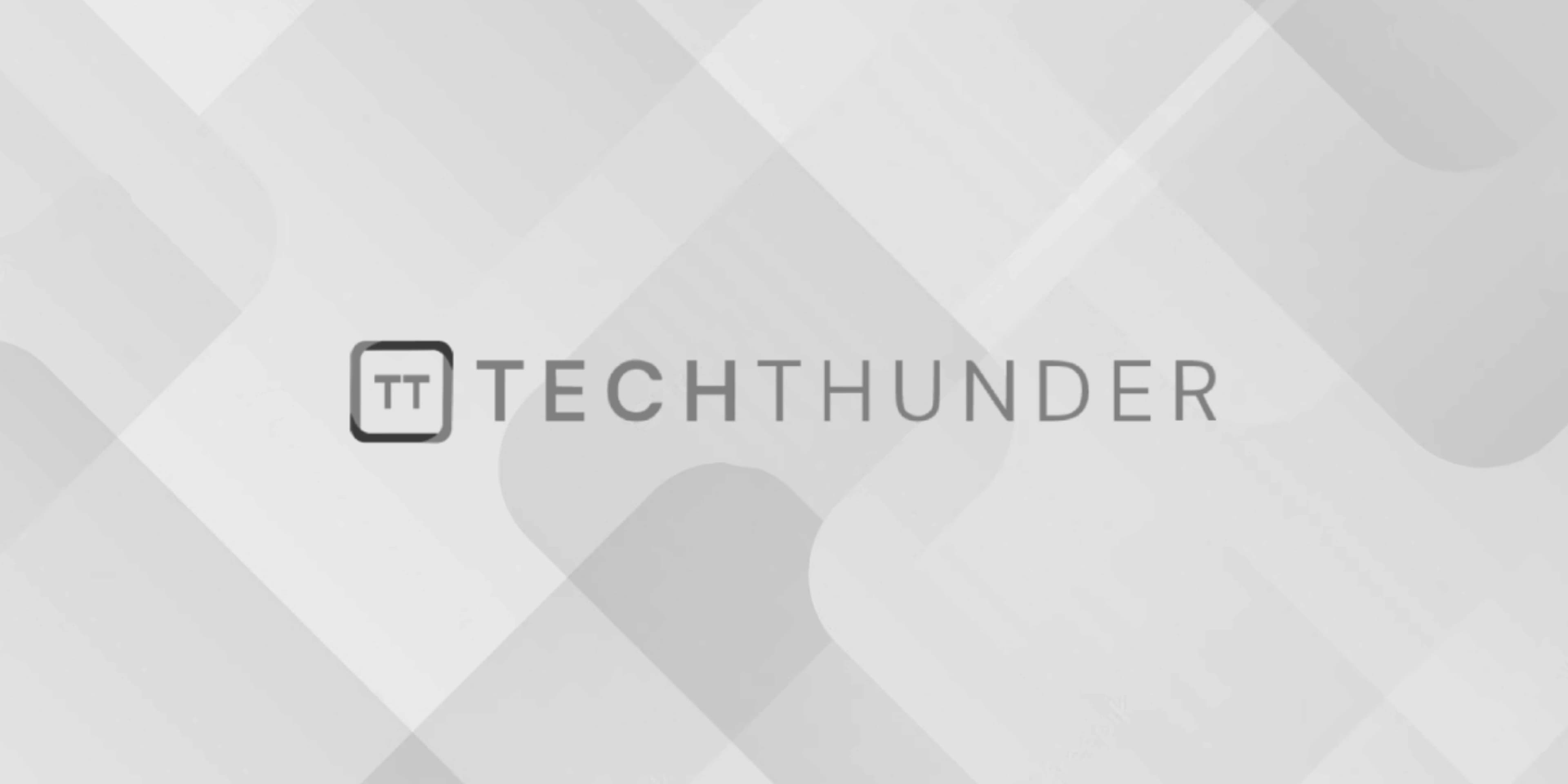
112 views
Department Management System in C
Creating a department management system in C can be a complex project, depending on the requirements and features you want to include. Below is a simplified console-based example of a department management system in C that focuses on managing a list of employees within a department. In this example, we’ll use an array to store employee data.
C
#include <stdio.h>
#include <string.h>
// Structure to represent an employee
struct Employee {
char name[50];
int employeeId;
char position[50];
double salary;
};
int main() {
struct Employee department[100]; // Array to store employee data
int numEmployees = 0; // Number of employees in the department
int choice;
do {
printf("\nDepartment Management System\n");
printf("1. Add Employee\n");
printf("2. List Employees\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
if (numEmployees < 100) {
struct Employee newEmployee;
printf("Enter employee name: ");
scanf("%s", newEmployee.name);
printf("Enter employee ID: ");
scanf("%d", &newEmployee.employeeId);
printf("Enter employee position: ");
scanf("%s", newEmployee.position);
printf("Enter employee salary: ");
scanf("%lf", &newEmployee.salary);
department[numEmployees] = newEmployee;
numEmployees++;
printf("Employee added successfully.\n");
} else {
printf("The department is full. Cannot add more employees.\n");
}
break;
case 2:
printf("List of Employees:\n");
for (int i = 0; i < numEmployees; i++) {
printf("Employee ID: %d, Name: %s, Position: %s, Salary: %.2lf\n",
department[i].employeeId, department[i].name, department[i].position, department[i].salary);
}
break;
case 3:
printf("Exiting the program.\n");
break;
default:
printf("Invalid choice. Please try again.\n");
}
} while (choice != 3);
return 0;
}
In this simplified department management system:
- We use a
struct Employee
to represent employee information, including their name, employee ID, position, and salary. - We create an array called
department
to store employee records. The array can hold up to 100 employees (you can adjust this limit as needed). - The program provides options to add new employees and list existing employees.
- The user can choose to exit the program when done.
Please note that this is a basic example. A real-world department management system would typically involve more features such as employee searching, updating, deleting, and possibly data persistence using files or a database.