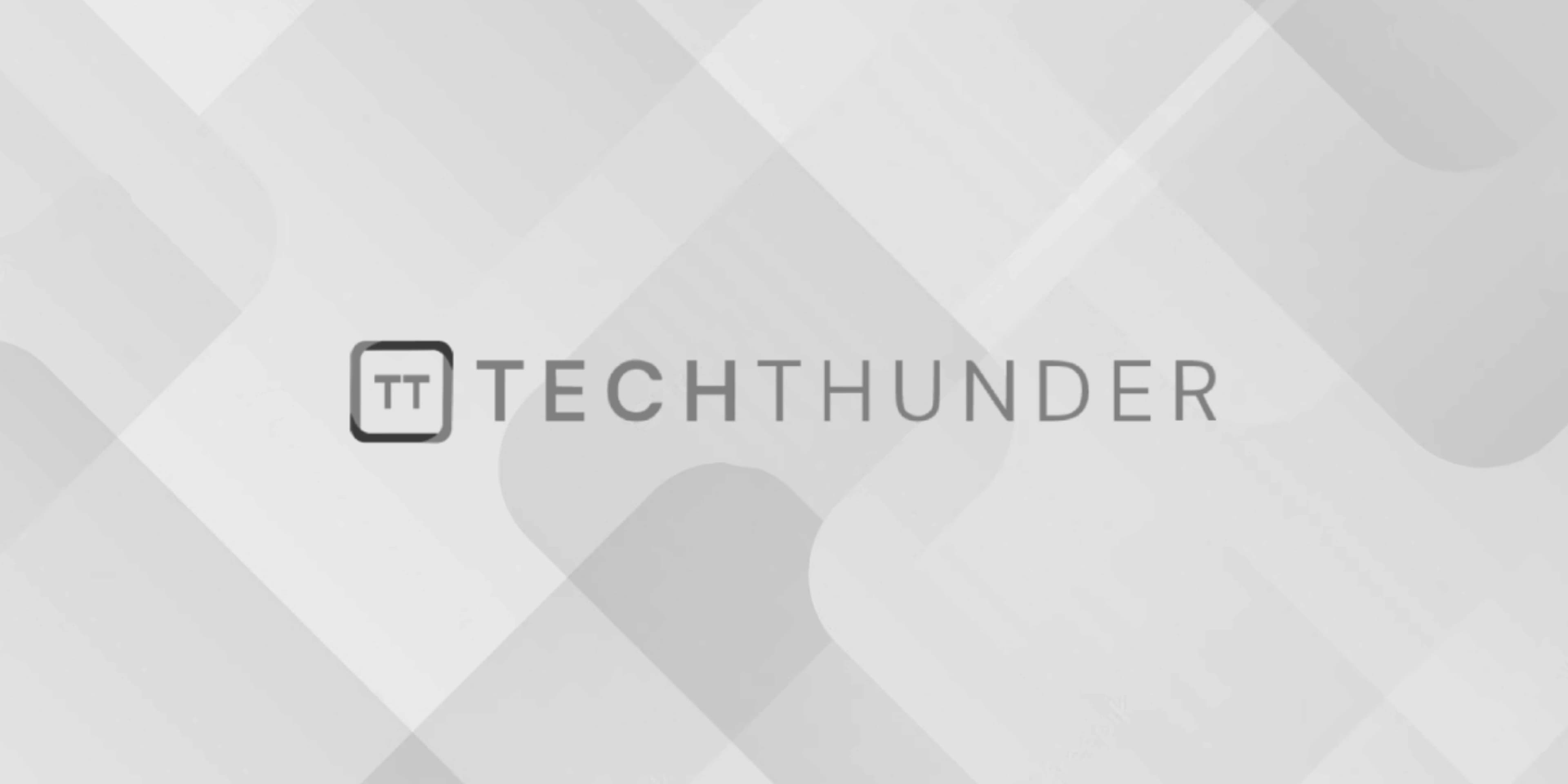
170 views
Alphabet Triangle in C
Creating an alphabet triangle in C involves printing letters of the alphabet in a triangular pattern. Here’s an example of how to create an alphabet triangle in C:
C
#include <stdio.h>
int main() {
int rows, i, j;
char ch = 'A'; // Start with 'A'
printf("Enter the number of rows: ");
scanf("%d", &rows);
for (i = 1; i <= rows; i++) {
// Print spaces before the letters
for (j = 1; j <= rows - i; j++) {
printf(" ");
}
// Print letters in the current row
for (j = 1; j <= i; j++) {
printf("%c ", ch);
ch++;
}
// Move to the next line for the next row
printf("\n");
}
return 0;
}
In this program:
- We prompt the user to enter the number of rows for the alphabet triangle.
- We use nested loops to print the letters in the triangular pattern.
- The outer loop (
i
) iterates through the rows. - The first inner loop (
j
) prints spaces before the letters to align them in a triangular shape. - The second inner loop (
j
) prints the letters in the current row, starting with ‘A’ and incrementing the character in each iteration.
Compile and run the program, and it will generate an alphabet triangle based on the user’s input. The letters of the alphabet will be displayed in a triangular pattern, with each row having one more letter than the previous row.