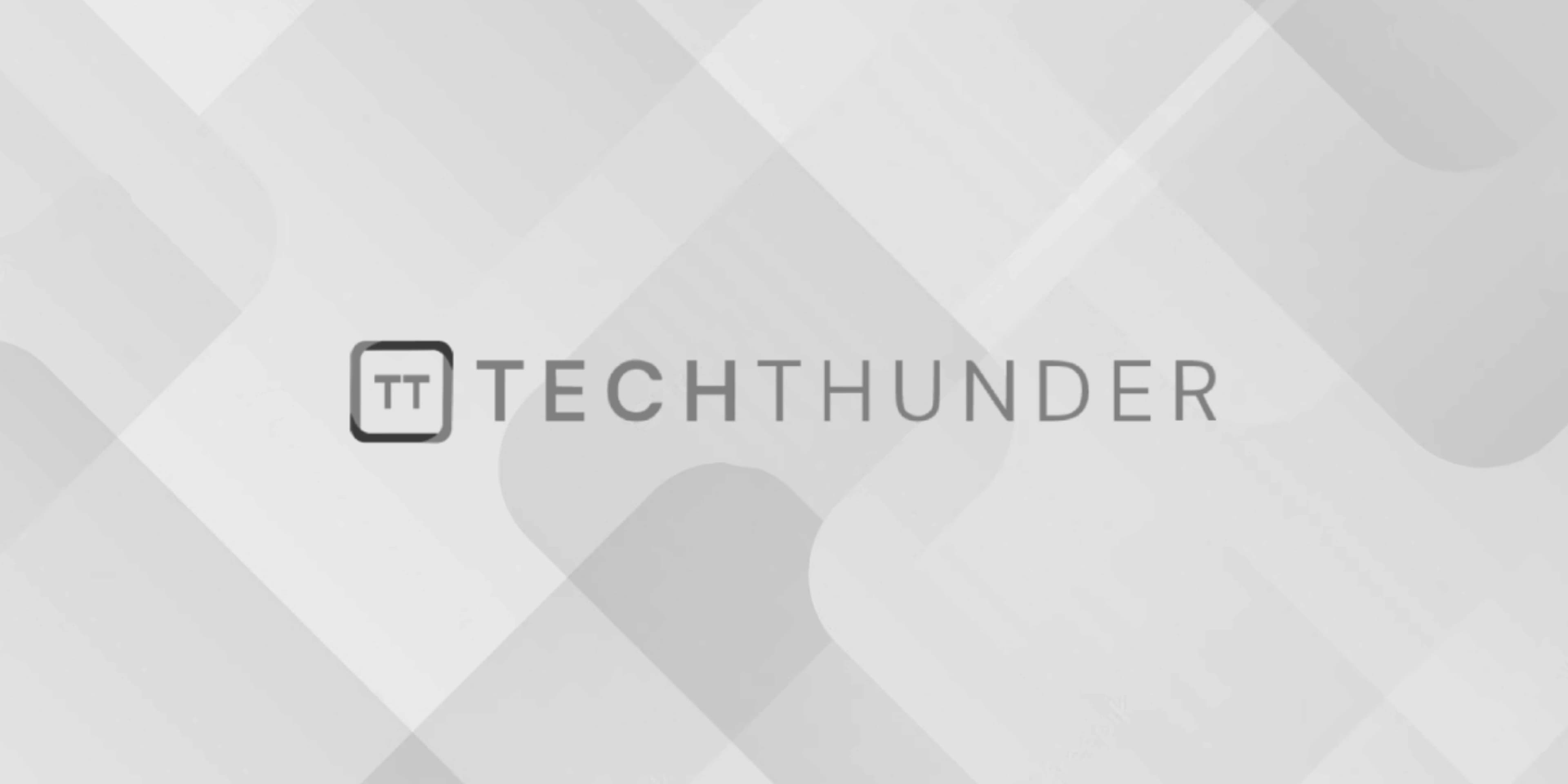
243 views
C Program For Printing Inverted Pyramid
To print an inverted pyramid in C, you can use nested loops. Here’s a simple C program to print an inverted pyramid pattern of asterisks (*):
C
#include <stdio.h>
int main() {
int rows, i, j;
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = rows; i >= 1; i--) {
// Print spaces
for (j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print asterisks
for (j = 1; j <= 2 * i - 1; j++) {
printf("*");
}
printf("\n");
}
return 0;
}
In this program:
- The user is prompted to enter the number of rows for the inverted pyramid.
- Two nested loops are used: one for controlling the rows (from
rows
down to 1), and another for controlling the spaces and asterisks within each row. - The inner loop prints spaces for each row (the number of spaces decreases as you go down the rows).
- The second inner loop prints asterisks, and the number of asterisks increases as you go down the rows.
- After printing the spaces and asterisks for each row, a newline character is added to move to the next line.
Compile and run this program, and it will display the inverted pyramid pattern based on the number of rows entered by the user.