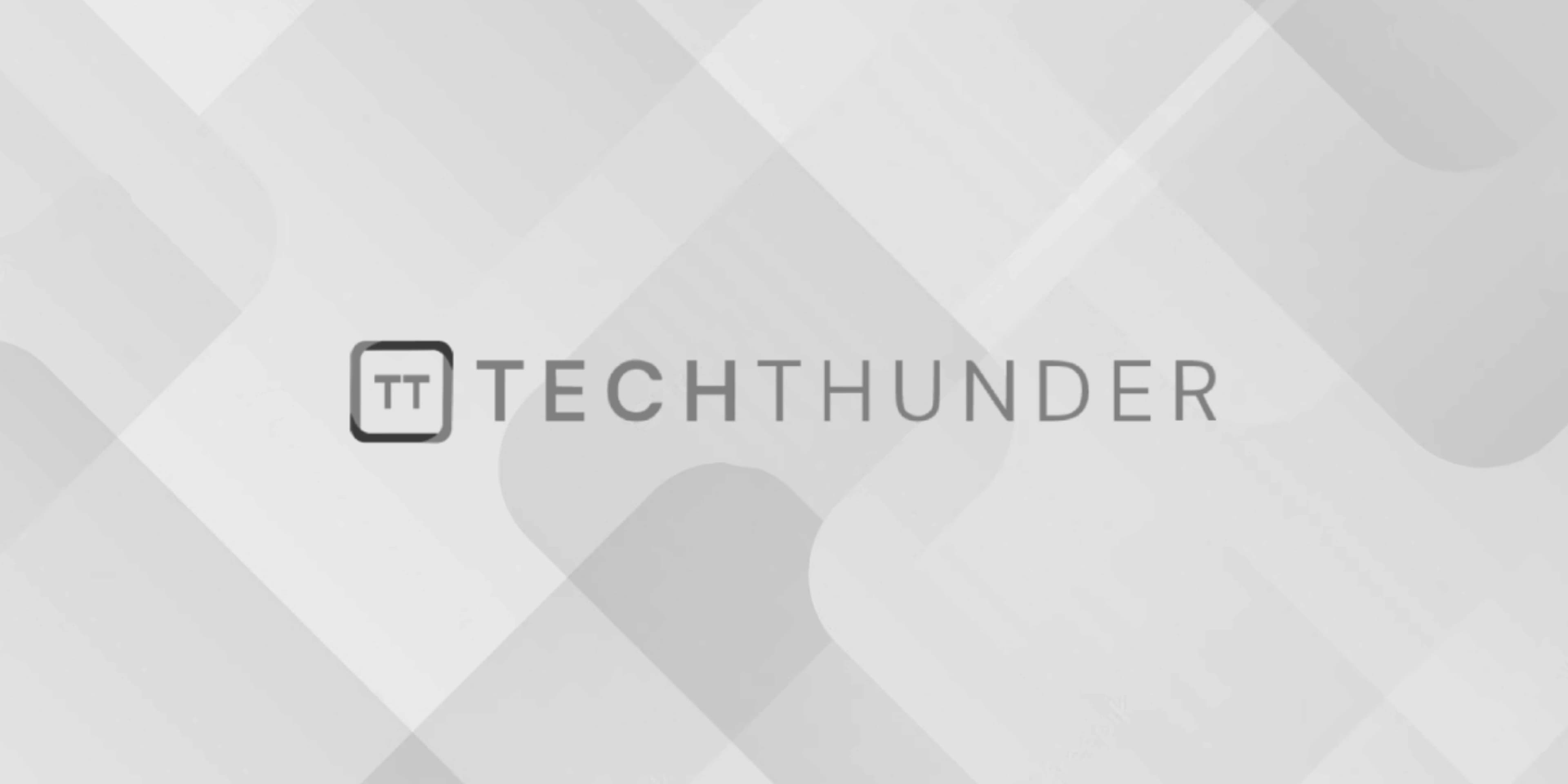
C switch
The switch
statement is a control flow statement that allows you to select one of many possible code blocks to execute based on the value of an expression. It provides an alternative to using multiple if-else if
statements when you need to make a decision among several options.
The basic syntax of the switch
statement is as follows:
switch (expression) {
case constant1:
// Code to execute if expression equals constant1
break; // Optional; used to exit the switch block
case constant2:
// Code to execute if expression equals constant2
break;
// Additional case labels as needed
default:
// Code to execute if expression does not match any case
}
Here’s how the switch
statement works:
- The
expression
is evaluated, and its value is compared to the constants in thecase
labels. - If a match is found, the code block associated with that
case
label is executed. - The
break
statement is used to exit theswitch
block after executing the code for a matchedcase
. Ifbreak
is not used, execution will continue into subsequentcase
blocks until abreak
is encountered or theswitch
block ends. - The
default
case is optional and is executed when none of thecase
labels match the value of the expression. It serves as the “default” action when no specific case is met.
Here’s an example that uses a switch
statement to determine the day of the week based on a numeric value:
#include <stdio.h>
int main() {
int day = 3; // Assuming 3 represents Wednesday
switch (day) {
case 1:
printf("Monday\n");
break;
case 2:
printf("Tuesday\n");
break;
case 3:
printf("Wednesday\n");
break;
case 4:
printf("Thursday\n");
break;
case 5:
printf("Friday\n");
break;
case 6:
printf("Saturday\n");
break;
case 7:
printf("Sunday\n");
break;
default:
printf("Invalid day\n");
}
return 0;
}
In this example, the value of day
is compared to each case
label, and the corresponding day of the week is printed. If day
does not match any of the case
labels, the default
case is executed, indicating that it’s an invalid day.
A few key points to keep in mind when using switch
:
- The
expression
inside theswitch
should evaluate to an integer or character type (e.g.,int
,char
,enum
). - Each
case
label must be a constant expression (e.g., integer constants, character literals, or enumeration constants). - Use
break
to exit theswitch
block when the desiredcase
is found. - The
default
case is optional but provides a fallback option for unmatched values. - You can have multiple
case
labels for the same block of code (fall-through behavior) if multiple cases should execute the same code.
The switch
statement is a powerful control flow construct for handling multiple conditional branches efficiently and is commonly used for menu-driven programs and state machines.