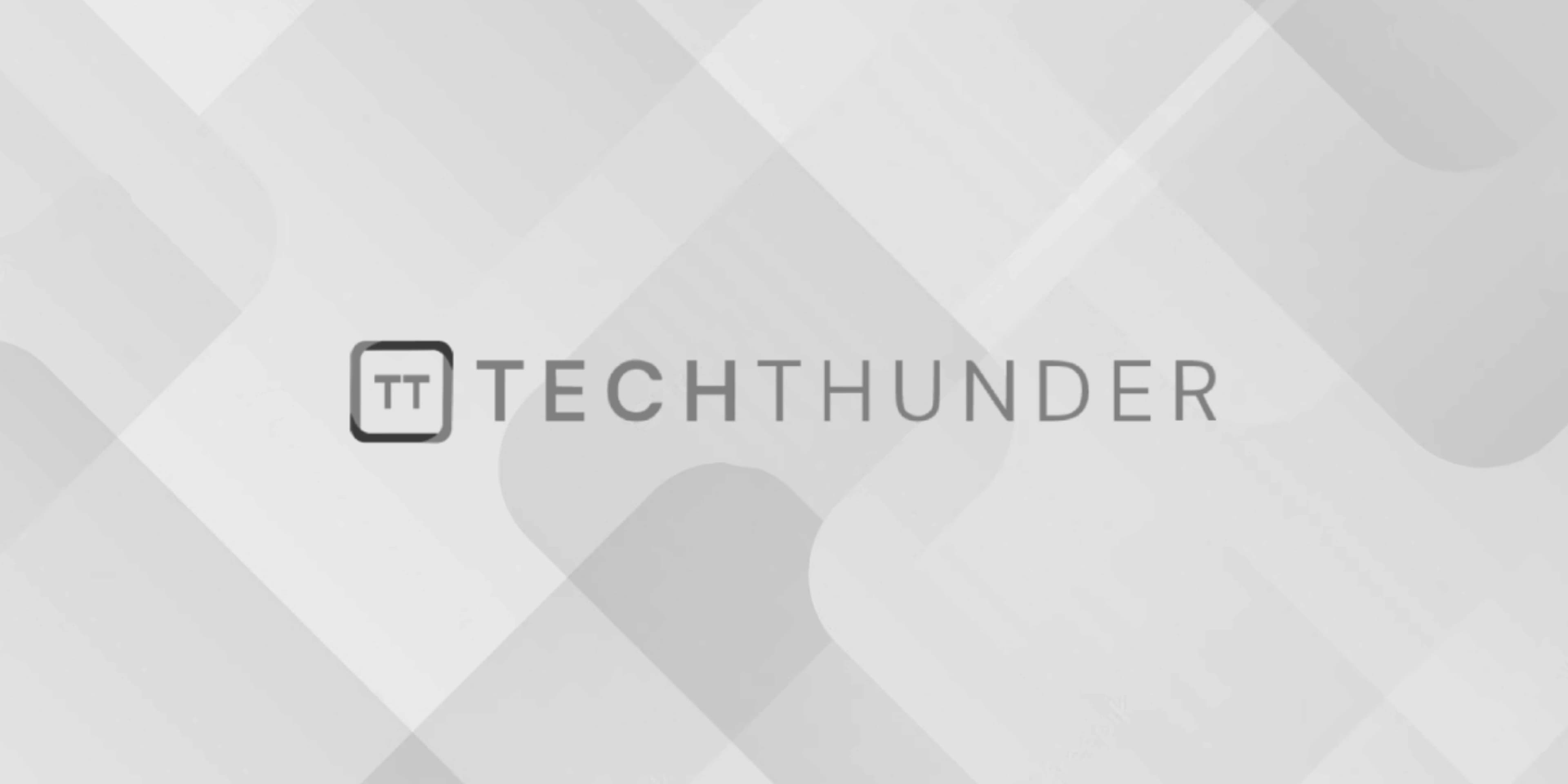
C Structure
The structure is a user-defined data type that allows you to group together variables of different data types under a single name. Structures are used to represent records, entities, or objects with multiple attributes or properties. Each attribute within a structure is called a member or field. Structures are especially useful when you need to organize and work with complex data in your program.
Here’s the basic syntax for defining a structure in C:
struct StructureName {
data_type member1_name;
data_type member2_name;
// Additional members...
};
StructureName
is the name of the structure.data_type
specifies the data type of each member.member1_name
,member2_name
, etc., are the names of the structure’s members.
Here’s an example of defining and using a structure:
#include <stdio.h>
// Define a structure named 'Person'
struct Person {
char name[50];
int age;
float height;
};
int main() {
// Declare a variable of type 'struct Person'
struct Person person1;
// Assign values to the structure members
strcpy(person1.name, "John");
person1.age = 30;
person1.height = 175.5;
// Access and print the structure members
printf("Name: %s\n", person1.name);
printf("Age: %d\n", person1.age);
printf("Height: %.1f\n", person1.height);
return 0;
}
In this example, we define a struct Person
with three members: name
, age
, and height
. We then declare a variable of type struct Person
named person1
and assign values to its members using dot notation. Finally, we access and print the members of the structure.
Structures are commonly used to represent complex data entities in C programs, such as employees, students, products, and more. They provide a way to group related data together for better organization and ease of access. Additionally, structures are often used in conjunction with arrays or linked lists to manage collections of data records.