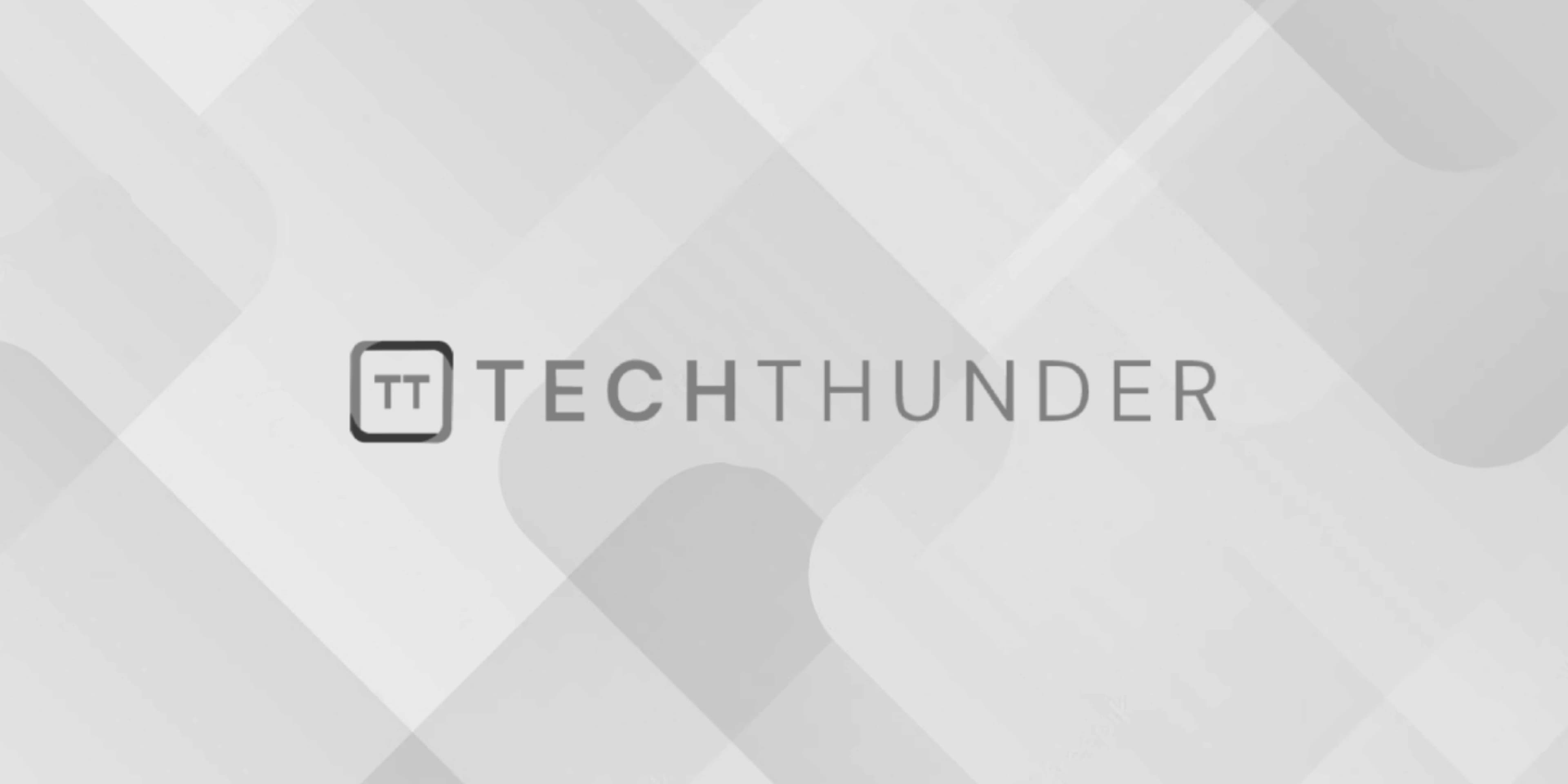
259 views
Null Pointer in C
The null pointer is a pointer that does not point to any valid memory location. It is represented by the constant value NULL
, which is typically defined as (void *)0
. Null pointers are commonly used to indicate that a pointer is not pointing to a valid object or memory location.
Here are some important points to know about null pointers in C:
- Initialization: You can initialize a pointer to be a null pointer by assigning it the value
NULL
or using the macroNULL
. For example:
C
int *ptr1 = NULL;
char *ptr2 = NULL;
- Dereferencing: Attempting to dereference (access the value pointed to by) a null pointer results in undefined behavior. It can lead to program crashes or unexpected behavior. It is essential to check for null pointers before dereferencing them.
- Checking for Null Pointers: Before dereferencing a pointer, you should check if it is a null pointer. You can do this using an
if
statement or a conditional expression. For example:
C
if (ptr != NULL) {
// It's safe to dereference ptr here
}
- Function Return Values: Some C functions return null pointers to indicate errors or failure, such as
malloc()
when it fails to allocate memory. It’s a good practice to check the return value of such functions to handle errors gracefully.
C
int *ptr = malloc(sizeof(int));
if (ptr == NULL) {
// Memory allocation failed, handle the error
}
- Pointer Arithmetic: Performing pointer arithmetic on a null pointer is undefined behavior. It’s essential to ensure that the pointer is valid before performing any pointer arithmetic.
- Assigning Null to Pointers: You can assign
NULL
to a pointer to indicate that it no longer points to a valid object. This can be useful when you want to release or invalidate a pointer.
C
int *ptr = malloc(sizeof(int));
// ...
free(ptr); // Deallocate memory
ptr = NULL; // Set the pointer to NULL to indicate it's no longer valid
- Null Pointers vs. Uninitialized Pointers: A null pointer is distinct from an uninitialized pointer, which is a pointer variable that has not been given a specific value yet. Uninitialized pointers can contain garbage values, and using them without initializing them first leads to undefined behavior.
In summary, null pointers in C are used to represent the absence of a valid memory location. Properly handling null pointers and checking for their presence is crucial for writing robust and safe C code.