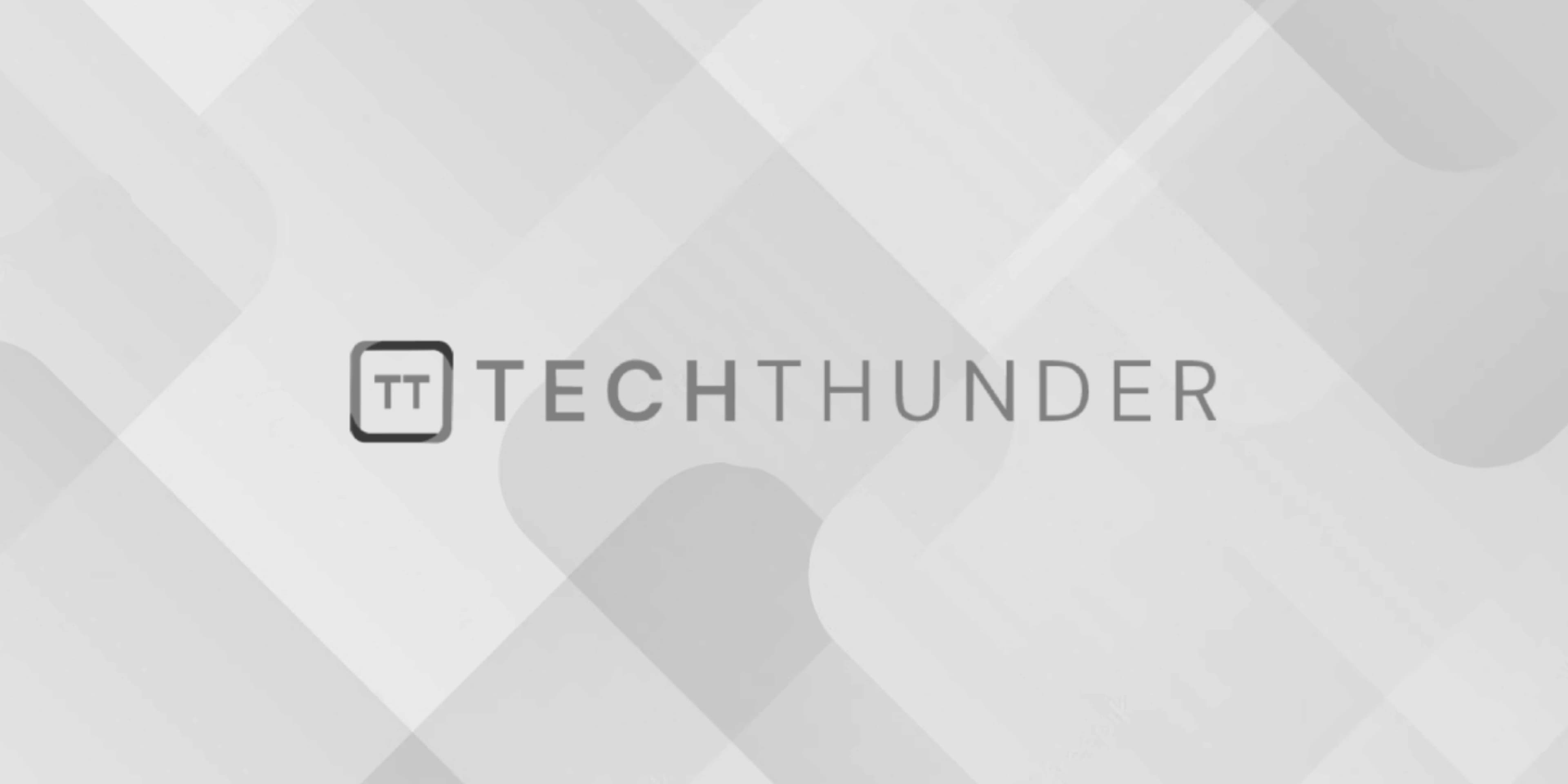
C rewind()
The rewind()
function is used to reset the file position indicator of a file stream back to the beginning of the file. It is typically used with files that have been opened using the fopen()
function and accessed using functions like fread()
and fwrite()
.
Here’s the syntax of rewind()
:
void rewind(FILE *stream);
stream
: A pointer to aFILE
object representing the file for which you want to reset the file position indicator.
The rewind()
function sets the file position indicator to the beginning of the file associated with the given file stream. After calling rewind()
, you can start reading or writing the file from the beginning.
Here’s an example of how to use rewind()
:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Read data from the file
char buffer[100];
fgets(buffer, sizeof(buffer), file);
printf("Data from the file: %s", buffer);
// Rewind the file to the beginning
rewind(file);
// Read data from the beginning again
fgets(buffer, sizeof(buffer), file);
printf("Data from the beginning: %s", buffer);
fclose(file);
return 0;
}
In this example, we open a file called “example.txt” in read mode, read some data from it, and then use rewind()
to reset the file position indicator to the beginning of the file. After rewinding, we read data from the beginning of the file again.
The rewind()
function is useful when you need to re-read or re-process the contents of a file from the beginning without closing and reopening the file.