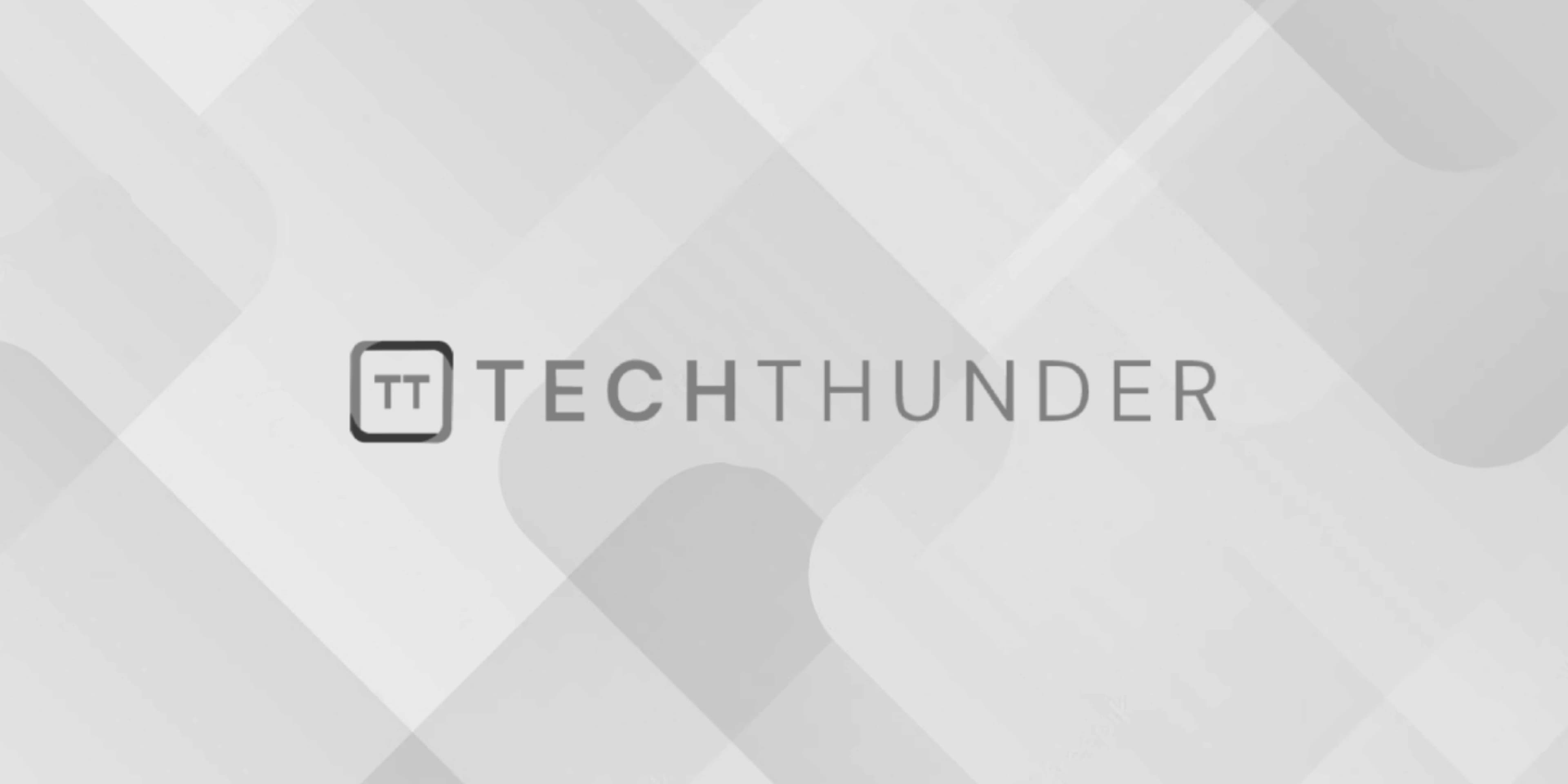
234 views
Enum in C
The enumeration, often referred to as an enum
, is a user-defined data type that consists of a set of named integer constants. Enumerations provide a way to define a list of named values, which makes the code more readable and maintainable.
Here’s how you can declare and use an enum in C:
C
#include <stdio.h>
// Declaration of an enumeration type named Color
enum Color {
RED, // 0
GREEN, // 1
BLUE // 2
};
int main() {
// Declaring variables of the enum type
enum Color myColor = GREEN;
enum Color anotherColor = BLUE;
// Using enum values
printf("My favorite color is %d\n", myColor); // Prints 1
printf("Another color is %d\n", anotherColor); // Prints 2
return 0;
}
In this example:
- We define an enumeration type called
Color
using theenum
keyword. - Inside the
enum Color
block, we list the possible values (constants):RED
,GREEN
, andBLUE
. By default,RED
is assigned the value 0,GREEN
is assigned 1, andBLUE
is assigned 2. You can specify explicit values if needed (e.g.,RED = 5
). - In the
main
function, we declare variables of theenum Color
type and assign them values. - We then print the values of these variables, which will display the corresponding integer values of the enum constants.
Enumerations are often used in C to define a set of related named constants, improving code readability and maintainability. They are especially useful when you want to work with a limited set of options or states in your program.