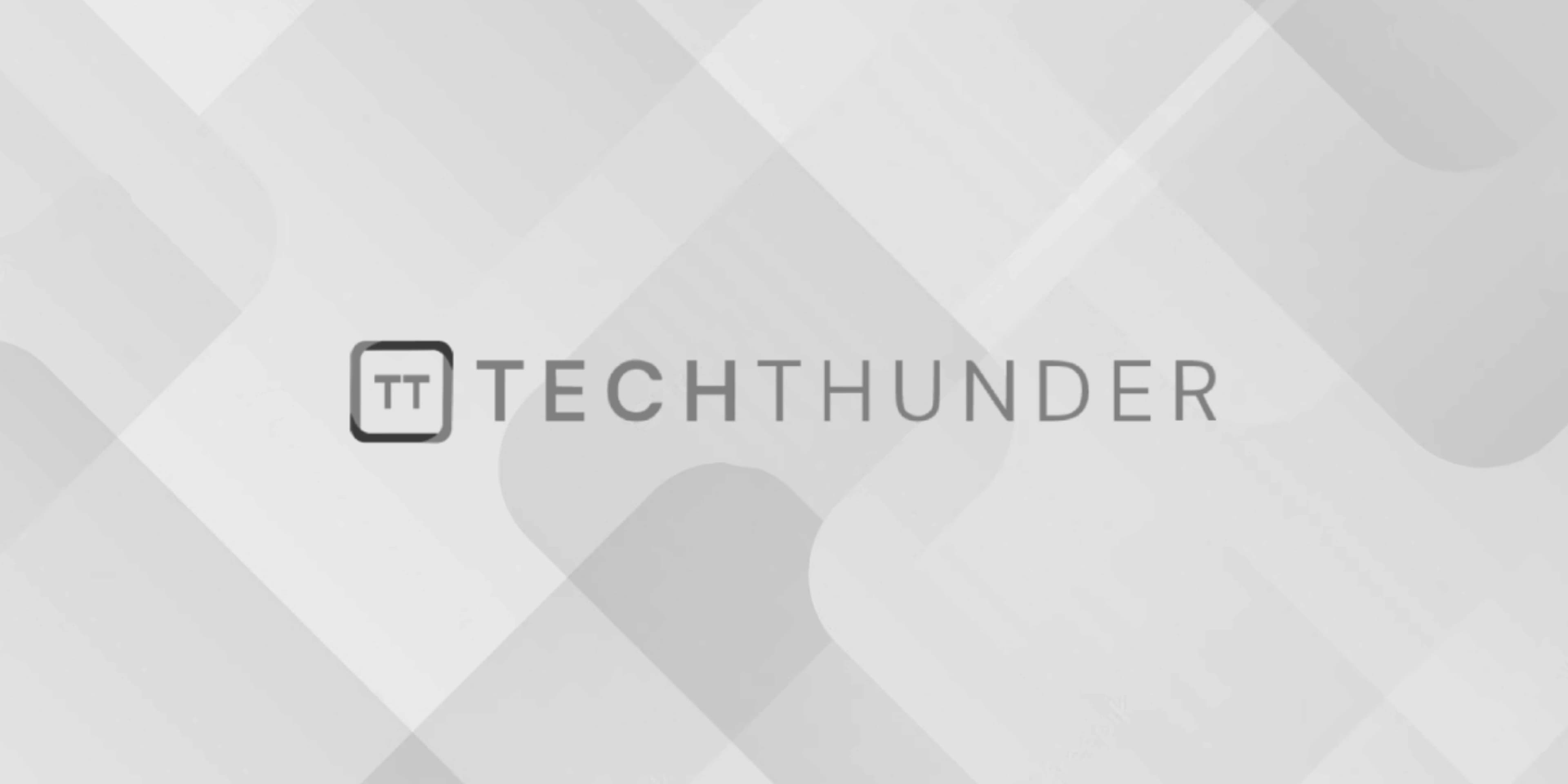
315 views
Matrix Multiplication in C
Matrix multiplication in C involves multiplying two matrices to produce a resulting matrix. To multiply two matrices, the number of columns in the first matrix must be equal to the number of rows in the second matrix. The resulting matrix will have dimensions equal to the number of rows in the first matrix and the number of columns in the second matrix.
Here’s a C program that demonstrates matrix multiplication:
C
#include <stdio.h>
void multiplyMatrices(int A[][3], int B[][2], int result[][2], int row1, int col1, int row2, int col2) {
if (col1 != row2) {
printf("Matrix multiplication is not possible.\n");
return;
}
for (int i = 0; i < row1; i++) {
for (int j = 0; j < col2; j++) {
result[i][j] = 0;
for (int k = 0; k < col1; k++) {
result[i][j] += A[i][k] * B[k][j];
}
}
}
}
void displayMatrix(int matrix[][2], int rows, int cols) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d\t", matrix[i][j]);
}
printf("\n");
}
}
int main() {
int row1 = 2, col1 = 3;
int row2 = 3, col2 = 2;
int matrixA[row1][3] = {{1, 2, 3}, {4, 5, 6}};
int matrixB[3][2] = {{7, 8}, {9, 10}, {11, 12}};
int result[row1][2];
multiplyMatrices(matrixA, matrixB, result, row1, col1, row2, col2);
printf("Matrix A:\n");
displayMatrix(matrixA, row1, col1);
printf("\nMatrix B:\n");
displayMatrix(matrixB, row2, col2);
printf("\nResulting Matrix:\n");
displayMatrix(result, row1, col2);
return 0;
}
In this program:
- The
multiplyMatrices
function takes two matricesA
andB
, along with their dimensions, and computes their product, storing the result in theresult
matrix. - The
displayMatrix
function is used to display the contents of a matrix. - In the
main
function, we define two matrices (matrixA
andmatrixB
) and their dimensions. We then callmultiplyMatrices
to perform the matrix multiplication and display the input matrices and the resulting matrix.
This program demonstrates matrix multiplication for matrices of different dimensions. Adjust the dimensions and matrix values as needed for your specific use case.