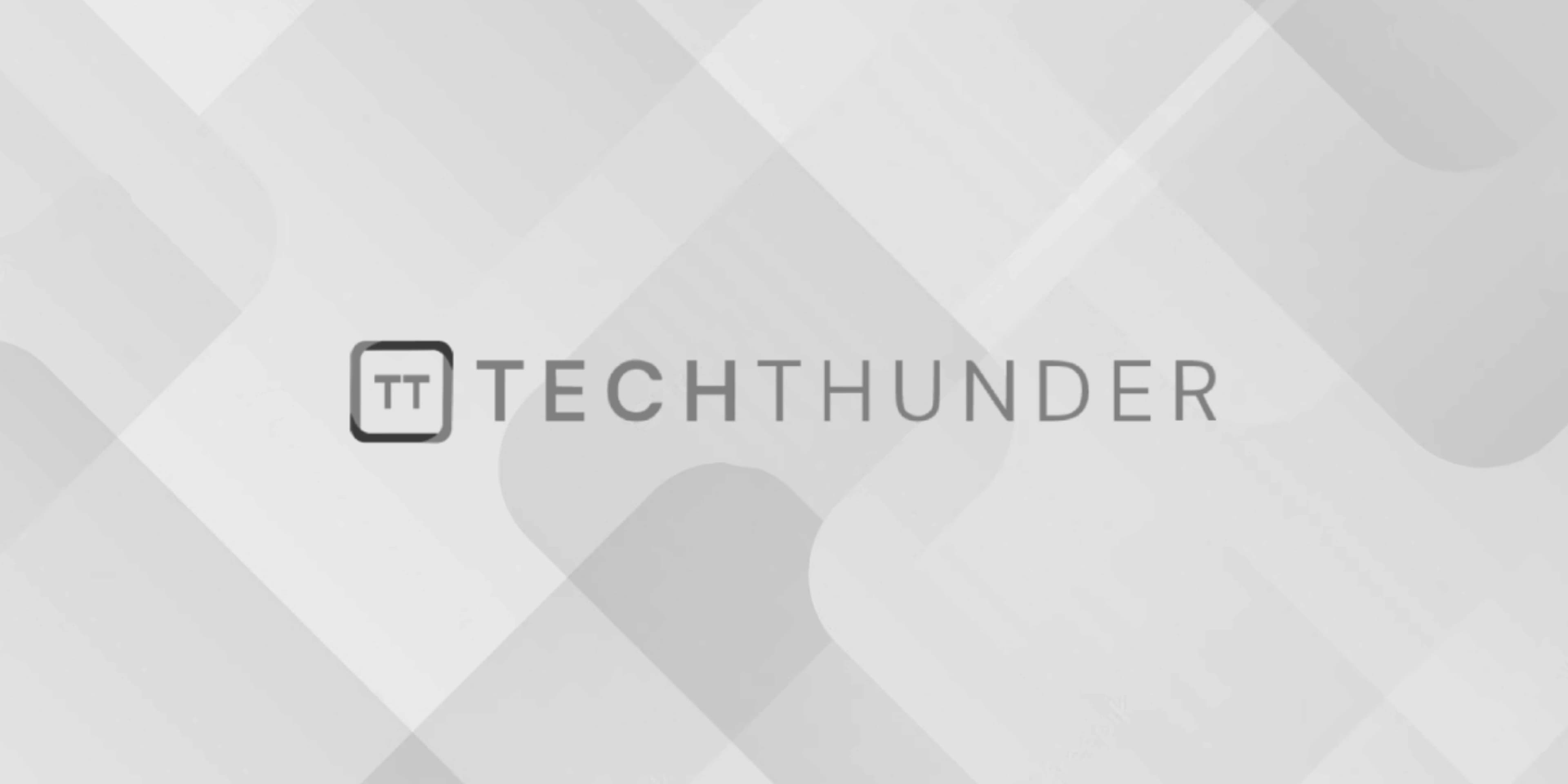
193 views
C Operators
The operators are symbols or keywords that are used to perform operations on data. Operators allow you to manipulate variables and values, make decisions, and perform various calculations in your C programs. C provides a wide range of operators, which can be categorized into the following main groups:
- Arithmetic Operators:
- Arithmetic operators perform mathematical operations on numeric operands.
- Examples:
+
(addition),-
(subtraction),*
(multiplication),/
(division),%
(modulus).
C
int a = 10, b = 5;
int sum = a + b; // Addition
int diff = a - b; // Subtraction
int product = a * b; // Multiplication
int quotient = a / b; // Division
int remainder = a % b; // Modulus
- Relational Operators:
- Relational operators compare two values and return a Boolean result (
true
orfalse
). - Examples:
==
(equal to),!=
(not equal to),<
(less than),>
(greater than),<=
(less than or equal to),>=
(greater than or equal to).
C
int x = 5, y = 10;
bool isEqual = (x == y); // Equal to
bool isNotEqual = (x != y); // Not equal to
bool isLess = (x < y); // Less than
bool isGreater = (x > y); // Greater than
bool isLessOrEqual = (x <= y); // Less than or equal to
bool isGreaterOrEqual = (x >= y); // Greater than or equal to
- Logical Operators:
- Logical operators perform logical operations on Boolean values.
- Examples:
&&
(logical AND),||
(logical OR),!
(logical NOT).
C
bool a = true, b = false;
bool andResult = (a && b); // Logical AND
bool orResult = (a || b); // Logical OR
bool notResult = !a; // Logical NOT
- Assignment Operators:
- Assignment operators assign a value to a variable.
- Examples:
=
(assignment),+=
(addition assignment),-=
(subtraction assignment),*=
(multiplication assignment),/=
(division assignment),%=
(modulus assignment).
C
int num = 10;
num += 5; // num is now 15
num -= 3; // num is now 12
num *= 2; // num is now 24
num /= 4; // num is now 6
num %= 2; // num is now 0
- Increment and Decrement Operators:
- Increment and decrement operators are used to increase or decrease the value of a variable by one.
- Examples:
++
(increment),--
(decrement).
C
int count = 5;
count++; // Increment count by 1 (count is now 6)
count--; // Decrement count by 1 (count is now 5 again)
- Conditional (Ternary) Operator:
- The conditional operator (
? :
) is a shorthand way of writing anif-else
statement. - Example:
condition ? value_if_true : value_if_false
C
int x = 10, y = 20;
int max = (x > y) ? x : y; // max will be assigned the larger of x and y
- Bitwise Operators:
- Bitwise operators perform operations at the bit level.
- Examples:
&
(bitwise AND),|
(bitwise OR),^
(bitwise XOR),~
(bitwise NOT),<<
(left shift),>>
(right shift).
C
unsigned int a = 5, b = 3;
unsigned int bitwiseAnd = a & b; // Bitwise AND
unsigned int bitwiseOr = a | b; // Bitwise OR
unsigned int bitwiseXor = a ^ b; // Bitwise XOR
unsigned int bitwiseNotA = ~a; // Bitwise NOT
unsigned int leftShift = a << 1; // Left shift by 1
unsigned int rightShift = a >> 1; // Right shift by 1
- Other Operators:
- C also includes other operators like the address-of operator
&
, the pointer dereference operator*
, the sizeof operatorsizeof
, and the comma operator,
, among others.
Operators in C are fundamental to performing operations and making decisions in your code. Understanding how to use them effectively is crucial for writing efficient and functional C programs.