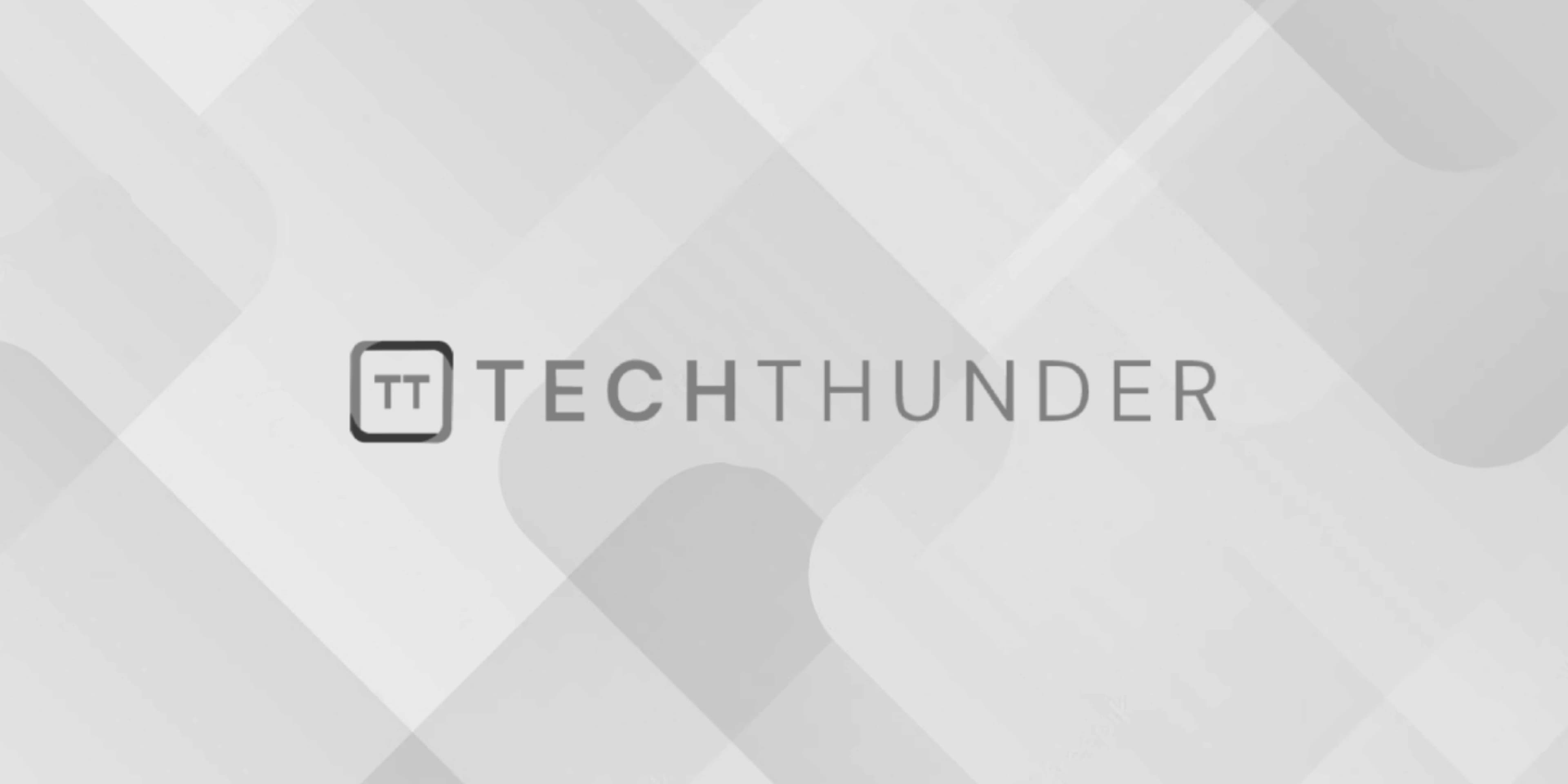
Restrict keyword in C
The restrict
keyword in C is a type qualifier that provides a hint to the compiler about the absence of aliasing between pointers. It’s used to optimize code by indicating that a particular pointer is the only one that will access a specific region of memory during its scope. The restrict
keyword can improve the performance of certain programs by allowing the compiler to make more aggressive optimizations.
The primary purpose of restrict
is to help the compiler generate more efficient code, especially in situations where it might otherwise need to reload data from memory multiple times or avoid certain optimizations due to pointer aliasing.
Here’s the basic syntax for using the restrict
keyword:
void some_function(int* restrict ptr1, int* restrict ptr2) {
// The compiler can assume that ptr1 and ptr2 do not alias each other
// and can perform optimizations accordingly.
}
In this example, ptr1
and ptr2
are declared as restrict
pointers. This means that within the scope of the some_function
function, the compiler can assume that ptr1
and ptr2
do not point to overlapping memory regions. As a result, it can perform optimizations that would otherwise be unsafe in the presence of aliasing.
It’s important to use the restrict
keyword with caution and only when you are absolutely certain that there is no aliasing between pointers. Violating the restrict keyword’s contract (i.e., having aliasing pointers) can lead to undefined behavior.
Keep in mind that the restrict
keyword is primarily an optimization hint, and its use may not always lead to significant performance improvements. Its effectiveness depends on the specific code, compiler, and optimization settings.
Here’s an example of using the restrict
keyword in a simple function:
#include <stdio.h>
void copy_data(int* restrict dest, const int* restrict src, size_t n) {
for (size_t i = 0; i < n; i++) {
dest[i] = src[i];
}
}
int main() {
int source[] = {1, 2, 3, 4, 5};
int destination[5];
copy_data(destination, source, 5);
for (size_t i = 0; i < 5; i++) {
printf("%d ", destination[i]);
}
printf("\n");
return 0;
}
In this example, the copy_data
function uses restrict
to indicate that dest
and src
pointers do not alias each other. This allows the compiler to optimize the memory copy operation more effectively.