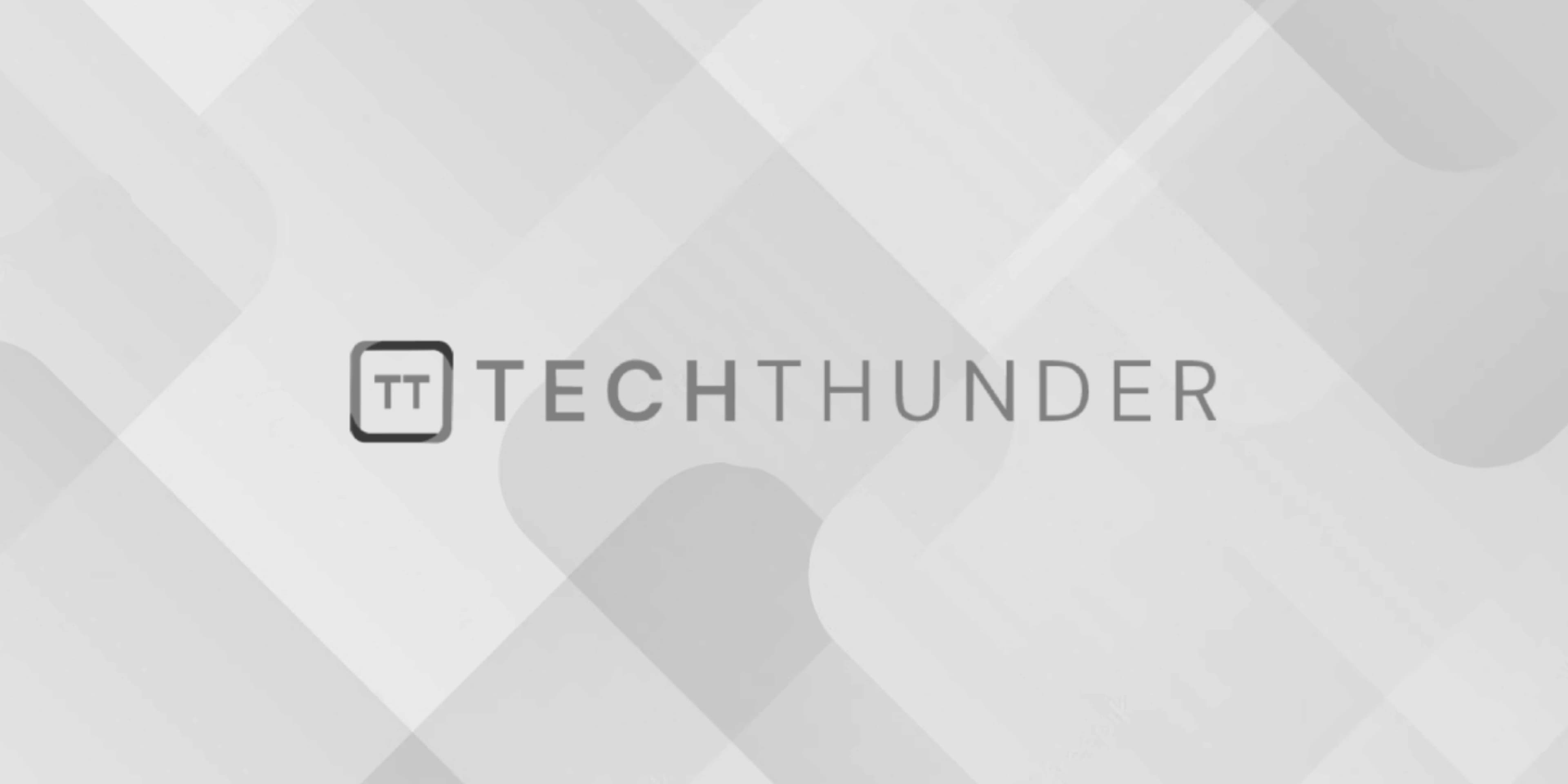
Clearing the Input Buffer in C
Clearing the input buffer in C is important to remove any extra characters (like newline characters) left in the buffer after using input functions like scanf
. It helps ensure that the input buffer is empty before reading new input. You can clear the input buffer in C using various methods:
- Using
getchar()
in a Loop:
int c;
while ((c = getchar()) != '\n' && c != EOF) {}
This code uses getchar()
in a loop to consume characters from the input buffer until a newline character ('\n'
) or the end-of-file (EOF
) is encountered.
- Using
fflush(stdin)
(Non-Standard):
fflush(stdin);
While this method works on some systems, it is not standard C, and its behavior may vary between compilers and platforms. It’s not recommended for portable code.
- Using a Custom Function: You can create a custom function to clear the input buffer:
void clearInputBuffer() {
int c;
while ((c = getchar()) != '\n' && c != EOF) {}
}
Then, call this function when you want to clear the input buffer:
clearInputBuffer();
- Using
fgets
for Input: An alternative approach is to usefgets
for input and then remove the newline character if needed:
char buffer[100];
fgets(buffer, sizeof(buffer), stdin);
// Remove the newline character if present
size_t len = strlen(buffer);
if (len > 0 && buffer[len - 1] == '\n') {
buffer[len - 1] = '\0';
}
This way, you control the input and can easily handle newline characters.
The choice of method depends on your specific needs and coding style. It’s generally a good practice to clear the input buffer when necessary to avoid unexpected behavior when reading input from the user.