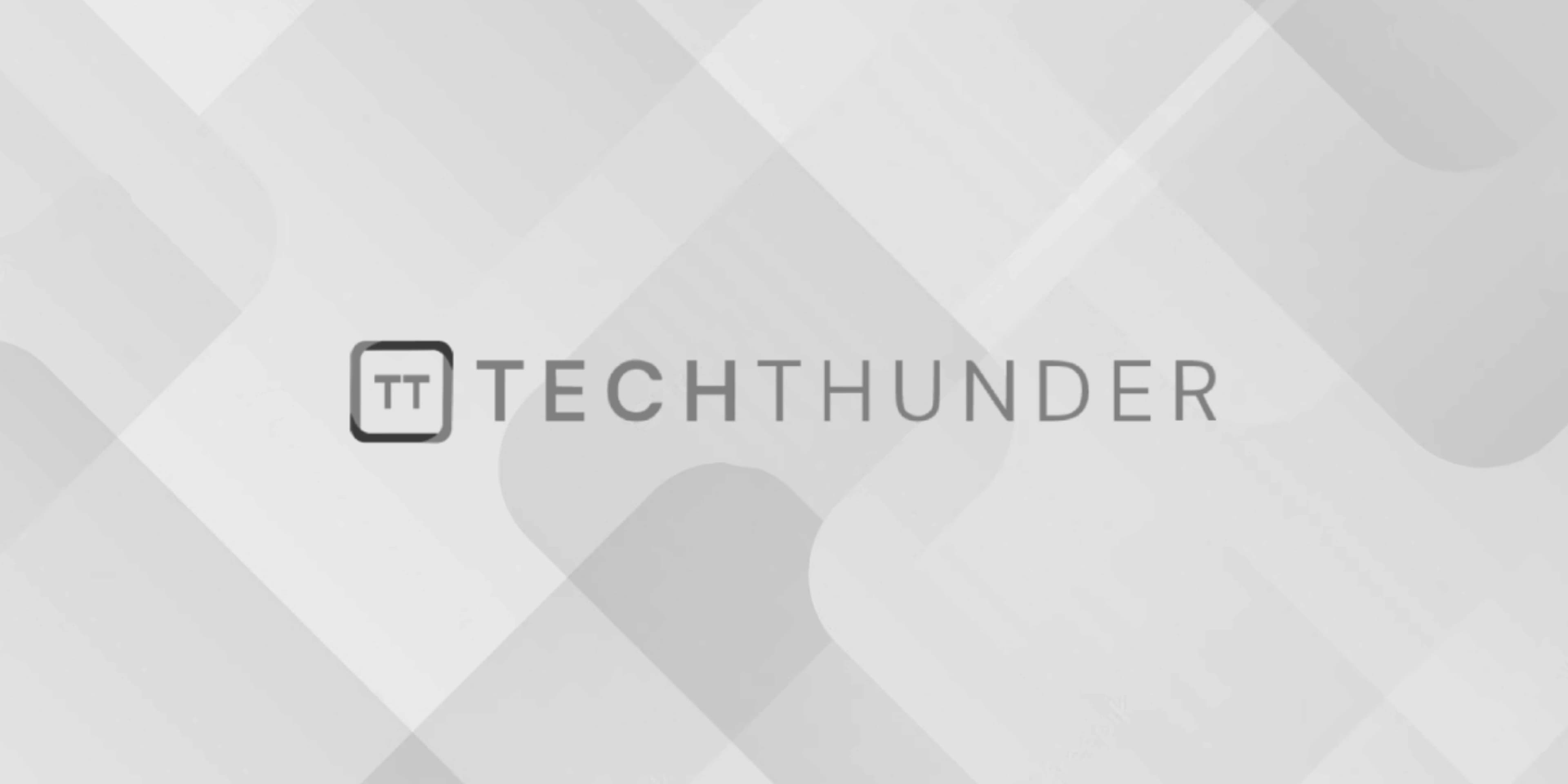
111 views
What is function in C
The function is a self-contained block of code that performs a specific task or set of tasks. Functions are one of the fundamental building blocks of C programs and are used to break down complex tasks into smaller, more manageable pieces. They allow you to organize code, make it more modular, and promote code reusability.
Here are some key points about functions in C:
- Function Declaration and Definition:
- To use a function in C, you first declare it. The declaration typically includes the function’s name, return type, and a list of parameters (if any). For example:
C
c int add(int a, int b); // Function declaration
- After declaring a function, you define its implementation, which contains the actual code that performs the task:
C
c int add(int a, int b) {
return a + b;
}
- Function Call:
- To use a function, you call it by its name and provide arguments (if required) based on the function’s parameter list. For example:
C
c int result = add(5, 3); // Function call
- Return Value:
- Functions can return a value to the caller using the
return
statement. The return type specified in the function declaration indicates the type of value that the function should return. - If a function does not return a value, its return type is specified as
void
.
- Parameters:
- Functions can accept parameters (also called arguments) that are used as inputs in the function’s computation. Parameters are specified in the function declaration and definition.
- Parameters allow you to pass data to functions and make them more flexible and reusable.
- Function Prototypes:
- In larger programs, it’s common to separate the declaration of functions from their implementation. This is done using function prototypes (declarations without the actual code).
- Function prototypes provide information about the function’s name, return type, and parameters, allowing you to use functions before they are defined.
- Standard Library Functions:
- C provides a standard library that includes a wide range of built-in functions for various tasks, such as input/output, string manipulation, math operations, and more. These functions can be used in your C programs without the need for additional declarations or definitions.
Here’s a simple example of a C function that adds two integers:
C
#include <stdio.h>
// Function declaration (prototype)
int add(int a, int b);
int main() {
int num1 = 5;
int num2 = 3;
// Function call
int result = add(num1, num2);
printf("Result: %d\n", result);
return 0;
}
// Function definition
int add(int a, int b) {
return a + b;
}
In this example, the add
function is declared with a prototype, defined with an implementation, and then called from the main
function to add two integers. Functions like this one allow you to encapsulate logic and make your code more organized and readable.