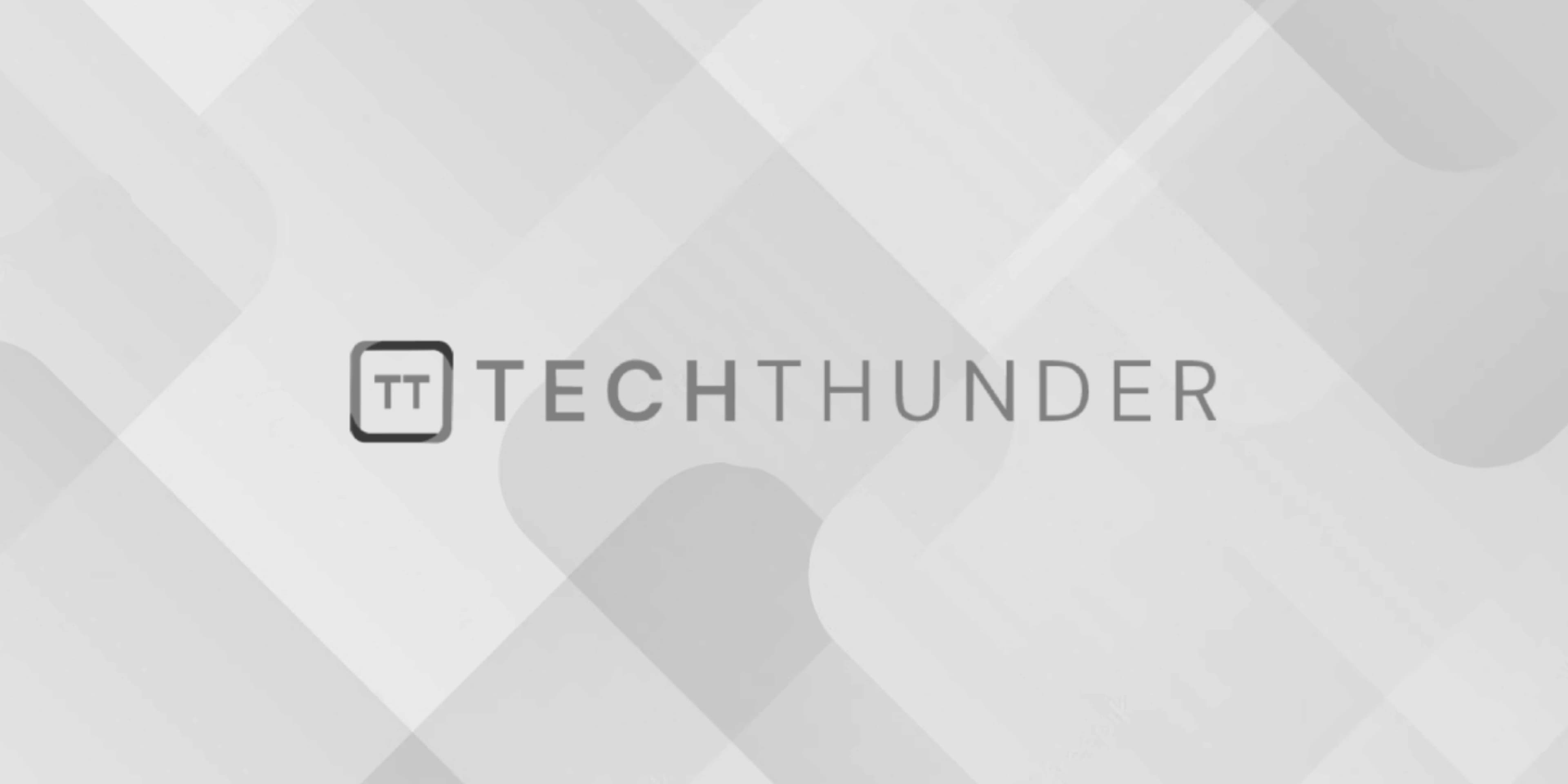
145 views
Hexadecimal to Decimal in C
You can convert a hexadecimal number to a decimal number in C using the scanf
function to input the hexadecimal value as a string and then using the strtol
function to perform the conversion. Here’s a C program that demonstrates this:
C
#include <stdio.h>
#include <stdlib.h>
int main() {
char hex[20]; // Assuming enough space for the hexadecimal representation
long decimalNum;
// Input a hexadecimal number as a string
printf("Enter a hexadecimal number: ");
scanf("%s", hex);
// Convert hexadecimal to decimal
decimalNum = strtol(hex, NULL, 16);
printf("Decimal equivalent: %ld\n", decimalNum);
return 0;
}
In this program:
- We declare a character array
hex
to store the input hexadecimal number as a string and along
variabledecimalNum
to store the decimal equivalent. - We use the
scanf
function to input the hexadecimal number as a string. - We then use the
strtol
function to convert the hexadecimal string to a decimal number. Thestrtol
function takes three arguments:
- The first argument is the string to convert (
hex
in this case). - The second argument is a pointer to a character pointer, which we set to
NULL
because we don’t need to track the end of the parsed string. - The third argument is the base of the conversion, which is 16 for hexadecimal.
- Finally, we print the decimal equivalent.
Compile and run the program, and it will allow you to enter a hexadecimal number, convert it to decimal, and display the result.