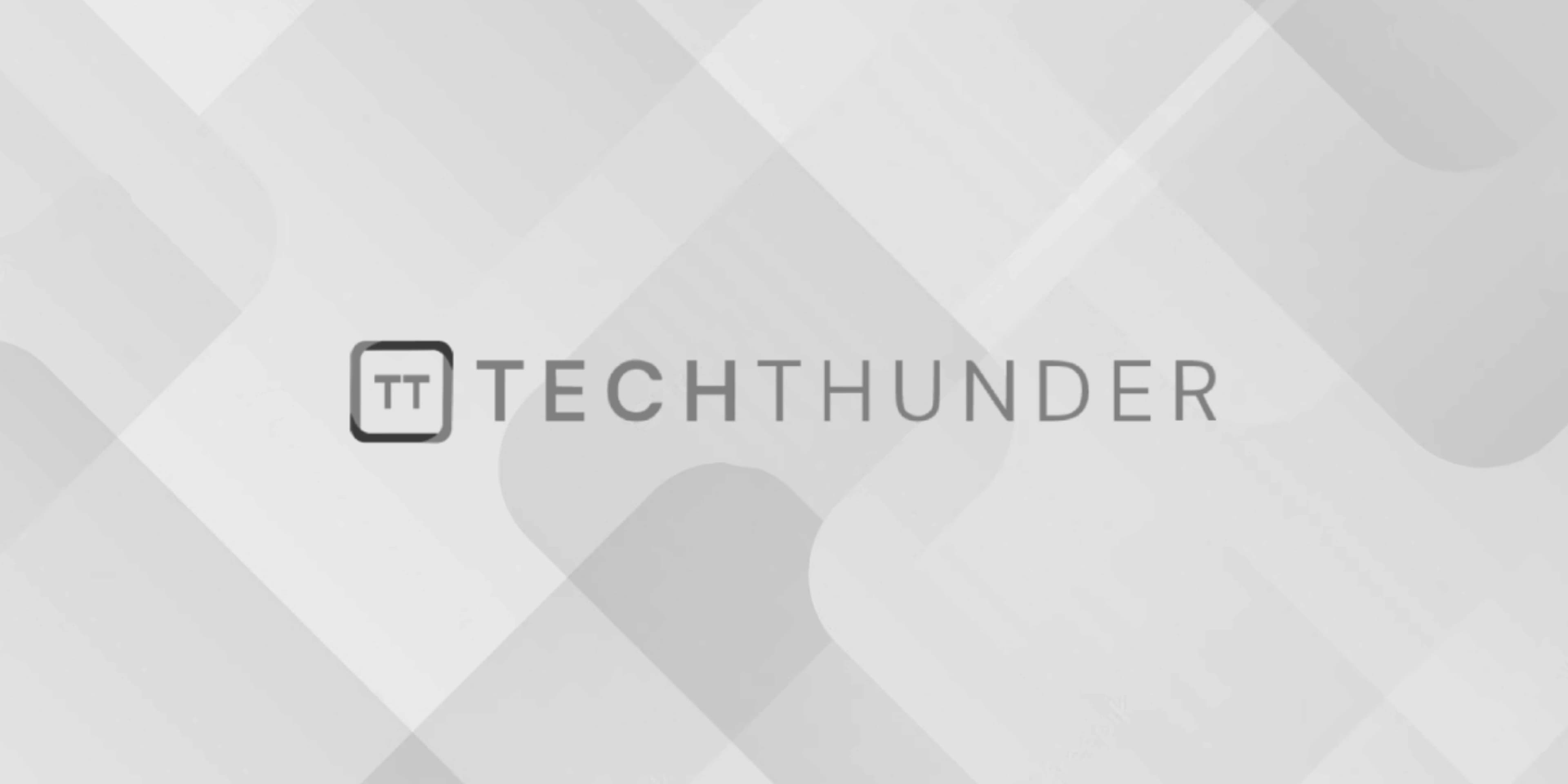
166 views
Octal to Hexadecimal in C
To convert an octal number to hexadecimal in C, you can first convert the octal number to decimal and then convert the decimal number to hexadecimal. Here’s a C program that demonstrates this conversion:
C
#include <stdio.h>
#include <stdlib.h>
int main() {
unsigned long octalNum;
// Input an octal number from the user
printf("Enter an octal number: ");
scanf("%lo", &octalNum);
// Convert octal to decimal
unsigned long decimalNum = 0, base = 1;
while (octalNum > 0) {
int digit = octalNum % 10;
decimalNum += digit * base;
base *= 8; // Octal is base-8
octalNum /= 10;
}
// Convert decimal to hexadecimal
char hex[20]; // Assuming enough space for the hexadecimal representation
int index = 0;
while (decimalNum > 0) {
int remainder = decimalNum % 16;
if (remainder < 10) {
hex[index++] = remainder + '0';
} else {
hex[index++] = remainder - 10 + 'A';
}
decimalNum /= 16;
}
hex[index] = '\0'; // Null-terminate the hexadecimal string
// Reverse the hexadecimal string
int start = 0, end = index - 1;
while (start < end) {
char temp = hex[start];
hex[start] = hex[end];
hex[end] = temp;
start++;
end--;
}
printf("Hexadecimal equivalent: 0x%s\n", hex);
return 0;
}
In this program:
- We first input an octal number from the user using
%lo
format specifier and store it in theoctalNum
variable. - We then convert the octal number to a decimal number by iterating through each digit of the octal number, multiplying it by the appropriate power of 8, and adding it to the
decimalNum
. - Next, we convert the decimal number to hexadecimal. We repeatedly divide the decimal number by 16, keeping track of remainders, and building the hexadecimal string character by character.
- The resulting hexadecimal string is reversed because the remainders were calculated from right to left, but the hexadecimal representation is written from left to right.
- Finally, we print the hexadecimal equivalent.
Compile and run the program, and you can enter an octal number to see its hexadecimal equivalent.