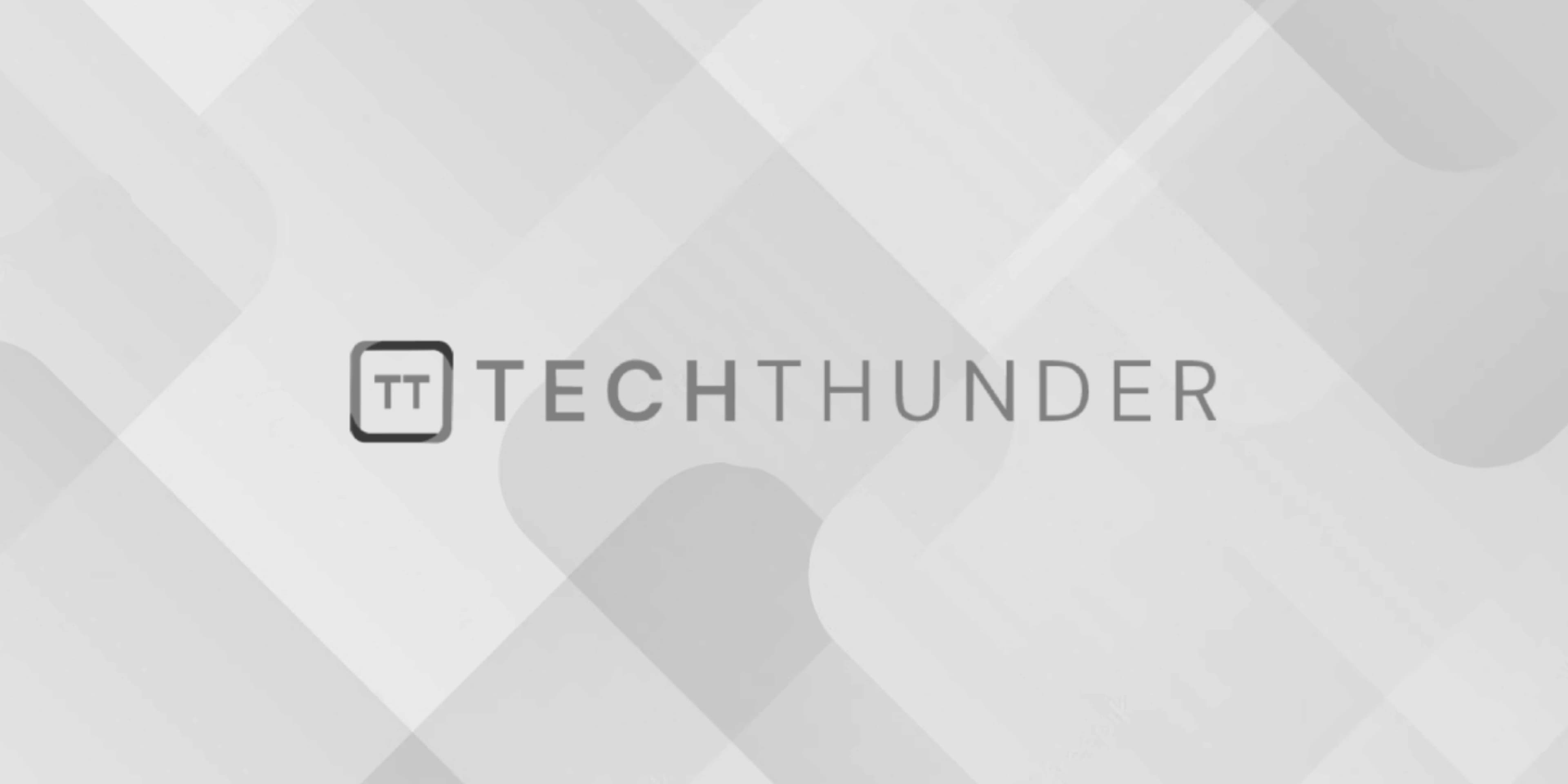
229 views
C Pointers Test
Testing pointers in C often involves tasks like allocating and deallocating memory dynamically, accessing data using pointers, and checking for null pointers. Here’s a simple example that demonstrates some basic pointer operations in C:
C
#include <stdio.h>
#include <stdlib.h>
int main() {
// Declare a pointer to an integer
int *ptr;
// Allocate memory for an integer using malloc
ptr = (int *)malloc(sizeof(int));
if (ptr == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
// Assign a value to the integer through the pointer
*ptr = 42;
// Access and print the value using the pointer
printf("Value at the pointer: %d\n", *ptr);
// Deallocate the memory
free(ptr);
// Check if the pointer is null after deallocation
if (ptr == NULL) {
printf("Pointer is null after deallocation.\n");
} else {
printf("Pointer is not null after deallocation.\n");
}
return 0;
}
In this example, we do the following:
- Declare a pointer to an integer
int *ptr;
. - Allocate memory for an integer using
malloc()
and assign the address of the allocated memory to the pointerptr
. We check if the allocation was successful by testing whetherptr
isNULL
. - Assign a value (42) to the integer that
ptr
points to using the dereference operator*ptr
. - Access and print the value using the pointer
printf("Value at the pointer: %d\n", *ptr);
. - Deallocate the dynamically allocated memory using
free(ptr);
. - Check if the pointer is
NULL
after deallocation to ensure that it’s a good practice to set the pointer toNULL
after freeing the memory.
This example provides a basic illustration of using pointers, dynamic memory allocation, and proper memory management. When working with pointers, it’s crucial to handle memory allocation and deallocation carefully to avoid memory leaks and undefined behavior.