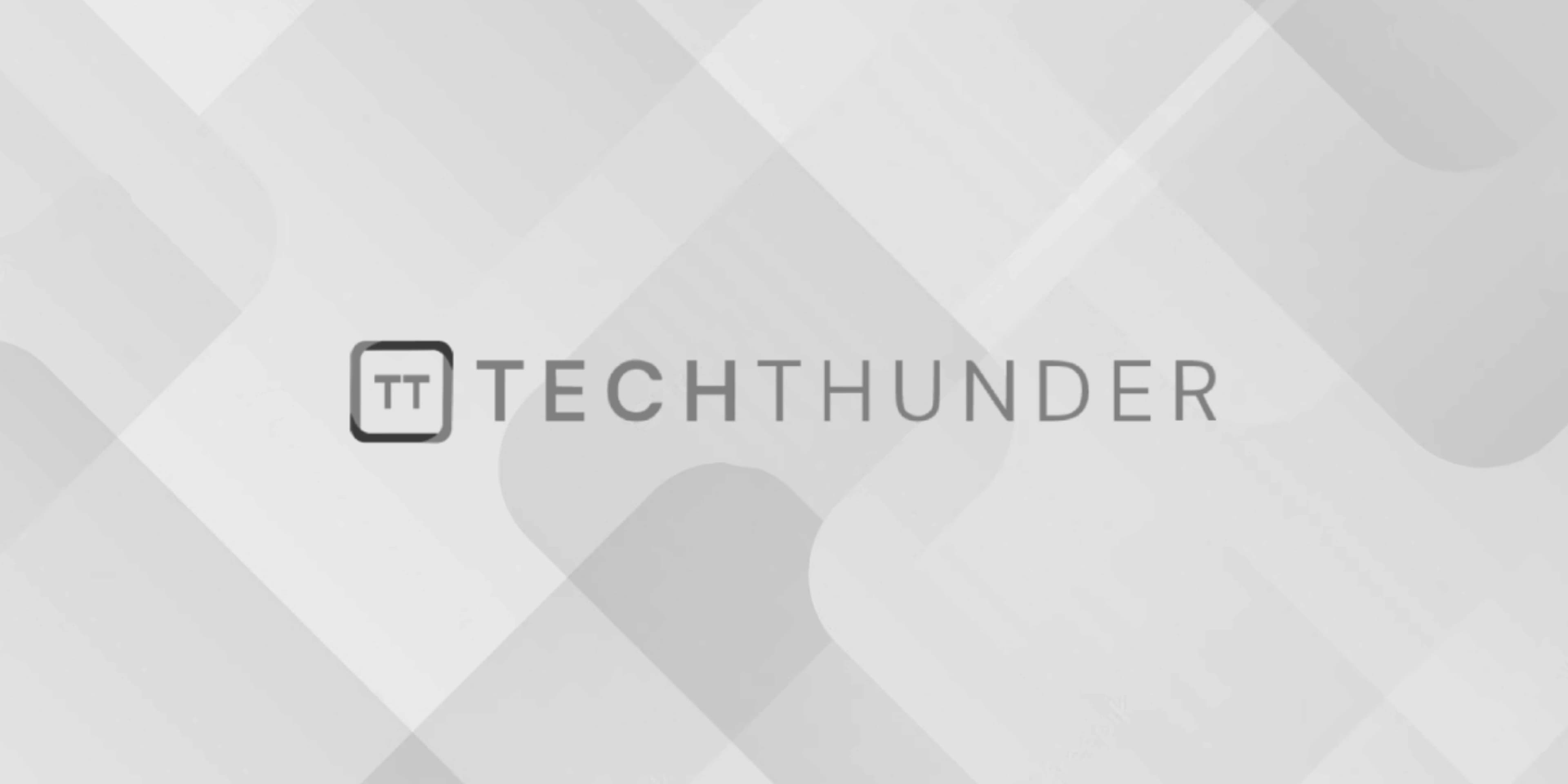
C #define
The #define
directive is used to create macros. Macros are symbolic names that represent a sequence of characters or values. These symbolic names can be used to replace text in your code. Macros are processed by the C preprocessor before the code is compiled, and they are often used to create constants or to define short, reusable code snippets.
Here’s the basic syntax of the #define
directive:
#define macro_name replacement_text
macro_name
is the name you want to give to the macro.replacement_text
is the text or value that the macro represents.
There are two common use cases for #define
:
- Creating Constants:
You can use#define
to create constants, which are values that don’t change during the program’s execution. For example:
#define PI 3.14159265359
After defining this macro, you can use PI
in your code, and the preprocessor will replace PI
with the specified value.
- Creating Code Macros:
Macros can be used to create short, reusable code snippets. For example:
#define SQUARE(x) ((x) * (x))
This macro defines a function-like macro SQUARE
that calculates the square of a number. You can use it in your code like this:
int result = SQUARE(5); // result will be 25
The preprocessor replaces SQUARE(5)
with ((5) * (5))
.
Here’s an example that demonstrates both constant and code macros:
#include <stdio.h>
#define PI 3.14159265359
#define SQUARE(x) ((x) * (x))
int main() {
double radius = 5.0;
double area = PI * SQUARE(radius);
printf("Area of a circle with radius %.2f is %.2f\n", radius, area);
return 0;
}
In this example:
- The
PI
constant is used to represent the mathematical constant π. - The
SQUARE
macro is used to calculate the square of theradius
.
The C preprocessor replaces PI
and SQUARE(radius)
with their respective values before the code is compiled, resulting in the calculation of the circle’s area.
Using #define
allows you to make your code more readable, maintainable, and flexible by defining meaningful names for constants and creating reusable code snippets.