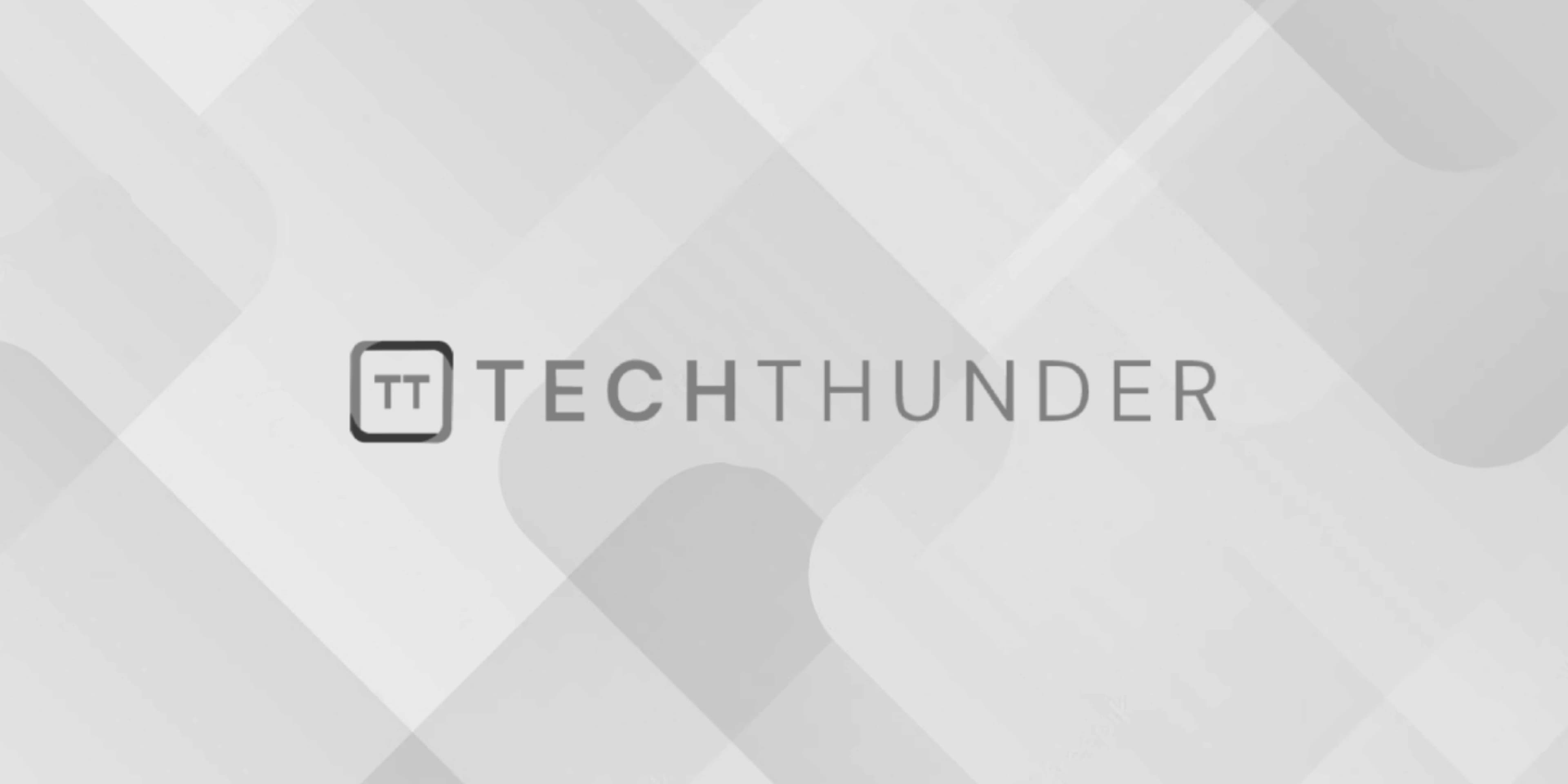
192 views
C Pointers
Pointers in C are variables that store memory addresses. They are a fundamental feature of the C programming language and are used for a variety of purposes, including dynamic memory allocation, data manipulation, and function call optimization. Here’s an overview of pointers in C:
- Declaration and Initialization:
- To declare a pointer variable, you use an asterisk
*
before the variable name. For example:int *ptr;
declares a pointer to an integer. - Pointers should be initialized with the address of a valid variable or memory location. For example:
int x = 10; int *ptr = &x;
initializesptr
with the address ofx
.
- Dereferencing:
- To access the value pointed to by a pointer, you use the dereference operator
*
. For example:int y = *ptr;
assigns the value ofx
toy
by dereferencingptr
.
- Address-of Operator:
- To obtain the memory address of a variable, you use the address-of operator
&
. For example:int *ptr = &x;
stores the address ofx
inptr
.
- Pointer Arithmetic:
- You can perform arithmetic operations on pointers, such as addition and subtraction. This is often used when working with arrays.
- Incrementing a pointer moves it to the next memory location of its type. For example:
ptr++;
movesptr
to the next integer in memory.
- Null Pointers:
- A null pointer is a pointer that doesn’t point to any valid memory location. It’s represented by the constant
NULL
or0
and is often used to indicate that a pointer is not currently pointing to anything.
- Void Pointers:
- A
void
pointer (void *
) is a special type of pointer that can point to objects of any data type. It’s commonly used in functions that need to accept or return pointers of unknown types.
- Pointer to Functions:
- You can declare pointers to functions, allowing you to call functions dynamically at runtime or pass functions as arguments to other functions.
- Dynamic Memory Allocation:
- Pointers are frequently used to allocate and deallocate memory dynamically using functions like
malloc()
,calloc()
, andfree()
. These functions return pointers to dynamically allocated memory.
- Pointers and Arrays:
- In C, arrays are closely related to pointers. When you use the name of an array without an index, it is treated as a pointer to its first element. For example,
int arr[5]; int *ptr = arr;
makesptr
point to the first element ofarr
.
- Pointer Safety:
- Pointers provide powerful capabilities but require careful use to avoid common issues like null pointer dereferences, buffer overflows, and memory leaks.
Pointers are a fundamental concept in C, and mastering their use is essential for becoming proficient in the language. They enable low-level memory manipulation and fine-grained control over data, making C a powerful language for system programming and embedded systems. However, improper use of pointers can lead to difficult-to-debug errors, so it’s important to use them with care.