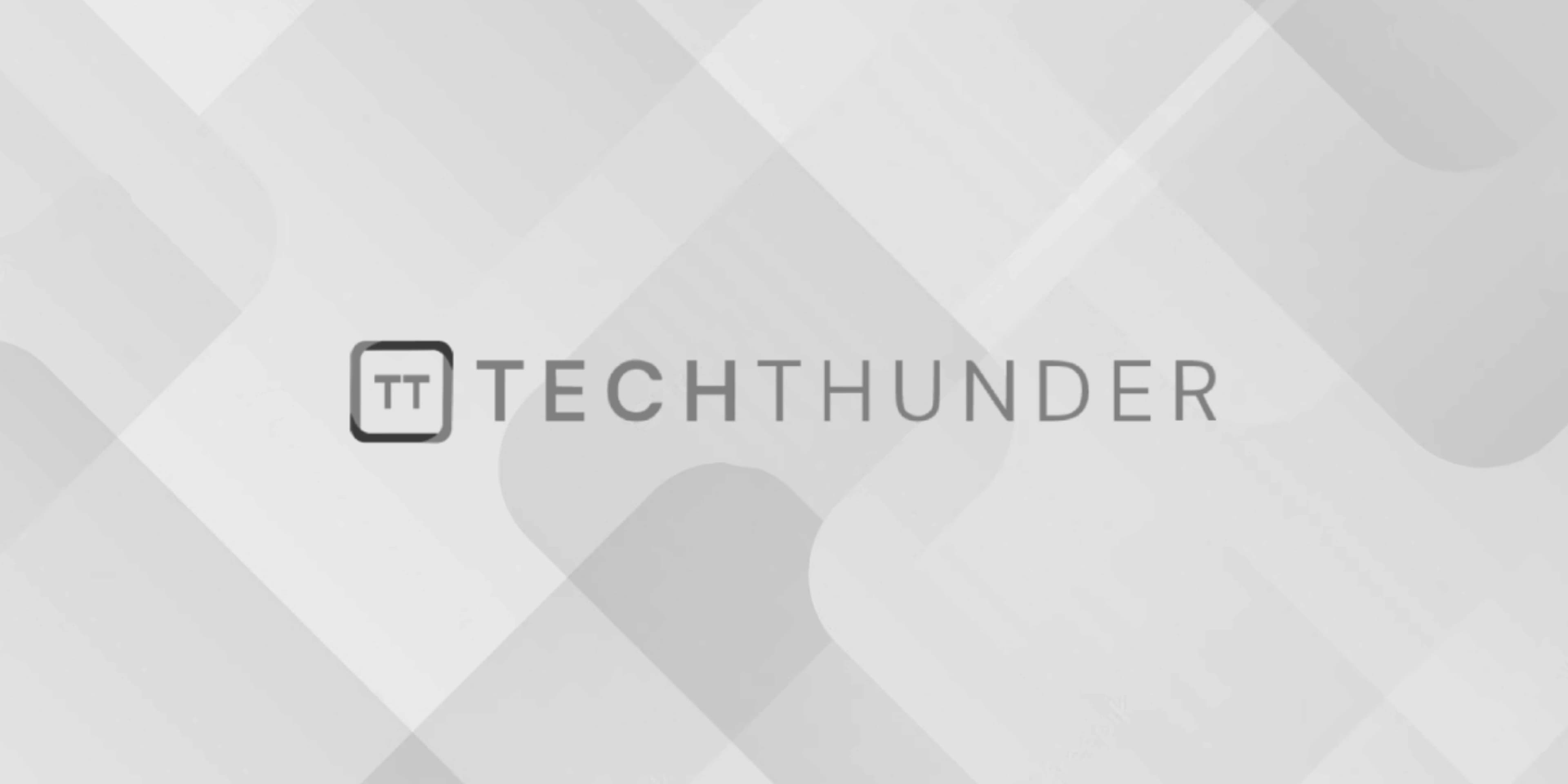
Deadlock Prevention using Bankers Algorithm in C
Deadlock prevention using the Banker’s algorithm is a way to manage resources in a multi-process or multi-threaded system to avoid deadlock situations. The Banker’s algorithm uses a set of data structures to track resource availability, process resource requests, and determine if a resource allocation should be granted to a process without causing a potential deadlock.
Here’s a simplified implementation of the Banker’s algorithm in C. Note that this example assumes a fixed number of processes and resources, which makes it easier to understand but less practical for real-world scenarios where these numbers may vary dynamically.
#include <stdio.h>
// Number of processes and resources
#define N 5
#define M 3
// Function to check if the requested resources can be granted to a process
int isSafe(int processes[N], int available[M], int max[N][M], int allocation[N][M]) {
int need[N][M];
int finish[N];
// Initialize the finish array to false
for (int i = 0; i < N; i++) {
finish[i] = 0;
}
// Calculate the need matrix
for (int i = 0; i < N; i++) {
for (int j = 0; j < M; j++) {
need[i][j] = max[i][j] - allocation[i][j];
}
}
int work[M];
for (int i = 0; i < M; i++) {
work[i] = available[i];
}
int count = 0;
while (count < N) {
int found = 0;
for (int i = 0; i < N; i++) {
if (finish[i] == 0) {
int j;
for (j = 0; j < M; j++) {
if (need[i][j] > work[j]) {
break;
}
}
if (j == M) {
for (int k = 0; k < M; k++) {
work[k] += allocation[i][k];
}
finish[i] = 1;
processes[count++] = i;
found = 1;
}
}
}
if (found == 0) {
// If no process can be granted resources, it's an unsafe state
return 0;
}
}
// If all processes have been granted resources, it's a safe state
return 1;
}
int main() {
int processes[N];
int available[M] = {3, 3, 2};
int max[N][M] = {{7, 5, 3}, {3, 2, 2}, {9, 0, 2}, {2, 2, 2}, {4, 3, 3}};
int allocation[N][M] = {{0, 1, 0}, {2, 0, 0}, {3, 0, 2}, {2, 1, 1}, {0, 0, 2}};
if (isSafe(processes, available, max, allocation)) {
printf("Safe state detected. Safe sequence: ");
for (int i = 0; i < N; i++) {
printf("P%d ", processes[i]);
}
printf("\n");
} else {
printf("Unsafe state detected. Deadlock may occur.\n");
}
return 0;
}
In this code:
N
represents the number of processes, andM
represents the number of resource types.- The
isSafe
function checks if the system is in a safe state or not by simulating resource allocation and releasing for each process. If a safe sequence is found, it returns 1; otherwise, it returns 0. - The
available
array represents the available instances of each resource type. - The
max
matrix represents the maximum demand of each process for each resource type. - The
allocation
matrix represents the resources currently allocated to each process for each resource type.
This is a simplified example for educational purposes. In a real-world scenario, you would likely have to deal with more dynamic process and resource allocation.